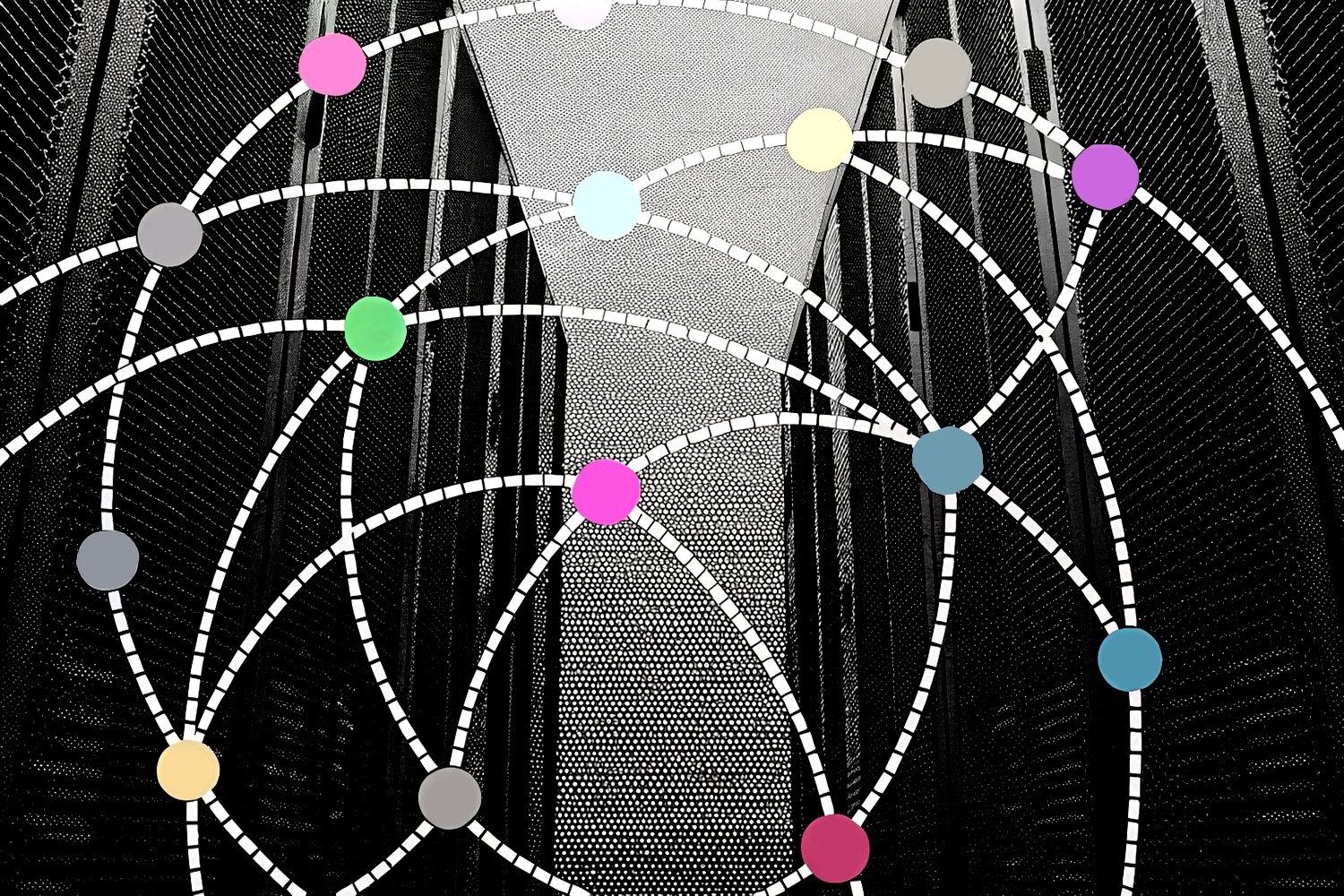
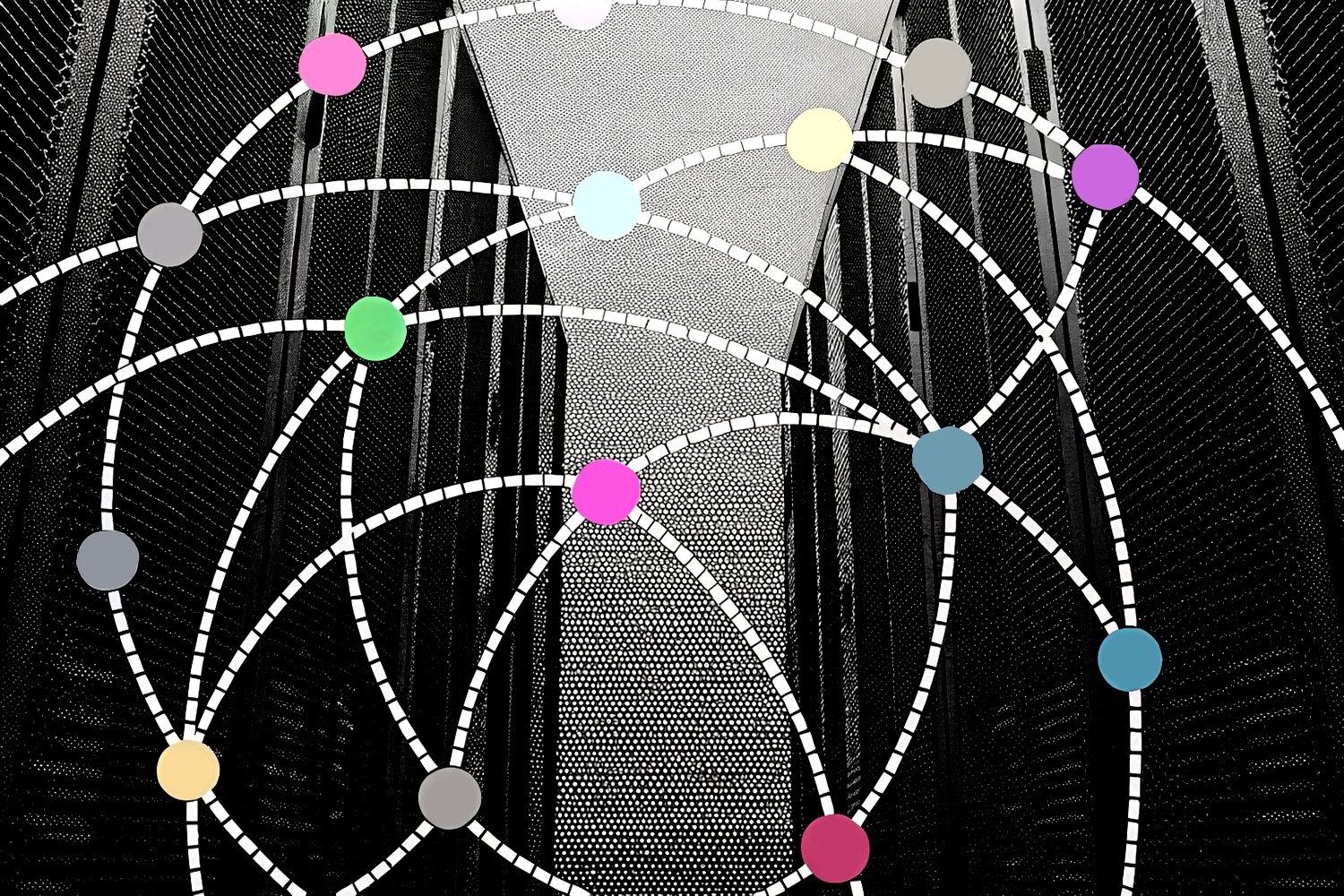
🎓 3/167
This post is a part of the Essentials educational series from my free course. Please keep in mind that the correct sequence of posts is outlined on the course page, while it can be arbitrary in Research.
I'm also happy to announce that I've started working on standalone paid courses, so you could support my work and get cheap educational material. These courses will be of completely different quality, with more theoretical depth and niche focus, and will feature challenging projects, quizzes, exercises, video lectures and supplementary stuff. Stay tuned!
...
Collaboration
Remote repository, git push, git pull and other remote commands (without explaining basic git commands for local machine). Git hosting platforms: GitHub/GitLab. Repository management. Key features: branching, pull requests, issues, CI/CD pipelines. CI/CD Tools: GitHub Actions, GitLab CI for automating deployment pipelines. Collaboration workflows: forking and cloning, branching strategies (e.g., GitFlow), code review and merge workflows.
In the realm of data science and machine learning, where projects often involve multifaceted teams and extensive codebases, effective collaboration tools are non-negotiable. Among these, remote repositories and version control platforms reign supreme, enabling seamless coordination, robust code management, and reproducible workflows. Let's dive into how tools and practices come together to create a symphony of collaboration.
Remote Repositories and Git Commands
At the core of modern collaboration lies the remote repository, a centralized hub where the team's codebase resides. Unlike local repositories, which exist on individual machines, remote repositories are accessible over a network, allowing contributors from anywhere to collaborate in real time. Common commands for interacting with remote repositories include:
git push
: This uploads local commits to the remote repository, effectively syncing changes with the team.git pull
: This retrieves updates from the remote repository, merging them into the local branch.git fetch
: Unlikegit pull
, this command fetches updates from the remote repository without merging, allowing developers to review changes before integrating them.git clone
: This creates a local copy of an entire remote repository, including its history and branches.
Git Hosting Platforms: GitHub and GitLab
Git hosting platforms like GitHub and GitLab provide the infrastructure for managing remote repositories. While both platforms offer overlapping features, their nuances often determine the best choice for a project.
- GitHub: Renowned for its vibrant open-source community, GitHub is the de facto platform for sharing and collaborating on code. Features like GitHub Actions for CI/CD and extensive third-party integrations make it a powerhouse for both individual and team projects.
- GitLab: While also popular for open-source, GitLab excels in DevOps with an all-in-one approach. Its built-in CI/CD tools, robust permission models, and self-hosting options cater to enterprise needs and privacy-conscious teams.
Repository Management: Features That Matter
Managing a remote repository is more than just hosting code — it's about enabling efficient workflows and minimizing friction. Key features include:
- Branching: Allows the team to work on different features or bug fixes simultaneously. strategies are common, where each branch represents a logical unit of work.
