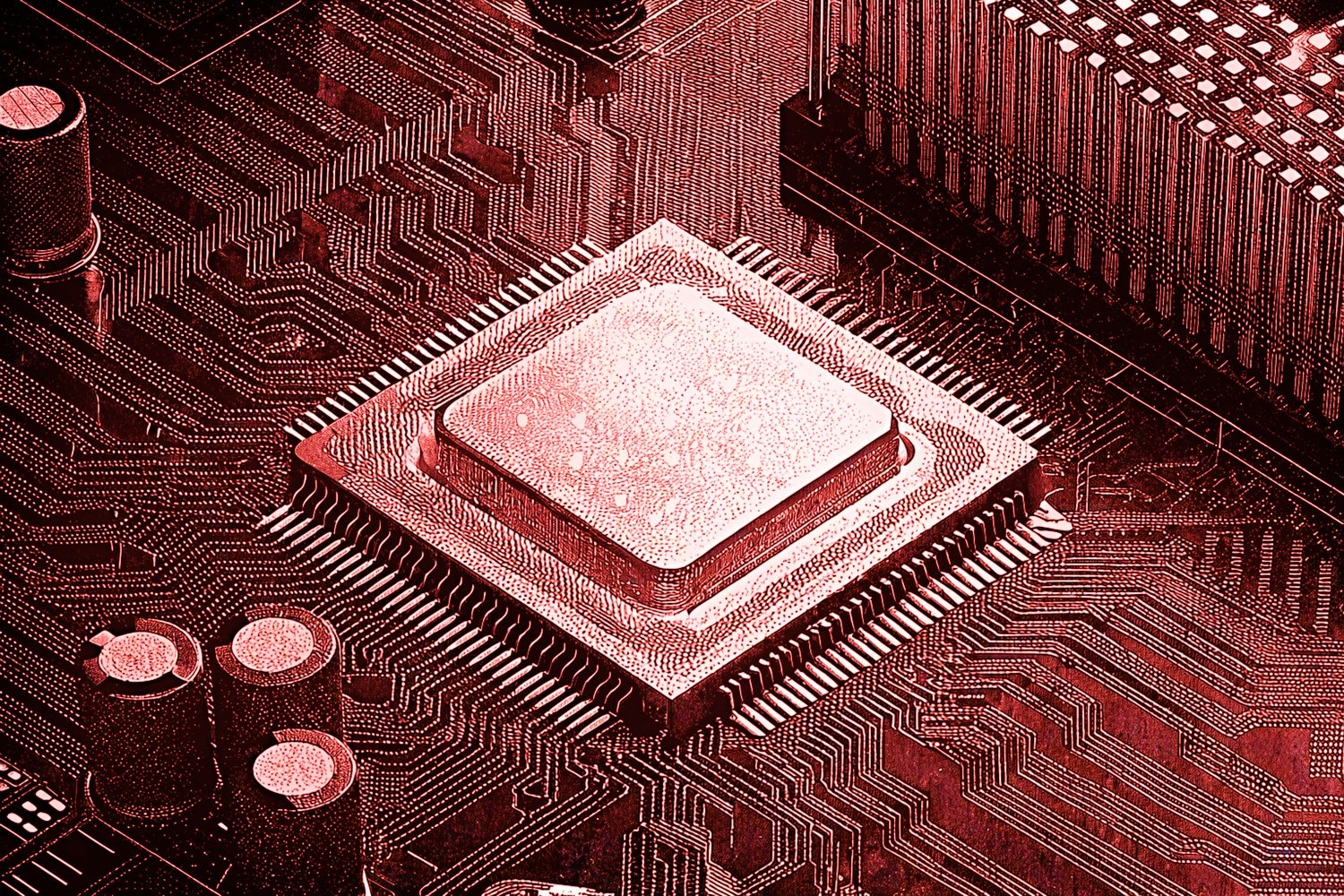
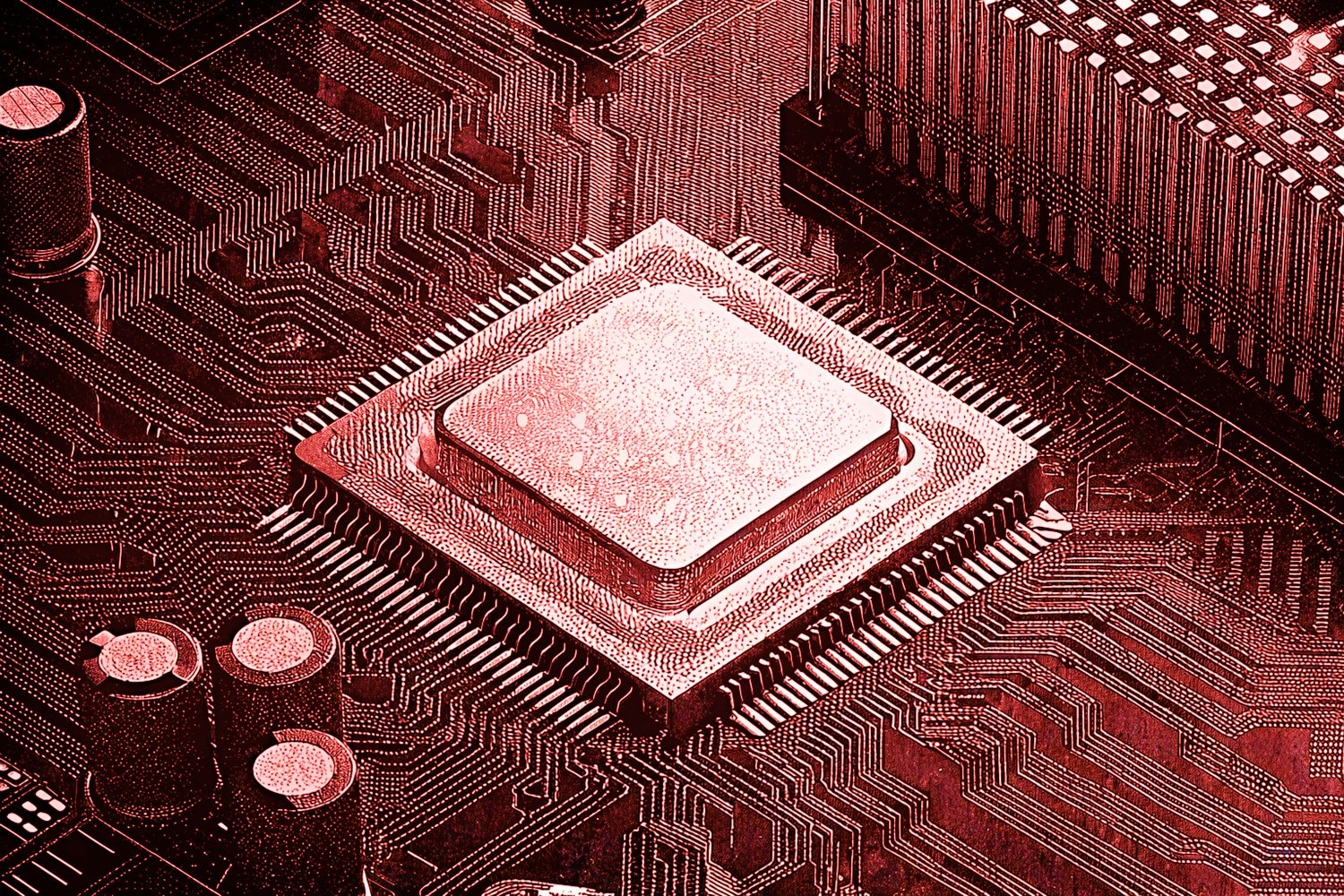
🎓 1/167
This post is a part of the Essentials educational series from my free course. Please keep in mind that the correct sequence of posts is outlined on the course page, while it can be arbitrary in Research.
I'm also happy to announce that I've started working on standalone paid courses, so you could support my work and get cheap educational material. These courses will be of completely different quality, with more theoretical depth and niche focus, and will feature challenging projects, quizzes, exercises, video lectures and supplementary stuff. Stay tuned!
This post was written to structure the fundamental computer science topics essential for data scientists and machine learning specialists.
I also have posts on Linux and web services, and I consider those essentials as well. Linux and web services is a must for most programmers, and even though it's not that close to analysis, you'll still need it sooner or later.
Software development and testing
For machine learning engineers and data scientists, software development principles often blend with the unique requirements of creating, maintaining, and optimizing complex pipelines. Testing, debugging, and profiling are not just technical chores — they're essential tools to ensure that your models perform reliably and efficiently.
Testing Frameworks and Debugging Techniques
The importance of robust testing frameworks in machine learning (ML) cannot be overstated. Your code doesn't just run mathematical models; it interacts with large datasets, external APIs, and often scales across distributed systems. Bugs here can lead to wasted compute hours, silent failures, or worse — misleading conclusions.
Unit Testing, Integration Testing, and System Testing for ML Pipelines
Testing in ML workflows happens at multiple levels:
- Unit Testing:
Unit tests isolate and test individual components of your pipeline. For example, if you're developing a custom data preprocessor, you would write unit tests to verify its handling of edge cases like missing values, NaNs, or unexpected data types.
Frameworks like Pytest simplify this by providing fixtures (reusable test setups) and a concise syntax. Here's a typical Pytest snippet for testing a function that normalizes numerical data:
import pytest
from my_pipeline.preprocessing import normalize
