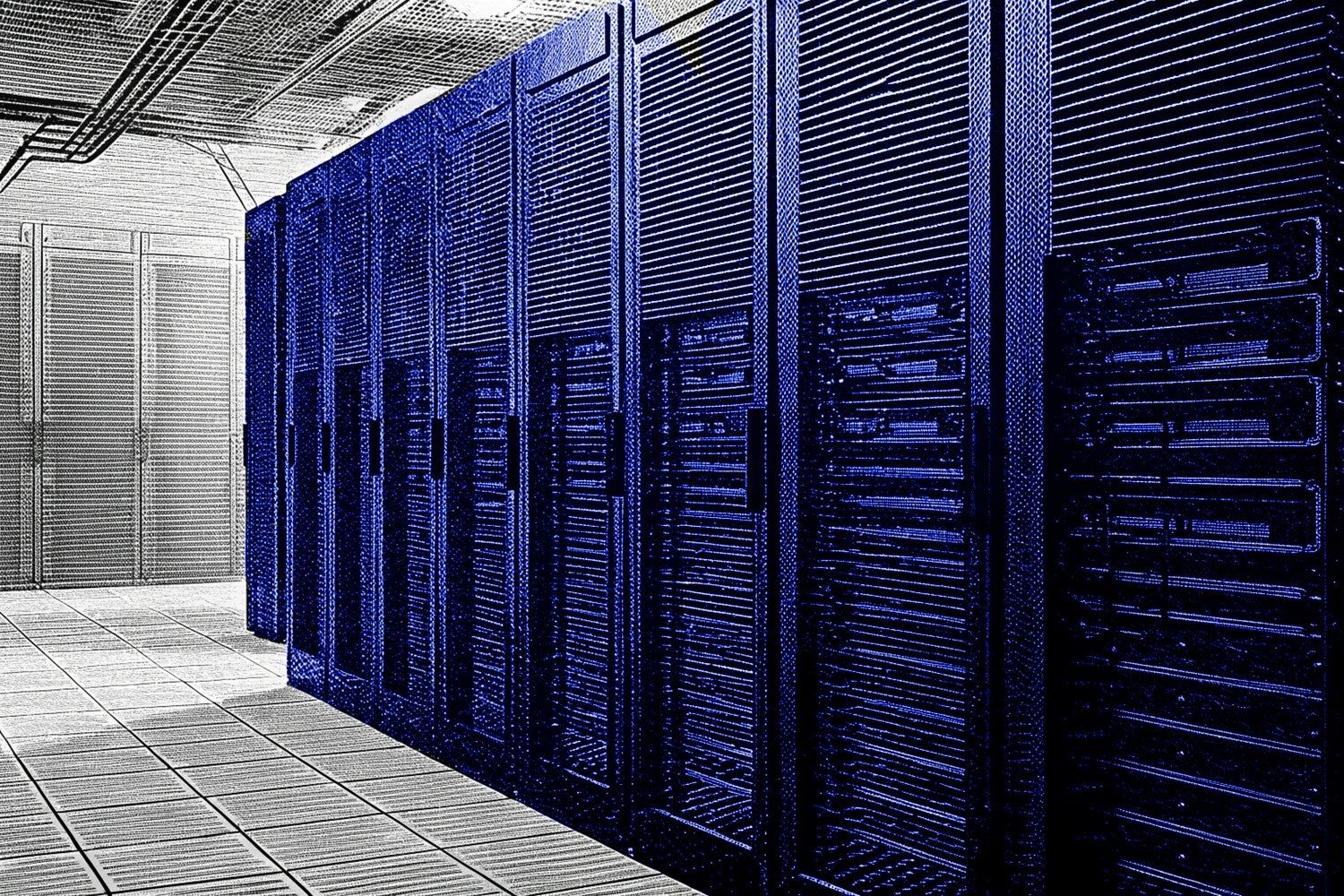
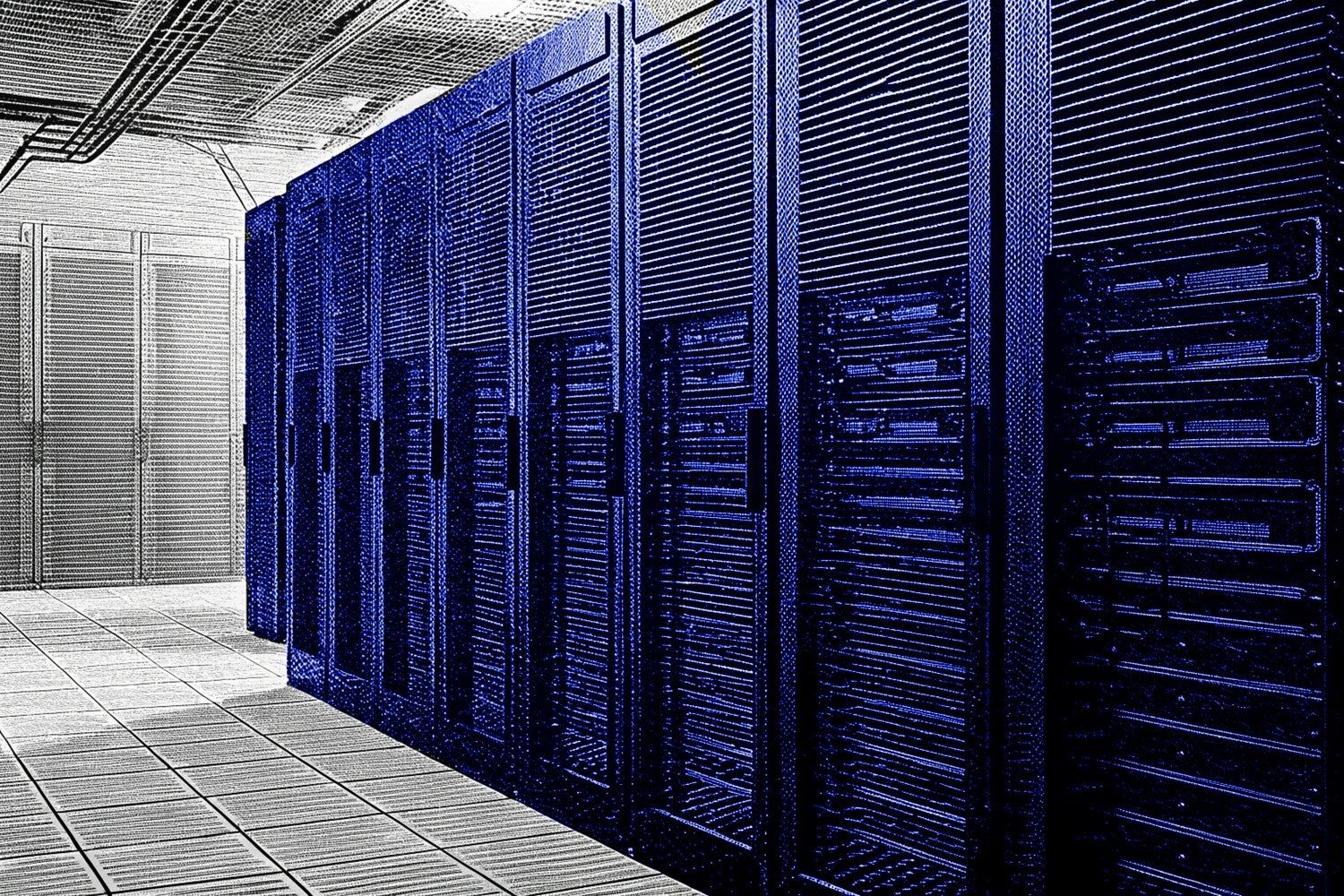
🎓 41/167
This post is a part of the Working with data educational series from my free course. Please keep in mind that the correct sequence of posts is outlined on the course page, while it can be arbitrary in Research.
I'm also happy to announce that I've started working on standalone paid courses, so you could support my work and get cheap educational material. These courses will be of completely different quality, with more theoretical depth and niche focus, and will feature challenging projects, quizzes, exercises, video lectures and supplementary stuff. Stay tuned!