- Pull Requests (PRs): Serve as formal proposals to merge changes from one branch into another. They facilitate code review, discussions, and ensure quality control.
- Issues: A lightweight task management system to track bugs, feature requests, and technical debt. Developers often link issues directly to commits or PRs for traceability.
- Continuous Integration/Continuous Deployment (CI/CD): Automates the testing, building, and deployment of code. This ensures that every change is validated and deployable.
CI/CD Tools: GitHub Actions and GitLab CI
Automating repetitive tasks like running tests, building models, or deploying apps is critical in fast-paced projects. GitHub Actions and GitLab CI are powerful CI/CD tools built into their respective platforms:
- GitHub Actions:
- Uses YAML files to define workflows, enabling tasks to run in response to triggers like pushes, PRs, or scheduled events.
- Supports community-contributed actions for tasks ranging from testing to deploying models on cloud platforms.
- Example snippet for running Python tests:
name: CI Pipeline on: [push, pull_request] jobs: test: runs-on: ubuntu-latest steps: - uses: actions/checkout@v3 - name: Set up Python uses: actions/setup-python@v4 with: python-version: '3.8' - run: pip install -r requirements.txt - run: pytest
- GitLab CI:
- Also YAML-based, but deeply integrated with the GitLab ecosystem.
- Supports multiple stages (build, test, deploy) and extensive configurations for pipelines.
- Example snippet for building a Docker image:
stages: - build build-job: stage: build script: - docker build -t my-image:latest . tags: - docker
Collaboration Workflows
Efficient workflows are the backbone of collaborative projects. Whether it's a small team or a sprawling enterprise, the right strategies ensure smooth sailing. Here's how:
-
Forking and Cloning:
- Forking: Creates a copy of a repository under your own account. Ideal for open-source contributions, it keeps the original repository untouched until changes are proposed via a PR.
- Cloning: Downloads the repository to your local system, enabling offline work. Typically used when you have write access to the remote repo.
-
Branching Strategies:
- GitFlow: A structured workflow with distinct branches for development, features, releases, and hotfixes.
- Feature Branching: Each feature or bug fix resides in its own branch, keeping the main branch stable.
- Trunk-Based Development: Prioritizes short-lived branches that are merged frequently into the main branch, fostering rapid iteration.
-
Code Review and Merge Workflows:
- Code Review: Pull requests serve as a focal point for peer reviews. Teams use comments, inline suggestions, and approvals to maintain code quality.
- Merge Strategies:
- Squash and Merge: Combines all commits from a branch into a single commit.
- Rebase and Merge: Integrates changes without creating merge commits, keeping history linear.
- Merge Commit: Default approach, preserving the history of all commits.
Real-World Applications
Imagine collaborating on a machine learning project where the model is deployed in production. The team might:
- Use GitHub Actions to test every commit, ensuring that data preprocessing pipelines and model training scripts work flawlessly.
- Follow a GitFlow strategy, with branches for hyperparameter tuning and exploratory analysis.
- Conduct code reviews via pull requests to maintain reproducibility and adhere to standards like PEP 8.
- Automate CI/CD pipelines to deploy the final model as an API using GitLab CI.
These tools and workflows not only streamline development but also foster a culture of accountability and shared ownership. Every contributor can confidently iterate on the project, knowing the tools are robust enough to catch mistakes and enforce best practices.
Cloud platforms
AWS (Amazon Web Services). Essential services for data science and ML:
- S3: Data storage.
- EC2: Virtual machines for compute.
- Lambda: Serverless computing.
- SageMaker: Managed ML service. Cost management tips and best practices. Other Cloud Platforms: Google Cloud Platform (GCP) and Microsoft Azure — brief comparisons.
Cloud Platforms
In the world of data science and machine learning (ML), cloud platforms are indispensable tools that offer scalable, cost-effective, and accessible resources. Among these, Amazon Web Services (AWS) is a leading provider with a suite of services tailored for data professionals. Let's dive into the essential AWS services for data science and ML, and explore how they can streamline workflows.
AWS: Essential Services for Data Science and ML
S3: Data Storage
Amazon Simple Storage Service (S3) is a highly scalable object storage service. For data scientists, S3 is a backbone for handling large datasets. Here are its key features:
- Scalability: S3 can handle petabytes of data, making it ideal for storing raw data, processed datasets, and model artifacts.
- Accessibility: With APIs, S3 integrates seamlessly with AWS services and external tools, making it easy to retrieve and manipulate data.
- Data Lifecycle Management: You can define rules to transition data between storage classes (e.g., from Standard to Glacier for long-term storage) to optimize costs.