def test_normalize():
input_data = [0, 1, 2]
expected_output = [0.0, 0.5, 1.0]
assert normalize(input_data) == expected_output
-
Integration Testing:
This validates the interaction between multiple components. For instance, does your preprocessed data flow correctly into your feature engineering module? Integration tests often require mock datasets to simulate real-world data. -
System Testing:
System tests evaluate the entire pipeline, including external dependencies like databases, APIs, or distributed frameworks. These tests simulate real-world conditions such as high data throughput or edge-case scenarios.
Tools: Pytest, Unittest, and Coverage Analysis
-
Pytest:
Preferred for its simplicity and extensive plugin ecosystem, Pytest supports everything from parameterized tests to distributed testing. -
Unittest:
Part of Python's standard library, it's more verbose than Pytest but integrates seamlessly into most environments. -
Coverage Analysis:
To ensure you're testing all critical code paths, use tools likecoverage.py
or Pytest'spytest-cov
plugin. These tools generate reports showing which parts of your code were executed during tests. A 100% coverage isn't always necessary but serves as a good metric for identifying weak spots.
Debugging Techniques for ML-Specific Issues
-
Numerical Instability:
Machine learning code often runs into issues like exploding gradients or precision errors. Debugging tools like TensorFlow'stf.debugging
module or manual gradient checks can catch these.Example: If your model loss suddenly returns
NaN
, check for divide-by-zero operations or use gradient clipping to stabilize training: -
Data Pipeline Errors:
Use data validation libraries like TensorFlow Data Validation (TFDV) or custom scripts to check for missing or corrupted records. Logging intermediate pipeline outputs can save hours of debugging. -
Floating-Point Precision:
Issues in numerical computations often arise due to floating-point precision. Use Python'sdecimal
module for critical calculations, or analyze tolerances during equality checks:
from math import isclose
assert isclose(0.1 + 0.2, 0.3, rel_tol=1e-9)
Code Optimization and Profiling
Once your code is functional and tested, the next step is to make it faster and more memory-efficient. Optimization isn't just about speed — inefficiencies in ML workflows often mean higher cloud costs or unmet latency requirements.