Modern data science pipelines rely heavily on the ability to store, retrieve, transform, and analyze vast quantities of data in an efficient, organized manner. While many data scientists initially focus on the modeling aspect — designing and training sophisticated machine learning algorithms — the underlying data infrastructure is often an equally significant factor in determining the success or failure of a project. Reliable data storage and management systems enable consistent data access for teams, while poor data infrastructure may lead to inefficient workflows, inaccurate results, or lost opportunities.
I have seen scenarios where raw data was stored haphazardly in individual CSV files scattered across a team's internal file-sharing system. Collaboration was painful, data consistency was questionable, and version control proved nearly impossible. In such situations, a well-designed database could have smoothed out these rough edges. Databases also help organizations maintain strong governance over data, preventing duplication and corruption while ensuring that essential information is accurately preserved. In addition, a robust data storage strategy can simplify tasks such as logging, auditing, securing, and tracking changes over time.
From a business perspective, databases allow multiple stakeholders — analysts, data scientists, operations specialists, and executives — to interact with the same centralized source of truth. This common data platform serves as a foundation for consistent reporting, dashboards, and predictive models that shape strategic decision-making. Meanwhile, in an ever more competitive and data-driven world, ensuring that the right data is accessible at the right time to the right individuals is essential for maintaining a competitive advantage.
For data science projects, databases serve as a critical part of the data pipeline, tying together ingestion, transformation, and analysis processes. They form the architectural backbone that supports everything from rapid prototyping of machine learning models to real-time analytics, recommendation engines, and AI-driven decision systems.
relational vs. nosql databases
Relational Database Management Systems (RDBMS) utilize a highly structured approach to data storage, typically enforcing a schema that defines how the data is organized in tables, how those tables relate to each other, and what constraints each field must respect. This rigid structure, and the powerful Structured Query Language (Commonly referred to simply as SQL) that comes with it, is often an advantage for many enterprise-level applications. Examples of relational databases include PostgreSQL, MySQL, Oracle Database, and Microsoft SQL Server.
On the other hand, NoSQL databases are typically designed to handle large volumes of unstructured or semi-structured data, offering greater flexibility in data schema, horizontal scalability across clusters of nodes, and performance optimizations for specific access patterns. They come in various flavors — such as document stores (MongoDB, CouchDB), key-value stores (Redis, Riak), column-family stores (Cassandra, HBase), and graph databases (Neo4j). Because they can eschew some of the consistency requirements of relational systems, many NoSQL platforms can distribute data more widely, handle massive workloads, and reduce latency under huge loads.
Researchers have identified a general design principle known as the CAP theorem (Brewer, 2000) that states: in a distributed data store, you can only guarantee two of the following three properties at the same time: Consistency, Availability, and Partition tolerance. Traditional RDBMS solutions tend to focus on consistency, while many NoSQL solutions focus on availability and horizontal scalability, particularly under partitioned network environments.
In data science contexts, the best choice between relational and NoSQL systems often depends on the nature of the data, the scale of operations, and the specific requirements of analysis. While large-scale clickstream logs may warrant a flexible schema in a high-throughput NoSQL platform, structured business transactions typically benefit from the reliability and consistency of relational systems.
why focus on postgresql for data science
PostgreSQL stands out among relational databases for a variety of reasons, making it highly appealing for data science projects. Unlike some proprietary solutions, PostgreSQL is open source, meaning it has a rich, vibrant community of contributors and a continuously evolving ecosystem of tools and extensions. PostgreSQL often implements advanced features ahead of other open-source relational databases — such as support for window functions, Common Table Expressions, multi-version concurrency control (MVCC) for high levels of concurrency, and an extensible type system.
Notably, PostgreSQL supports powerful geospatial capabilities through the PostGIS extension, advanced text search, and even a growing ecosystem of machine learning extensions (for instance, there are attempts to build in-database ML capabilities, though these are still somewhat experimental). Its high compliance with SQL standards, combined with an emphasis on reliability and data integrity, ensures that data scientists can rely on stable SQL features, strong ACID (Atomicity, Consistency, Isolation, Durability) guarantees, and a robust user management system.