For example, let's say you're training a neural network and have terabytes of images. You can upload the dataset to S3 and access it directly from a training script running on an EC2 instance. Using AWS SDKs like Boto3 in Python simplifies tasks such as listing files, downloading, or uploading data:
import boto3
s3 = boto3.client('s3')
bucket_name = 'my-data-bucket'
file_key = 'dataset/train_images.zip'
# Download file from S3
s3.download_file(bucket_name, file_key, 'train_images.zip')
EC2: Virtual Machines for Compute
Amazon Elastic Compute Cloud (EC2) provides virtual machines (VMs) that can be customized to meet your compute needs. These instances are perfect for running experiments, training ML models, and performing large-scale data analysis. Key considerations include:
- Instance Types: AWS offers a variety of EC2 instance families. For data science, GPU instances (e.g.,
g4dn
,p3
) are commonly used for deep learning tasks, while CPU-focused instances (e.g.,m5
,c5
) are better suited for preprocessing and traditional ML algorithms. - Elasticity: You can scale up or down based on demand, ensuring cost-efficiency.
Here's an example workflow:
- Spin up an EC2 instance with the required compute resources.
- Attach an IAM role for secure access to S3 and other AWS services.
- Run your training or analysis scripts.
- Shut down the instance to avoid incurring unnecessary costs.
Using EC2 Spot Instances — which allow you to bid on unused capacity — can reduce costs significantly, although they're best suited for fault-tolerant tasks.
Lambda: Serverless Computing
AWS Lambda is a serverless computing service that lets you run code without managing servers. For data scientists, Lambda is excellent for lightweight tasks such as:
- Preprocessing: Automate ETL (Extract, Transform, Load) operations.
- Inference: Deploy lightweight ML models for real-time predictions.
For instance, you could write a Lambda function that triggers when a new file is uploaded to an S3 bucket, preprocesses the file, and stores the cleaned data in another location. Below is an example using Python:
def lambda_handler(event, context):
import boto3
s3 = boto3.client('s3')
source_bucket = event['Records'][0]['s3']['bucket']['name']
file_key = event['Records'][0]['s3']['object']['key']
# Perform some preprocessing...
processed_data = preprocess_data(file_key)
# Save processed data
destination_bucket = 'processed-data-bucket'
s3.put_object(Bucket=destination_bucket, Key=file_key, Body=processed_data)
Lambda charges only for the compute time used, making it highly cost-effective for intermittent tasks.
SageMaker: Managed ML Service
Amazon SageMaker is an all-in-one platform for developing, training, and deploying ML models. It removes the complexity of managing infrastructure and provides a variety of tools tailored to data scientists:
- Notebook Instances: Fully managed Jupyter notebooks integrated with AWS services.
- Built-in Algorithms: Pre-optimized ML algorithms for common tasks such as classification, regression, and clustering.
- Training Jobs: Train models at scale using distributed computing.
- Model Deployment: Deploy models with a few clicks, scaling seamlessly with endpoint traffic.
For example, training a model in SageMaker involves three main steps:
- Prepare your dataset in S3.
- Define a training job with the dataset and algorithm.
- Launch the training job, and monitor progress via the SageMaker dashboard.
SageMaker also supports custom algorithms and frameworks like TensorFlow, PyTorch, and Scikit-learn.
Cost Management Tips and Best Practices
While AWS provides immense power, costs can spiral out of control without careful planning. Here are some tips to optimize spending:
- Set Budgets: Use AWS Budgets to monitor spending and receive alerts for threshold breaches.
- Optimize S3 Costs: Transition infrequently accessed data to cheaper storage classes, like S3 Glacier.
- Leverage Spot Instances: For non-critical workloads, use EC2 Spot Instances for up to 90% cost savings.
- Turn Off Idle Resources: Regularly audit running EC2 instances, SageMaker endpoints, and unused EBS volumes.
- Right-Sizing: Choose instance types and sizes that match your workload requirements.
Other Cloud Platforms
While AWS is a dominant player, Google Cloud Platform (GCP) and Microsoft Azure also offer robust tools for data scientists:
Google Cloud Platform (GCP)
- BigQuery: A serverless, highly scalable data warehouse ideal for analytics and querying massive datasets using SQL.
- AI Platform: Managed services for training and deploying ML models, similar to SageMaker.
- TensorFlow Integration: GCP is tightly integrated with TensorFlow, offering optimized environments for training and deploying deep learning models.
Microsoft Azure
- Azure Machine Learning: A comprehensive ML platform with features for experiment tracking, automated ML, and deployment.
- Azure Blob Storage: Scalable object storage, similar to S3.
- Synapse Analytics: A powerful analytics service for big data processing.
Both GCP and Azure have unique strengths, and the choice often depends on existing workflows, preferred tools, and cost considerations. However, AWS's mature ecosystem and wide-ranging services make it a go-to platform for many data scientists.
Containerization and virtualization
Docker. Role in creating reproducible environments. Basics of Dockerfiles and Docker Compose. Use cases: deploying ML models, managing dependencies.
Containerization and Virtualization
In the fast-evolving fields of data science and machine learning, ensuring consistency across development, testing, and production environments can be a daunting task. Containerization and virtualization are powerful techniques that address this challenge by isolating applications and their dependencies from the underlying system.
Docker: A Data Scientist's Best Friend
At the heart of containerization is Docker, a platform that packages applications and their dependencies into standardized units called containers. These containers are lightweight and portable, making them ideal for reproducibility and scaling in data science workflows.