Identifying Bottlenecks in ML/DS Workflows
-
Profiling:
Profiling helps pinpoint performance bottlenecks. Tools likecProfile
provide a detailed view of function execution times. For line-by-line analysis,line_profiler
is invaluable.Example workflow:
kernprof -l -v my_script.py
This produces an annotated output, showing the time spent on each line. Focus on hotspots where most of the execution time occurs.
Tools for Profiling and Memory Analysis
-
cProfile:
A built-in Python module that generates reports on function calls, execution time, and call frequency. -
line_profiler:
A more granular tool that shows where your code spends time at the line level. -
memory_profiler:
Monitors memory usage during execution. For instance, you can trace memory consumption in data preprocessing or model training, ensuring that you're not inadvertently loading massive datasets into RAM.
Parallelization and Concurrency Debugging for DS Workflows
Machine learning workflows often involve compute-intensive operations like hyperparameter tuning, training, or distributed inference. Parallelizing these tasks can dramatically reduce execution time.
-
Parallelization Tools:
Use libraries likejoblib
or Python's multiprocessing module to distribute tasks. For example, parallelizing feature engineering across CPU cores can save hours on large datasets. -
Concurrency Debugging:
Debugging concurrent code is notoriously difficult due to race conditions and deadlocks. Tools like thread dumps or Python'sthreading
module can help identify locking issues. -
GPU Profiling:
If you're using GPUs, NVIDIA's Nsight Systems or PyTorch's built-in profiler can measure kernel execution times, memory throughput, and GPU utilization.
Incorporating these techniques and tools into your workflow will not only save you time but also significantly enhance the reliability and performance of your ML systems. Remember, testing and profiling aren't one-time efforts — they evolve alongside your code. Always aim for reproducibility and modularity, and the rest will follow naturally.
Computer architecture
For data scientists and machine learning engineers, understanding computer architecture is critical to building performant systems. The hardware you use can dictate your algorithmic choices and influence the practical viability of your solutions. Let's dive deeper into the nuances of CPU, GPU, memory, and distributed computing to optimize your workflows.
CPU vs. GPU Workloads
Key Differences Between CPU and GPU Architectures
At a high level, CPUs (Central Processing Units) and GPUs (Graphics Processing Units) are designed for different purposes. Understanding these differences can help you decide where to run specific parts of your ML pipeline.
-
CPU Architecture:
CPUs excel at executing a few threads quickly due to their high clock speeds and sophisticated control units. They have fewer cores (typically 4-64 in modern processors) but are optimized for low-latency, sequential tasks like data preprocessing with pandas or decision-tree-based models like XGBoost. -
GPU Architecture:
GPUs are designed to execute thousands of threads simultaneously, making them ideal for highly parallel tasks such as matrix multiplications and deep learning. For example, in TensorFlow or PyTorch, the bulk of training involves operations like:GPUs can handle this efficiently by distributing the work across hundreds or thousands of cores.
Optimizing Workloads
-
CPU Workloads:
Use CPUs for operations that are memory-bound or involve significant branching logic. Examples include:- Feature engineering with pandas/numpy.
- Training ensemble methods (e.g., random forests).
- Preprocessing tasks like parsing JSON or CSV files.
-
GPU Workloads:
Reserve GPUs for computationally intensive tasks like:- Deep neural network training and inference.
- Large-scale matrix computations.
- Batch processing tasks with high throughput.
Best Practices for CPU and GPU Utilization
- Mixed Workloads: Use libraries like cuDF to preprocess data on GPUs while training models simultaneously.
- Pinned Memory: Use pinned memory to improve data transfer speeds between CPU and GPU. For instance:
import torch
data = torch.randn(1000, 1000).pin_memory()
gpu_data = data.cuda(non_blocking=True)
- Batching: Always process data in batches when working with GPUs to minimize idle time and maximize throughput.
Memory Hierarchies and Caching
Memory architecture plays a pivotal role in ML workflows, especially when handling large datasets or training massive models.
Understanding RAM, Cache, and Storage Hierarchies
The memory hierarchy impacts both speed and cost. Here's a breakdown:
- CPU Cache: The fastest memory, but limited in size (measured in MB). Ideal for storing frequently accessed variables during computation.
- RAM (Random Access Memory): Significantly larger but slower than the CPU cache. It stores active processes and data during execution.
- Storage (HDD/SSD): The slowest in the hierarchy but critical for persistent storage. SSDs, being faster than HDDs, are preferred for ML workloads.
How Memory Bandwidth Impacts ML Performance
Memory bandwidth refers to the rate at which data can be transferred between memory and processors. Bandwidth bottlenecks can lead to slower training or inference times, especially for memory-intensive models. For example:
- Loading large datasets into RAM can overwhelm bandwidth, slowing down preprocessing steps.
- GPUs with higher memory bandwidth (e.g., HBM2 in NVIDIA A100) can significantly accelerate tensor computations.
Effective Use of Shared Memory on GPUs
Shared memory on GPUs is a small, fast memory accessible by all threads within a block. Proper use of shared memory can dramatically improve performance in algorithms like convolutions or reductions. Example:
- Without Shared Memory: Threads fetch the same data from global memory multiple times.
- With Shared Memory: Threads collaborate by storing data in shared memory once and reusing it.
// Example: CUDA kernel using shared memory
__shared__ float tile[BLOCK_SIZE][BLOCK_SIZE];
tile[threadIdx.y][threadIdx.x] = global_data[index];
__syncthreads();
Distributed Computing Basics
Scaling ML workflows often requires distributing tasks across multiple cores or machines.