These core advantages make PostgreSQL a prime candidate for handling structured data within many data pipelines. While data scientists often use Python or R for model building, they need a trustworthy relational store to handle critical data that requires transactional consistency, joins, or complex analytical queries. PostgreSQL's ecosystem — combined with its well-tested performance and flexibility — typically satisfies these demands.
role of databases in data pipelines
Databases sit at the heart of many data pipelines, which may include:
- Extraction of raw data from multiple sources (APIs, logs, sensors, third-party databases).
- Transformation of data through cleaning, normalization, and feature engineering.
- Loading the results into analytical stores, data warehouses, or data lakes for further processing.
In a typical scenario, data may be extracted from operational systems (like an e-commerce platform) via batch jobs or real-time streams, validated and cleaned in transit, then loaded into PostgreSQL or another database for further analysis. The database may also serve as a staging area for downstream machine learning tasks, where the clean data is extracted again, fed into modeling pipelines, or integrated with third-party analytics tools. Once the results are generated (for example, churn predictions or product recommendations), the final outcomes might be written back to the database, enabling dashboards, audits, or updates to user-facing applications.
This cyclical interplay between databases, transformation layers, and analytics engines underscores how critical database design and optimization are in building high-quality data science solutions. An ill-designed database schema or insufficient indexing strategy can substantially hamper the performance and reliability of an otherwise well-designed data science project. By contrast, a carefully engineered database system can yield a stable foundation for experimentation, iteration, and real-time decision-making.
relational databases and postgresql
core concepts of sql
Structured Query Language (SQL) is a domain-specific language used in programming and designed for managing data held in a relational database management system. It facilitates:
- Data Definition (creating, altering, dropping database objects such as tables, indexes, or triggers).
- Data Manipulation (inserting, updating, deleting rows).
- Data Querying (retrieving records from one or multiple tables).
- Data Control (managing user privileges, transactions, locks).
SQL queries often follow a standard pattern:
<Code text={`
SELECT columns
FROM table
WHERE condition
GROUP BY some_column
HAVING group_condition
ORDER BY some_column [ASC | DESC]
`}/>
This structure offers data scientists significant flexibility in slicing, dicing, and aggregating data. For example, one could quickly compute the average number of items purchased per user in a dataset of transactions. SQL also supplies powerful tools like joins (INNER, LEFT, RIGHT, FULL) that help merge multiple tables based on matching keys, simplifying the process of weaving together various aspects of an organization's data.
Consider a typical scenario in which you have two tables: users and orders. The following SQL snippet demonstrates joining them to retrieve users and their total order amounts:
<Code text={`
SELECT
u.user_id,
u.name,
SUM(o.order_total) AS total_spent
FROM users AS u
INNER JOIN orders AS o
ON u.user_id = o.user_id
GROUP BY u.user_id, u.name
ORDER BY total_spent DESC;
`}/>
Here, we're linking each user to their orders using user_id, then aggregating total spending using SUM, grouping the results by user ID and sorting them based on total spending. Such expressions can get much more complex in practice, but the fundamental concept remains: we can combine data in meaningful ways with just a few lines of SQL.
schema design fundamentals
Database schema design determines how tables, relationships, constraints, and indexes are structured, and is a critical aspect of building robust data systems. The main considerations include:
-
Normalization: The idea of organizing columns and tables to reduce data redundancy and improve data integrity. Early normal forms (1NF, 2NF, 3NF) aim to ensure that each table focuses on a specific entity, and that attributes are properly broken out so as not to repeat the same information across multiple tables unnecessarily.
-
Relationships: Relational databases represent associations between entities using primary and foreign key constraints. For instance, each order might contain a user_id that references the primary key in the users table. This ensures referential integrity — that no order can reference a user that does not exist.
-
Indexes: Indexes speed up data retrieval at the cost of additional storage and overhead when inserting/updating records. Common indexing strategies include B-tree indexes for equality and range queries, or specialized indexes like GIN (Generalized Inverted Index) or GiST (Generalized Search Tree) for text search or geospatial queries. Proper indexing can drastically reduce query times, but over-indexing can slow down write operations and cause maintenance headaches.