Imagine you've developed a machine learning model that works perfectly on your laptop but throws errors when deployed to a server. This common frustration often stems from mismatched library versions, dependency conflicts, or missing system configurations. Docker eliminates these problems by encapsulating everything your application needs — from Python libraries to operating system dependencies — in a single container.
Key Concepts in Docker
Let's break down some essential Docker components:
-
Docker Images: These are immutable snapshots of your application and its environment. Think of them as templates for creating containers. For example, you might use an image based on
python:3.9
to ensure your code always runs on Python 3.9. -
Docker Containers: Containers are the running instances of Docker images. While images are static, containers are dynamic and can be started, stopped, and modified as needed.
-
Docker Hub: This is a repository for sharing and downloading Docker images. It's like GitHub, but for container images. Many pre-built images (e.g., TensorFlow, PyTorch) are available here to accelerate your workflow.
Writing a Dockerfile
A Dockerfile
is a text file containing a set of instructions to build a Docker image. Here's an example Dockerfile
for a machine learning project:
# Use an official Python runtime as a base image
FROM python:3.9
# Set the working directory
WORKDIR /app
# Copy the requirements file into the container
COPY requirements.txt ./
# Install dependencies
RUN pip install --no-cache-dir -r requirements.txt
# Copy the rest of the application code
COPY . .
# Specify the command to run the application
CMD [ "python", "main.py" ]
Let's dissect this step by step:
- Base Image:
FROM python:3.9
ensures the container starts with Python 3.9 pre-installed. - Working Directory:
WORKDIR /app
sets/app
as the working directory inside the container. - Dependencies: The
COPY requirements.txt ./
andRUN pip install
steps install Python dependencies listed inrequirements.txt
. - Application Code:
COPY . .
copies all your project files into the container. - Default Command:
CMD [ "python", "main.py" ]
specifies the default command to run when the container starts.
Docker Compose: Orchestrating Multiple Containers
In real-world projects, your machine learning pipeline might involve multiple services, such as a database, a message queue, and an API server. Docker Compose simplifies managing these interconnected services by allowing you to define them in a single docker-compose.yml
file.
Here's an example docker-compose.yml
for deploying an ML model:
version: '3.8'
services:
app:
build: .
ports:
- "5000:5000"
volumes:
- .:/app
redis:
image: "redis:latest"
In this configuration:
- The
app
service builds the image from yourDockerfile
and maps port 5000 on your host to port 5000 in the container. - The
redis
service pulls the latest Redis image from Docker Hub to handle caching or queuing tasks.
Use Cases: Deploying ML Models and Managing Dependencies
Deploying ML Models
One of the most common use cases for Docker in data science is deploying machine learning models as REST APIs. By containerizing the model and its serving logic (e.g., Flask, FastAPI), you ensure it runs consistently across environments. For example:
- Package the trained model (
.pkl
or.h5
file) into a Docker image. - Serve predictions via an API endpoint exposed by the container.
Managing Dependencies
Data science projects often rely on a tangled web of dependencies. Docker ensures you're working with the exact versions of libraries and tools your project requires. This is particularly useful when collaborating across teams or sharing code, as teammates can spin up identical environments using your Docker image.
For instance, if your project uses TensorFlow 2.11 and a specific CUDA version, your Dockerfile
can lock these dependencies to prevent compatibility issues.
REST APIs for model deployment
Introduction to REST APIs. Role in integrating ML models into web applications. Overview of REST principles: endpoints, HTTP methods (GET, POST, etc.), and JSON payloads. Flask: brief overview, just to get familiar with the technology. Basics of setting up a web server. Creating endpoints for ML model predictions. Running and testing a local Flask app. Mark Flask as an optional technology, but very useful for data scientists.
REST APIs for Model Deployment
When deploying machine learning models, making them accessible to users, applications, or other services is crucial. A common way to achieve this is through REST APIs. Let's break down what REST APIs are, why they matter for ML model integration, and how you can set up a simple API using Flask to serve your predictions.
What is a REST API?
A REST API (Representational State Transfer Application Programming Interface) is a standardized way for different software systems to communicate over the web. It allows clients (like web browsers, mobile apps, or other services) to request data or perform actions on a server, which can host anything from databases to ML models.
Key characteristics of REST APIs include:
- Statelessness: Each request from a client to the server must contain all the information needed to process the request. The server does not retain client state between requests.
- Resource-based: REST APIs revolve around the concept of resources, which are data entities like user profiles, datasets, or predictions from a model.
- Uniform Interface: Clients interact with resources via a standardized set of HTTP methods:
- GET: Retrieve data (e.g., fetch predictions or model details).
- POST: Submit data to be processed (e.g., send input for model inference).
- PUT/PATCH: Update existing data.
- DELETE: Remove data.
A typical REST API exchange involves:
- Endpoints: URLs representing resources (e.g.,
/predict
). - Payloads: Data sent between the client and server, often in JSON format.
- Responses: The server's reply, typically containing status codes (e.g.,
200 OK
or400 Bad Request
) and any requested data.
Why REST APIs for Machine Learning Models?
When a machine learning model is trained and ready for production, deploying it through a REST API enables:
- Interoperability: Any client or application that can make HTTP requests can use the model, regardless of programming language or platform.