Multi-Core Processors and Hyper-Threading
Modern CPUs leverage multiple cores and hyper-threading to parallelize tasks. For instance:
- Parallel Pandas: Use Dask to process dataframes across CPU cores.
- Numba: A JIT compiler that can parallelize operations over multi-core processors:
from numba import jit, prange
@jit(parallel=True)
def compute_sum(arr):
result = 0
for i in prange(len(arr)):
result += arr[i]
return result
MPI and Distributed Frameworks for ML
Distributed computing frameworks enable scaling across clusters of machines.
-
MPI (Message Passing Interface):
Used for tightly coupled tasks, MPI excels in high-performance computing (HPC) scenarios like parallel matrix factorizations. -
Dask:
Dask simplifies distributed computing for dataframes and arrays. It integrates seamlessly with existing Python libraries like pandas, allowing you to scale preprocessing to clusters with minimal code changes. -
Spark:
Apache Spark is ideal for big data processing. It uses a distributed data abstraction called RDD (Resilient Distributed Dataset) to process large datasets efficiently. -
Horovod:
Horovod simplifies distributed deep learning by scaling TensorFlow, PyTorch, and Keras training across GPUs and nodes. It uses a ring-allreduce algorithm to optimize communication overhead:
Key Considerations for Distributed ML
- Data Sharding: Split data across nodes to reduce memory load.
- Checkpointing: Save intermediate states to handle failures gracefully.
- Cluster Resource Management: Tools like Kubernetes or SLURM can help manage distributed ML jobs efficiently.
Understanding computer architecture is essential for optimizing machine learning workloads. From leveraging GPU acceleration to scaling across distributed clusters, knowing how your code interacts with hardware can unlock significant performance gains. The next time you face a slow pipeline or training process, remember — it's not always the algorithm; sometimes, it's the architecture.
Operating systems and virtualization
Understanding operating systems (OS) and virtualization is vital for optimizing and deploying machine learning (ML) workflows. The OS serves as the bridge between hardware and software, managing resources like CPU, memory, and storage. Virtualization and containerization tools add another layer of abstraction, enabling scalability and reproducibility. Let's explore these concepts and their relevance to data science (DS) and ML.
OS-Level Resource Management
Process Scheduling and Resource Allocation for ML Jobs
Modern operating systems use process schedulers to distribute CPU time among active tasks. For ML workloads, efficient resource allocation can significantly impact performance.
- Schedulers:
Most OS kernels employ preemptive multitasking with schedulers like the Completely Fair Scheduler (CFS) in Linux. ML engineers can optimize job execution by setting process priorities or affinities:- Use
nice
orrenice
commands to adjust process priorities. - Bind tasks to specific CPU cores for better cache utilization using tools like
taskset
:
- Use
taskset -c 0,1 python train_model.py
- Memory Allocation:
Memory management is critical, especially for large models or datasets. Use tools likeulimit
to manage memory limits for processes:
ulimit -m 819200 # Set memory limit to 800MB
Handling I/O Bottlenecks for Data-Intensive Tasks
I/O bottlenecks often arise when tasks wait for data to be read from disk or transferred over the network.
-
Asynchronous I/O:
Use asynchronous libraries like Python'sasyncio
to overlap computation with I/O operations, reducing idle time. -
Batching I/O:
Aggregate small I/O requests into larger ones to minimize latency. For example:
import pandas as pd
chunk_size = 10**6
for chunk in pd.read_csv('large_dataset.csv', chunksize=chunk_size):
process(chunk)
- Disk Prefetching:
Tools like Linux I/O schedulers (e.g.,noop
,deadline
) allow tuning disk read/write behavior. For high-throughput workloads, thedeadline
scheduler often performs better by reducing seek times.
Managing File Descriptors, Thread Pools, and Process Limits
File descriptors represent open files, sockets, or pipes. ML pipelines often hit limits when handling numerous open files, particularly in distributed systems or high-concurrency scenarios.
- Increase File Descriptors:
Adjust system-wide limits for open files in Linux:
echo "fs.file-max = 100000" >> /etc/sysctl.conf
sysctl -p
- Thread and Process Pools:
Libraries like Python'sconcurrent.futures
ormultiprocessing
help efficiently manage threads and processes. Use thread pools for I/O-bound tasks and process pools for CPU-bound tasks.
Containerization and Virtualization
Docker and Kubernetes for Deploying DS/ML Models
Containerization allows ML engineers to package code, dependencies, and configurations into isolated environments.
- Docker:
Docker simplifies the deployment of ML models across different environments. A typical Dockerfile for ML looks like:
FROM python:3.9-slim
RUN pip install numpy pandas scikit-learn
COPY app.py /app/
CMD ["python", "/app/app.py"]
- Kubernetes:
Kubernetes (K8s) orchestrates containers, making it easier to scale ML deployments. Use Kubernetes to manage resources dynamically across nodes and ensure high availability. Key features include:- Horizontal Pod Autoscaling for scaling ML inference workloads.
- Persistent Volumes for shared storage across containers.
Efficient Resource Utilization in Containerized Environments
Containers share the host OS kernel but can isolate resource usage (CPU, memory) through control groups (cgroups). To optimize resource utilization:
- Limit container CPU and memory usage:
docker run --cpus=2 --memory=4g my_ml_container
- Use GPU-enabled containers with NVIDIA Docker:
docker run --gpus all nvcr.io/nvidia/pytorch:latest
Best Practices for Reproducibility in Experiments
- Environment Pinning:
Userequirements.txt
orconda
to ensure consistent package versions inside containers:
pip freeze > requirements.txt
- Snapshot Containers:
Save container states as Docker images to ensure future reproducibility:
docker commit container_id my_ml_snapshot
- Version Control for Configurations:
Store environment variables, model parameters, and pipeline configurations in version-controlled files like YAML or JSON.