- Scalability: REST APIs are designed to support high traffic, making them suitable for large-scale applications.
- Seamless Integration: Many modern web and mobile apps are built to consume REST APIs, making it a natural fit for serving ML predictions.
Imagine a use case where you have a trained model for image classification. A REST API could allow users to upload an image via a POST request and receive the predicted label in the response. This makes the model accessible beyond your local environment, bridging the gap between development and practical usage.
REST Principles: A Quick Overview
-
Endpoints: Each endpoint corresponds to a specific resource or action.
- Example:
/predict
for sending input data to your model.
- Example:
-
HTTP Methods:
- GET: Retrieve resources (e.g., get the model's metadata).
- POST: Submit input data to the model (e.g., make predictions).
- PUT/PATCH: Update configurations or hyperparameters (optional in some cases).
- DELETE: Remove or reset certain model states (rare in typical deployments).
-
JSON Payloads: REST APIs typically exchange data in JSON (JavaScript Object Notation) format due to its lightweight structure and human readability.
- Example payload for a POST request to predict house prices:
{ "features": [1200, 3, 2, 0.5] }
- Example payload for a POST request to predict house prices:
Setting Up a REST API with Flask
While various frameworks can be used to build REST APIs, Flask is an excellent option for data scientists due to its simplicity and flexibility. Flask is a lightweight Python web framework that makes it easy to set up a local web server and define API endpoints.
Step 1: Install Flask
Ensure you have Flask installed in your Python environment:
pip install flask
Step 2: Create a Simple Flask App
Here's an example of a minimal Flask app to expose a machine learning model for predictions:
from flask import Flask, request, jsonify
import pickle # Replace with your model-loading logic
app = Flask(__name__)
# Load the trained ML model
with open('model.pkl', 'rb') as f:
model = pickle.load(f)
@app.route('/predict', methods=['POST'])
def predict():
# Parse input JSON payload
data = request.json
features = data['features']
# Make prediction
prediction = model.predict([features])
# Return prediction as JSON
return jsonify({'prediction': prediction[0]})
if __name__ == '__main__':
app.run(debug=True)
Explanation of the Code
-
Flask
app initialization:- The
Flask
object,app
, is the core of your web server. It handles incoming requests and routes them to appropriate functions.
- The
-
Loading the model:
- This example uses
pickle
to load a previously saved model. You can replace this with libraries likejoblib
or any custom serialization logic.
- This example uses
-
Defining the
/predict
endpoint:- The
@app.route
decorator defines a URL endpoint (/predict
) that accepts POST requests. - The
request.json
object extracts the JSON payload from the client.
- The
-
Returning JSON responses:
- Use
jsonify()
to send structured responses, ensuring compatibility with REST standards.
- Use
-
Running the app:
- The
app.run(debug=True)
command starts the server. Usedebug=True
during development for real-time error tracking.
- The
Testing the Flask App
-
Run the App Locally: Save the script as
app.py
and run:python app.py
The app will start a server at
http://127.0.0.1:5000
. -
Use a Tool for Testing:
- cURL: A command-line tool for making HTTP requests.
curl -X POST http://127.0.0.1:5000/predict \ -H "Content-Type: application/json" \ -d '{"features": [1200, 3, 2, 0.5]}'
- Postman: A GUI tool for crafting and sending API requests.
- cURL: A command-line tool for making HTTP requests.
-
Inspect the Response: The server should return a JSON object with the model's prediction:
{ "prediction": 350000 }
Flask: An Optional Yet Valuable Tool
While Flask is highly accessible and an excellent choice for small-scale deployments or prototyping, it's not the only option. Frameworks like FastAPI offer better performance and built-in validation, and larger-scale systems might use Django or a cloud-native service like AWS API Gateway. However, Flask remains a practical, approachable choice for data scientists venturing into web app development.
Things you don't have to learn, but should get familiar with
1. Basics of orchestration
Kubernetes fundamentals for data scientists, just to get familiar with the technology. This tools is mostly considered DevOps tools, but knowing how to communicate with DevOps is kinda important for data scientists. Managing containerized applications at scale. Overview of key concepts: pods, deployments, services. Use Cases in ML: deploying models using Kubernetes, integrating with cloud services like AWS EKS or GCP GKE.
2. Basics of monitoring and logging
Fundamentals of monitoring and logging. Importance for production systems. Tools like Prometheus, Grafana, and ELK Stack. This tools is mostly considered DevOps tools, but knowing how to communicate with DevOps is kinda important for data scientists.
3. Basics of data pipelines
Tools like Apache Airflow for workflow orchestration. Differences of data pipelines and other kinds of pipelines (CI/CD pipelines). This tools is mostly considered data engineering tools, not data science tools, but it's worth to get familliar.
Things You Don't Have to Learn, But Should Get Familiar With
1. Basics of Orchestration
As a data scientist, you're likely immersed in datasets, modeling, and analysis, but there's a parallel world of DevOps and orchestration that, while not core to your role, can significantly enhance your effectiveness. Enter Kubernetes — a powerful tool for managing containerized applications at scale. While it's often associated with DevOps, having a basic understanding of Kubernetes can smoothen your collaboration with DevOps teams and help you deploy and manage ML models more effectively.