File Systems and Disk I/O
Performance Considerations for Local vs. Distributed File Systems
The choice of file system affects how data is stored and retrieved:
-
Local File Systems:
Systems like EXT4 or NTFS are ideal for single-node setups but can become a bottleneck for large-scale distributed ML workflows. -
Distributed File Systems:
Systems like Hadoop Distributed File System (HDFS) or Amazon S3 are designed for scalability and fault tolerance. Use these for handling datasets that exceed local storage capacity.- HDFS: Efficient for batch processing in frameworks like Apache Spark.
- S3: Offers high availability and integrates seamlessly with cloud-based ML workflows.
Managing Large Datasets and Access Patterns
-
Read-Heavy Workloads:
Use techniques like sharding to split data across storage nodes, reducing contention. Libraries likefsspec
in Python can interface with distributed storage. -
Write-Heavy Workloads:
Optimize disk writes by buffering data in memory before committing to disk:
with open("output.csv", "w", buffering=10**7) as f:
f.write(large_string)
- File Compression:
Compress large files to save disk space and reduce I/O overhead. Common formats like Parquet or Avro are optimized for analytical queries.
Monitoring Disk I/O
Use tools like iotop
or iostat
to monitor disk performance during ML training or preprocessing:
- iotop: Shows real-time disk read/write rates.
- iostat: Provides detailed statistics on I/O operations, helping identify bottlenecks.
Operating systems and virtualization provide the foundational tools for managing resources and deploying ML systems at scale. By leveraging these techniques, you can ensure that your pipelines run efficiently and reproducibly, even as workloads grow in complexity.
Computer networks
For machine learning engineers and data scientists, understanding computer networks is crucial when building systems that rely on distributed computation, cloud deployments, or serving models to end-users. This chapter focuses on the networking principles that underpin ML workflows, distributed training, and secure model deployment.
Networking Basics for ML Applications
Networking provides the backbone for connecting components of modern ML systems, whether they are APIs serving predictions or distributed training clusters exchanging data.
HTTP/HTTPS Protocols for APIs and Model Deployment
-
HTTP (Hypertext Transfer Protocol):
HTTP is a stateless protocol used to send and receive data between clients and servers. It's widely used for RESTful APIs in ML deployments.Example: A RESTful endpoint for model inference might look like this:
POST /predict HTTP/1.1
Content-Type: application/json
{
"input": [0.1, 0.5, 0.2]
}
The server responds with predictions:
HTTP/1.1 200 OK
Content-Type: application/json
{
"prediction": "class_A"
}
- HTTPS (HTTP Secure):
HTTPS encrypts data between the client and server using SSL/TLS, ensuring confidentiality and integrity. Always use HTTPS when deploying ML models over the web to protect sensitive data.
Low-Level Networking Concepts: TCP/IP, Sockets, and RESTful APIs
-
TCP/IP (Transmission Control Protocol/Internet Protocol):
The foundational suite of protocols for the internet. TCP ensures reliable, ordered, and error-checked delivery of data, making it ideal for APIs and data transfer in ML pipelines. -
Sockets:
Sockets provide an interface for network communication. Python'ssocket
library allows for low-level TCP/IP communication:
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind(("localhost", 8080))
s.listen(1)
conn, addr = s.accept()
print(f"Connected by {addr}")
- RESTful APIs:
Representational State Transfer (REST) is a popular architectural style for APIs. RESTful services are stateless and use HTTP methods like GET, POST, PUT, and DELETE. Frameworks like Flask and FastAPI make it easy to deploy ML models as RESTful endpoints.
Distributed Training and Model Serving
As datasets and models grow, distributed training becomes essential. Similarly, serving models to users across the globe requires careful consideration of network performance.
Network Considerations in Distributed ML Frameworks
Distributed ML frameworks like TensorFlow, PyTorch, or Horovod rely on network communication to synchronize model parameters and exchange data.
-
Parameter Servers:
Centralized parameter servers manage the model state during training. Workers fetch parameters, compute gradients, and send updates back to the server. This approach is efficient for smaller clusters but can become a bottleneck at scale. -
Collective Communications:
Frameworks like Horovod use collective operations (e.g.,allreduce
) to aggregate gradients across workers. Collective communications are often more efficient in high-bandwidth environments, like clusters with NVIDIA's NVLink.Example:
Latency and Bandwidth Optimization in Cloud-Based Model Serving
When serving models over the network, latency and bandwidth play critical roles in user experience:
-
Reduce Latency:
- Use Content Delivery Networks (CDNs) to cache responses closer to users.
- Optimize API responses by compressing data (e.g., JSON → Protobuf or gRPC).
- Minimize model size using techniques like quantization.
-
Improve Bandwidth Utilization:
- Use batch inference to reduce the frequency of network requests:
inputs = [{"input": x} for x in dataset]
predictions = requests.post("https://api.example.com/batch_predict", json={"inputs": inputs})
- Streamline communication protocols; for example, gRPC uses HTTP/2 for multiplexed, binary data transfer, which is faster than JSON over HTTP/1.1.
Security in Networked ML Systems
ML systems, especially those exposed via APIs or running in distributed setups, are vulnerable to security threats. Protecting these systems is paramount to maintaining data integrity and user trust.
Secure Communication Protocols for APIs
-
TLS/SSL Encryption:
Ensure all communications between the client and server are encrypted using TLS (Transport Layer Security). Tools like Certbot can automate SSL certificate generation for HTTPS. -
API Authentication and Authorization:
- Use tokens (e.g., JWT or OAuth2) to verify clients.
- Implement role-based access control (RBAC) for sensitive operations like retraining models or modifying configurations.
-
Rate Limiting:
Protect APIs from abuse or Denial of Service (DoS) attacks by rate-limiting requests using tools like NGINX or AWS API Gateway.
Preventing Man-in-the-Middle (MITM) Attacks and Eavesdropping
MITM attacks involve intercepting communications between two parties. To mitigate these:
- Enforce HTTPS for all endpoints.
- Use mutual TLS (mTLS) for highly sensitive environments, requiring both client and server to authenticate each other.
- Regularly update software dependencies to patch vulnerabilities.
Additional Security Best Practices
- Input Validation: Sanitize incoming data to prevent injection attacks. For instance:
from werkzeug.security import safe_str_cmp
if not safe_str_cmp(user_input, expected_value):
raise ValueError("Invalid input")
- Logging and Monitoring:
Continuously monitor network traffic for suspicious patterns using tools like Wireshark or cloud-native solutions like AWS CloudWatch and Google Cloud Operations Suite. - Isolation:
Run ML APIs in isolated environments using Docker containers or Kubernetes pods to minimize the impact of potential breaches.
By mastering networking principles, you can design and deploy scalable, efficient, and secure ML systems. Whether optimizing distributed training or ensuring secure model serving, these strategies will help you navigate the complexities of modern computer networks.
Version control (Git)
Git is the cornerstone of modern software development and version control. For data scientists and machine learning engineers, it's an essential tool for tracking changes, collaborating with teammates, and managing project versions — especially in code-driven environments where reproducibility and traceability are key.
Imagine you're working on a machine learning model, tweaking hyperparameters, and modifying feature engineering steps. What happens if a new change breaks your previously successful pipeline? Or, suppose you're collaborating with other engineers, and you need to merge their changes into your work without overwriting their progress. Git provides a robust framework for addressing these challenges.
Basics
Here's a quick overview of how Git works. First, initialize a Git repository in your project directory:
git init
This command initializes an empty repository, creating a .git
folder to track changes.
To track changes, add files to staging:
git add filename.py
Or stage all changes:
git add .
Then commit changes with a descriptive message:
git commit -m "Add initial data preprocessing pipeline"
To see the current state of your repository:
git status
Check what's been modified:
git diff
View commit history:
git log
Branching and merging
Branches let you develop features in isolation. Here's how to use them:
- Create a Branch:
- Make a new branch:
git branch feature-branch
- Switch to it:
git checkout feature-branch
- Merge Changes:
- Combine changes from
feature-branch
intomain
:
- Combine changes from
git checkout main
git merge feature-branch
- Resolve Conflicts:
- If there are conflicts during a merge, Git will prompt you to resolve them manually. Use a code editor or tool like
vim
to edit the conflicting files, then:
- If there are conflicts during a merge, Git will prompt you to resolve them manually. Use a code editor or tool like
git add resolved_file
git commit
Useful commands
- Undo Changes:
- Revert a staged file:
git reset HEAD filename.py
- Undo the last commit but keep changes:
git reset --soft HEAD~1
- Stash Changes: Temporarily save changes without committing:
git stash
Reapply stashed changes:
git stash pop
- Tagging Releases: Mark specific commits with tags:
git tag -a v1.0 -m "Initial model release"
git push origin v1.0
Tips
- Write clear, concise commit messages that explain why changes were made.
- Use
.gitignore
to exclude unnecessary files from version control, such as temporary logs or large datasets. - Regularly push your changes to the remote repository to avoid losing work.
- Take advantage of Git hosting platforms for collaboration — code reviews, pull requests, and issue tracking streamline teamwork.
Cryptography and security
Security is paramount in data science (DS) and machine learning (ML), where sensitive data and valuable models are integral to workflows. Cryptography provides the tools for ensuring confidentiality, integrity, and authenticity, while broader security practices mitigate risks to both data and models. This chapter dives into the principles and applications of cryptography and security in DS/ML systems.
Data Security Fundamentals
Protecting data in ML pipelines ensures confidentiality and compliance with data protection regulations like GDPR and HIPAA. Encryption is a cornerstone of data security, safeguarding information during storage and transfer.
Encryption Techniques for Secure Data Storage and Transfer
Encryption converts plaintext into unreadable ciphertext using cryptographic keys. Decryption reverses this process for authorized parties.
- Symmetric Encryption:
Uses a single key for both encryption and decryption. Examples include AES (Advanced Encryption Standard), which is widely used due to its speed and security.
from Cryptodome.Cipher import AES
from Cryptodome.Random import get_random_bytes
key = get_random_bytes(16) # Generate a secure key
cipher = AES.new(key, AES.MODE_EAX) # Initialize AES cipher
ciphertext, tag = cipher.encrypt_and_digest(b"My secret data")
- Asymmetric Encryption:
Employs a pair of keys (public and private). The public key encrypts data, and the private key decrypts it. RSA is a common algorithm.
from Cryptodome.PublicKey import RSA
from Cryptodome.Cipher import PKCS1_OAEP
key = RSA.generate(2048)
public_key = key.publickey().export_key()
private_key = key.export_key()
cipher = PKCS1_OAEP.new(RSA.import_key(public_key))
encrypted_data = cipher.encrypt(b"My secret data")
- Hashing:
Irreversible algorithms like SHA-256 create fixed-length digests from input data, ensuring integrity but not confidentiality.
Tools for Encrypting Sensitive Data in ML Workflows
Several libraries simplify encryption tasks in Python:
- PyCryptodome: A comprehensive library for symmetric/asymmetric encryption, hashing, and digital signatures.
- Fernet (from Cryptography library): Provides high-level symmetric encryption, ensuring security and ease of use.
from cryptography.fernet import Fernet
key = Fernet.generate_key()
cipher_suite = Fernet(key)
encrypted_data = cipher_suite.encrypt(b"My sensitive ML data")
decrypted_data = cipher_suite.decrypt(encrypted_data)
Model Security
As ML models become central to business operations, they also become targets for attacks. Adversaries might attempt to steal models, manipulate predictions, or infer sensitive training data. Implementing robust model security measures is essential.