Kubernetes Fundamentals
At its core, Kubernetes (often abbreviated as K8s) is an open-source platform designed to automate deploying, scaling, and operating application containers. Containers are lightweight, standalone, executable packages of software that include everything needed to run an application: code, runtime, libraries, and settings.
Key concepts to understand in Kubernetes:
- Pods: The smallest deployable unit in Kubernetes. A pod usually contains one container, but it can host multiple containers that share the same network namespace and storage. Think of pods as wrappers around your application.
- Deployments: Higher-level abstractions that manage pods. Deployments ensure that the specified number of pod replicas are running at any given time. If a pod crashes, the deployment will replace it.
- Services: Persistent abstractions that expose your pods to external systems or other internal services. They act as load balancers and maintain connectivity even if pod IPs change.
Why Kubernetes Matters for ML
While Kubernetes might feel like DevOps territory, it's invaluable for:
- Deploying Models: Kubernetes allows you to deploy ML models as containerized applications, scaling them up or down based on demand. For instance, you could deploy a trained model as a REST API using tools like Flask or FastAPI, containerize it using Docker, and manage it with Kubernetes.
- Cloud Integration: Popular cloud services like AWS Elastic Kubernetes Service (EKS) and Google Kubernetes Engine (GKE) integrate seamlessly with Kubernetes, providing additional scalability and resilience.
Even if you're not managing Kubernetes clusters yourself, knowing its basics can help you:
- Communicate with DevOps about deploying your models.
- Debug deployment issues effectively.
- Understand how infrastructure decisions impact your applications.
2. Basics of Monitoring and Logging
Production ML systems are rarely "set it and forget it" — they require ongoing monitoring and logging to ensure performance, reliability, and accuracy. While setting up monitoring and logging may not fall directly under your responsibilities, understanding these fundamentals can be invaluable.
Why Monitoring and Logging Matter
-
Monitoring helps you observe system performance in real-time. Key metrics for ML systems might include:
- Model inference latency.
- API response times.
- Resource usage (CPU, GPU, memory).
-
Logging involves capturing a record of events within the system. Logs are critical for troubleshooting errors, identifying anomalies, and auditing.
Key Tools for Monitoring and Logging
-
Prometheus:
- A monitoring and alerting toolkit designed for time-series data. It uses a flexible query language (PromQL) to create dashboards and alerts.
- In ML, Prometheus can monitor metrics like model inference time or system load.
-
Grafana:
- A visualization tool that integrates seamlessly with Prometheus. It provides interactive dashboards to monitor your system's health at a glance.
-
ELK Stack:
- A combination of three tools: Elasticsearch (search engine), Logstash (data processing pipeline), and Kibana (visualization).
- The ELK Stack is ideal for aggregating, searching, and analyzing logs from multiple sources.
How This Relates to ML
Imagine you've deployed a model predicting customer churn. Monitoring tools can alert you if the model's response times spike or if the service goes down. Logs can help you trace back and understand if an unexpected input format caused the failure or if there was an infrastructure issue. Understanding these processes can:
- Improve collaboration with DevOps.
- Enhance your troubleshooting capabilities.
- Give you visibility into your model's real-world performance.
3. Basics of Data Pipelines
Data pipelines are essential for automating data workflows, especially when preparing data for ML models. While they're often the domain of data engineers, a working knowledge can save you time and enable smoother collaboration.
Data Pipelines vs. CI/CD Pipelines
- Data Pipelines focus on moving, transforming, and processing data. They ensure that raw data from multiple sources is cleaned, aggregated, and ready for analysis or modeling.
- CI/CD Pipelines (Continuous Integration/Continuous Deployment) focus on automating software development workflows, including testing and deploying code.
Tools to Know: Apache Airflow
Apache Airflow is a popular open-source tool for orchestrating workflows. It's highly extensible and used widely in data engineering for tasks like ETL (Extract, Transform, Load).
Key Concepts in Airflow:
- DAGs (Directed Acyclic Graphs): These define workflows as a series of tasks with dependencies. For instance, a pipeline might involve downloading data, cleaning it, training a model, and evaluating its performance.
- Operators: Building blocks of DAGs. Examples include Python operators for custom scripts or Bash operators for shell commands.
- Schedulers: Ensure tasks run at the specified time or based on triggers.
Relevance to Data Science
Consider an ML pipeline where:
- Data ingestion occurs daily from an external API.
- The data is cleaned, aggregated, and stored in a data warehouse.
- The cleaned data triggers model training with the latest batch.
- Predictions are generated and pushed to a dashboard or downstream application.
Airflow can manage the entire pipeline, ensuring reproducibility and minimizing manual intervention. Understanding how these pipelines are built helps you:
- Collaborate with data engineers.
- Design workflows that integrate seamlessly with existing infrastructure.
- Debug issues when data doesn't arrive or is incorrectly processed.
Example workflow
Combining the tools into a seamless pipeline. Developing an ML model → Dockerizing the environment → Setting up CI/CD pipelines with GitHub Actions → Deploying with Flask on AWS → Orchestrating with Kubernetes. Tips for maintaining reproducibility and scalability.