Safeguarding Trained Models Against Adversarial Attacks
Adversarial attacks involve crafting inputs designed to deceive ML models. For instance, slight perturbations in an image can mislead a classifier.
- Adversarial Training:
Augment training datasets with adversarial examples to improve model robustness.
from cleverhans.attacks import fast_gradient_method
adv_x = fast_gradient_method(estimator=model, x=original_image, eps=0.1, norm=np.inf)
- Defensive Distillation:
Train a secondary model on the predictions of the original model to reduce its sensitivity to input perturbations.
Techniques for Model Protection
-
Differential Privacy:
Ensures that outputs do not reveal sensitive details about individual training samples. Libraries like PySyft and TensorFlow Privacy implement this technique. -
Model Watermarking:
Embed identifiable features into models to detect theft or unauthorized use. For example, subtle biases in predictions or unique metadata in exported files. -
Robustness Testing:
Continuously evaluate models against simulated attacks. Frameworks like Adversarial Robustness Toolbox (ART) facilitate such testing.
Secure Authentication and Authorization
Ensuring that only authorized users and systems can access data, models, or APIs is critical for secure ML workflows.
OAuth, JWT, and API Key Management in DS/ML Systems
-
OAuth 2.0:
OAuth enables secure access delegation, allowing clients to interact with APIs on behalf of users without exposing credentials. Popular services like Google APIs use OAuth tokens. -
JWT (JSON Web Tokens):
Compact tokens for securely transmitting information between parties. They encode claims (e.g., user ID, access level) and are signed to ensure integrity.
import jwt
secret = "supersecretkey"
payload = {"user_id": 123, "role": "data_scientist"}
token = jwt.encode(payload, secret, algorithm="HS256")
decoded_payload = jwt.decode(token, secret, algorithms=["HS256"])
- API Keys:
API keys are simple tokens used to authenticate clients. They are easy to implement but should be combined with rate-limiting and IP restrictions for enhanced security.
Role-Based Access Control (RBAC) for Datasets and Resources
RBAC restricts access to resources based on predefined roles, ensuring that users only perform actions necessary for their responsibilities.
- Implementing RBAC:
Assign roles (e.g., "viewer", "editor", "admin") and enforce access policies:
{
"role": "editor",
"permissions": ["read_data", "modify_model"]
}
- Tools for RBAC:
Frameworks like Keycloak and AWS IAM provide robust solutions for managing role-based access.
Best Practices for Authentication and Authorization
- Use multi-factor authentication (MFA) to add an extra layer of security.
- Rotate API keys and tokens periodically to minimize the risk of misuse.
- Audit access logs regularly to identify anomalies or unauthorized access attempts.
Secure Shell (SSH) in ML Workflows
Secure Shell (SSH) is a cryptographic network protocol widely used to securely access and manage remote machines. For data scientists and ML engineers, SSH is essential for interacting with cloud servers, transferring data, and maintaining secure communication during distributed training or deployment.
What is SSH?
SSH provides a secure channel over an unsecured network by encrypting data between a client and server. It ensures confidentiality, integrity, and authenticity using:
- Symmetric encryption to encrypt the session.
- Asymmetric key exchange (e.g., RSA or ECDSA) for initial authentication and secure session setup.
- Hashing (e.g., SHA) to verify data integrity.
Key Use Cases in DS/ML
- Remote Server Access:
Data scientists often use SSH to log in to cloud servers hosting Jupyter Notebooks, training scripts, or deployed models.
ssh username@remote_server_ip
- File Transfer with SCP/RSYNC:
SSH facilitates secure file transfers, such as uploading datasets or downloading model artifacts.- SCP:
scp local_file username@remote_server_ip:/path/to/destination
- RSYNC (for syncing directories):
rsync -avz -e ssh local_directory username@remote_server_ip:/path/to/destination
- Tunneling for Port Forwarding:
SSH tunnels allow access to remote services (e.g., Jupyter Notebooks) by securely forwarding ports:
ssh -L 8888:localhost:8888 username@remote_server_ip
Open a browser at localhost:8888
to access the remote Jupyter instance.
- Distributed Training Coordination:
SSH is used for secure communication between nodes in distributed ML frameworks like Horovod or PyTorch DDP.
Key-Based Authentication
SSH keys eliminate the need for passwords and enhance security. A key pair consists of a private key (kept secure) and a public key (shared with the server).
- Generate an SSH Key Pair:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
Save the key and optionally set a passphrase for extra protection.
- Add Public Key to Remote Server:
ssh-copy-id username@remote_server_ip
This adds the key to the ~/.ssh/authorized_keys
file on the server.
- Login Using Key-Based Authentication: After setup, SSH automatically uses the private key for authentication:
ssh username@remote_server_ip
Best Practices for SSH in ML Workflows
-
Use Strong Keys:
RSA keys should have a minimum length of 2048 bits; 4096 is preferred. Alternatively, use modern algorithms like Ed25519 for better performance and security. -
Protect Private Keys:
Store private keys securely (e.g., using a hardware security module or key management tools). Restrict permissions:
chmod 600 ~/.ssh/id_rsa
-
Enable Two-Factor Authentication (2FA):
Combine SSH keys with tools like Google Authenticator or hardware tokens for enhanced security. -
Disable Password Authentication:
Once SSH keys are configured, improve security by disallowing password logins. Edit/etc/ssh/sshd_config
on the server:
PasswordAuthentication no
Restart the SSH service:
sudo systemctl restart ssh
- Use SSH Config for Multiple Connections:
If managing multiple servers, create a~/.ssh/config
file for convenience:
Host myserver
HostName remote_server_ip
User username
IdentityFile ~/.ssh/id_rsa
Port 22
Connect using:
ssh myserver
Advanced Features of SSH
- Agent Forwarding:
Enables seamless authentication across chained SSH sessions without sharing private keys directly:
ssh -A username@intermediate_server
- Multiplexing Connections:
Reuse a single SSH session for multiple connections, improving speed:
Host *
ControlMaster auto
ControlPath ~/.ssh/sockets/%r@%h-%p
ControlPersist 10m
- Secure Git Access:
ML engineers often pull or push code to Git repositories over SSH for better security:
git clone git@github.com:username/repository.git
Advanced topics
This chapter explores cutting-edge topics in machine learning systems engineering, emphasizing the intersection of hardware, algorithms, and deployment paradigms. These areas are critical for optimizing performance, scalability, and efficiency in ML workflows.
Parallel and Distributed Computing for ML
As datasets grow and models become more complex, leveraging parallel and distributed computing is essential. However, understanding their design principles and limitations ensures effective implementation.
Parallel Algorithms in ML and Their Limitations
-
Embarrassingly Parallel Tasks:
Certain tasks, like hyperparameter tuning or independent model training, are trivially parallelizable. Each process works independently, minimizing inter-process communication. -
Data Parallelism:
Splits data across multiple processors while maintaining a single copy of the model. Each processor computes gradients on its subset of the data, followed by aggregation. Common in frameworks like PyTorch and TensorFlow. -
Model Parallelism:
Divides the model itself across processors, often used for large models that don't fit in the memory of a single GPU. Each processor computes a portion of the forward and backward passes.Limitations:
- Communication overhead: Frequent synchronization slows down training.
- Load imbalance: Some tasks may dominate execution time.
- Diminishing returns: Adding more processors doesn't always linearly improve performance due to Amdahl's Law.
Distributed Frameworks
Distributed systems enable training across multiple machines, leveraging both data and model parallelism:
-
MapReduce:
A distributed programming model suited for preprocessing and feature engineering. The "Map" step distributes tasks, while the "Reduce" step aggregates results. Tools like Hadoop and Spark implement this paradigm. -
TensorFlow-Distributed:
TensorFlow'stf.distribute.Strategy
simplifies distributed training. The MirroredStrategy is ideal for synchronous training across multiple GPUs, while MultiWorkerMirroredStrategy extends this across nodes. -
PyTorch Distributed Data Parallel (DDP):
PyTorch DDP is a popular choice for synchronous data parallelism. It minimizes communication overhead by reducing gradients in-place across GPUs.
import torch
from torch.nn.parallel import DistributedDataParallel as DDP
model = DDP(model, device_ids=[0, 1])
Hardware-Aware ML
Optimizing ML workloads for modern hardware accelerators improves speed and energy efficiency, which is especially important in large-scale or resource-constrained environments.
Optimizing ML Workloads for Accelerators
- TPUs (Tensor Processing Units):
Specialized hardware by Google designed for high-throughput matrix operations. TensorFlow provides seamless TPU integration:
resolver = tf.distribute.cluster_resolver.TPUClusterResolver(tpu='your-tpu-address')
tf.config.experimental_connect_to_cluster(resolver)
-
FPGAs (Field Programmable Gate Arrays):
Reconfigurable chips that can be optimized for specific workloads. Tools like Xilinx Vitis AI help deploy ML models on FPGAs, providing a balance between flexibility and performance. -
ASICs (Application-Specific Integrated Circuits):
Fixed-function hardware designed for specific ML tasks. ASICs like Google's TPU chips or inference chips in mobile devices offer unmatched energy efficiency.
Profiling Hardware Utilization and Energy Efficiency
Maximizing hardware utilization requires understanding bottlenecks:
- Use NVIDIA Nsight or nvprof for GPU profiling, identifying inefficiencies like low occupancy or memory-bound operations.
- Analyze energy efficiency using tools like PowerAPI or specialized APIs provided by hardware vendors.
Cloud and Edge Computing in ML
The deployment of ML models often involves trade-offs between cloud-based and edge-based systems, each offering unique benefits and challenges.
Trade-Offs Between Centralized Cloud and Decentralized Edge
-
Cloud Computing:
Centralized infrastructure provides scalable compute and storage. Cloud platforms like AWS, GCP, and Azure offer managed ML services for training and inference.- Advantages: Elastic resources, powerful GPUs/TPUs, easy scaling.
- Disadvantages: Latency for real-time applications, potential data privacy concerns.
-
Edge Computing:
Inference happens locally on edge devices (e.g., mobile phones, IoT sensors), reducing latency and reliance on network connectivity.- Advantages: Low latency, privacy preservation, offline capabilities.
- Disadvantages: Limited compute power, memory constraints.
Deployment Strategies for Edge-Based ML Applications
Deploying models to edge devices involves compression and optimization:
- Model Quantization:
Convert floating-point weights to lower precision (e.g., INT8) to reduce model size and computation requirements.
import tensorflow as tf
converter = tf.lite.TFLiteConverter.from_saved_model('model_dir')
converter.optimizations = [tf.lite.Optimize.DEFAULT]
tflite_model = converter.convert()
-
Pruning:
Remove redundant weights and neurons from a model without significantly impacting accuracy. -
Frameworks for Edge Deployment:
- TensorFlow Lite: Optimized for mobile and embedded devices.
- ONNX Runtime: Supports various hardware backends, making it versatile for edge deployments.
Example for TensorFlow Lite:
import tflite_runtime.interpreter as tflite
interpreter = tflite.Interpreter(model_path="model.tflite")
interpreter.allocate_tensors()