Example Workflow: Combining the Tools into a Seamless Pipeline
Building a successful machine learning (ML) project doesn't end with training a model. In fact, that's often just the beginning. This workflow outlines how you can develop an ML model and deploy it to production while ensuring reproducibility, scalability, and maintainability. Let's break it down step by step:
Step 1: Developing the ML Model
This phase is familiar to most data scientists. It involves data preprocessing, feature engineering, model training, and evaluation. Typically, this work is done in environments like Jupyter Notebooks or integrated development environments (IDEs) such as PyCharm or VSCode.
Tips for this step:
- Use version control for your code and data. Tools like DVC (Data Version Control) are great for tracking datasets and ML experiments.
- Consider libraries like MLflow or Weights & Biases for experiment tracking. These tools help you maintain a record of hyperparameters, metrics, and artifacts.
- Document everything, from preprocessing pipelines to model performance metrics.
Step 2: Dockerizing the Environment
Once the model is trained, the next step is to ensure that your environment is reproducible. This is where Docker comes in. Docker allows you to package your application and its dependencies into a container that can run consistently across different environments.
Creating a Dockerfile:
A Dockerfile
is a script that defines the environment for your application. Below is a minimal example for an ML project:
FROM python:3.9-slim
WORKDIR /app
COPY requirements.txt ./
RUN pip install --no-cache-dir -r requirements.txt
COPY . ./
CMD ["python", "app.py"]
FROM python:3.9-slim
: Specifies the base image with Python installed.WORKDIR /app
: Sets the working directory inside the container.COPY requirements.txt ./
: Copies the dependency file.RUN pip install
: Installs dependencies.CMD ["python", "app.py"]
: Specifies the command to run the application.
Testing Locally: Build and run your Docker container locally to ensure everything works before moving on:
docker build -t my-ml-app .
docker run -p 5000:5000 my-ml-app
Step 3: Setting Up CI/CD Pipelines with GitHub Actions
Continuous Integration/Continuous Deployment (CI/CD) automates the process of testing, building, and deploying your application.
Why GitHub Actions? GitHub Actions integrates seamlessly with GitHub repositories and allows you to define workflows in a YAML file.
Example CI/CD Workflow:
name: CI/CD Pipeline
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v3
- name: Set up Python
uses: actions/setup-python@v3
with:
python-version: 3.9
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: pytest
deploy:
runs-on: ubuntu-latest
needs: build
steps:
- name: Deploy to AWS
run: ./deploy_script.sh
In this example:
- The
build
job checks out the code, sets up Python, installs dependencies, and runs tests. - The
deploy
job runs only if thebuild
job succeeds.
Step 4: Deploying with Flask on AWS
Flask is a lightweight web framework perfect for exposing ML models as REST APIs. Let's look at a basic deployment.
Creating a Flask App: Here's a simple example of a Flask app that serves predictions:
from flask import Flask, request, jsonify
import joblib
app = Flask(__name__)
model = joblib.load("model.pkl")
@app.route('/predict', methods=['POST'])
def predict():
data = request.get_json()
prediction = model.predict([data['features']])
return jsonify({'prediction': prediction.tolist()})
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
Deploying to AWS:
- Set up an EC2 instance: Use an Ubuntu-based instance and install Docker.
- Run the Flask app inside a Docker container: Use the Docker image you created earlier.
Example deployment script:
#!/bin/bash
docker pull my-ml-app
sudo docker run -d -p 80:5000 my-ml-app
Step 5: Orchestrating with Kubernetes
Kubernetes (K8s) is essential for managing containerized applications at scale. While it's primarily a DevOps tool, data scientists can benefit from knowing its basics.
Key Concepts:
- Pods: The smallest deployable units in Kubernetes. Each pod contains one or more containers.
- Deployments: Declarative updates for pods.
- Services: Expose your application to the network.
Deploying an ML Model with Kubernetes:
Here's an example deployment.yaml
:
apiVersion: apps/v1
kind: Deployment
metadata:
name: ml-model-deployment
spec:
replicas: 3
selector:
matchLabels:
app: ml-model
template:
metadata:
labels:
app: ml-model
spec:
containers:
- name: ml-model
image: my-ml-app
ports:
- containerPort: 5000
Apply this configuration using:
kubectl apply -f deployment.yaml
Use a Kubernetes service to expose the deployment:
apiVersion: v1
kind: Service
metadata:
name: ml-model-service
spec:
selector:
app: ml-model
ports:
- protocol: TCP
port: 80
targetPort: 5000
type: LoadBalancer
Tips for Reproducibility and Scalability
-
Reproducibility:
- Pin dependency versions in your
requirements.txt
. - Use container registries (e.g., Docker Hub) to store and version Docker images.
- Document all configurations and environment variables.
- Pin dependency versions in your
-
Scalability:
- Use horizontal scaling (e.g., adding more replicas in Kubernetes) for handling increased load.
- Leverage managed services like AWS Elastic Kubernetes Service (EKS) to simplify Kubernetes management.
- Monitor system performance using tools like Prometheus and Grafana.
By combining these tools and techniques, you can create a seamless ML pipeline that's not only robust but also ready for real-world production environments.