-
Denormalization: In certain analytical workloads, you might intentionally duplicate or flatten data for faster read performance. This typically happens in data warehousing or real-time analytics scenarios where query performance is more important than strict normalization rules. Denormalizing can reduce the overhead of complex joins in queries that must aggregate or filter billions of records.
A well-designed schema is the foundation upon which everything else in your data pipeline rests. Understanding the trade-offs between normalization and denormalization, the appropriate use of relationships, and strategic indexing is essential for building high-performance data applications.
query optimization techniques
Even the most elegantly designed schema can exhibit performance bottlenecks if queries are not planned and optimized properly. PostgreSQL uses a cost-based query optimizer that considers multiple execution plans and picks the most efficient one. Key aspects of query optimization include:
-
Indexing: Ensure that columns frequently used in filters or joins have suitable indexes. Additionally, partial indexes can be created for selective queries, and advanced indexing strategies — such as BRIN (Block Range Index) — can optimize range queries for very large tables.
-
Analyzing query plans: PostgreSQL offers the EXPLAIN command that gives insights into the query plan. By running EXPLAIN ANALYZE, you can see the actual execution path used, plus detailed timing statistics. This helps identify slow steps, suboptimal join algorithms (nested loop, hash, or merge), and indicates whether indexes are used.
-
Vacuuming and statistics: PostgreSQL uses VACUUM (and AUTOVACUUM) to clean up stale row versions left behind by its MVCC mechanism. Ensuring that vacuum jobs run consistently helps maintain healthy performance. Meanwhile, the ANALYZE operation updates table statistics, guiding the optimizer to make better decisions about the most efficient access paths.
-
Avoiding unnecessary data movement: Minimizing the amount of data processed or transferred through the pipeline is crucial. This can include pushing down filters or aggregations as close to the data source as possible, and projecting only the needed columns instead of selecting * (all columns).
-
Materialized views: For repetitive, expensive queries (e.g., large aggregations on a massive table), storing the precomputed result in a materialized view may be worthwhile. The
Materialized views can be refreshed, but they are not automatically updated whenever the underlying tables change. Minimizing overhead is key; if your data updates frequently, you have to maintain a balance between the cost of refreshing vs. the performance gained.
In the formula above, each phase of the query (like scanning a table, joining two sets of records, or sorting results) contributes to a total cost that the optimizer attempts to minimize. The weights for CPU, I/O, memory, and other factors come from PostgreSQL's internal cost model. Fine-tuning these can improve the planner's ability to choose optimal strategies.
installing and setting up postgresql
Getting started with PostgreSQL involves:
-
Installation: On Linux, you might use a package manager such as apt or yum:
<Code text={` sudo apt-get update sudo apt-get install postgresql postgresql-contrib `}/>
On macOS, you could use Homebrew:
<Code text={` brew update brew install postgresql `}/>
Windows users can grab an official installer from the PostgreSQL website.
-
Initialization: PostgreSQL typically starts a service/daemon in the background. You can check its status or configure auto-start, as needed. The default administrative user is often
postgres
. -
Creating a Database:
<Code text={` sudo -u postgres psql CREATE DATABASE my_database; CREATE USER my_user WITH PASSWORD 'my_password'; GRANT ALL PRIVILEGES ON DATABASE my_database TO my_user; `}/>
-
Configuration: In the
postgresql.conf
andpg_hba.conf
files, you can enable remote connections, configure memory usage parameters likeshared_buffers
,work_mem
, or set up replication parameters. -
Connecting from Python:
<Code text={` import psycopg2 conn = psycopg2.connect( dbname="my_database", user="my_user", password="my_password", host="localhost", port="5432" ) cursor = conn.cursor() cursor.execute("SELECT version();") print(cursor.fetchone()) cursor.close() conn.close() `}/>
Once set up, you can dive into deeper configuration details, enabling advanced extensions or fine-tuning performance for your specific workloads.
basic sql commands and usage
In PostgreSQL, typical commands for daily data manipulation include:
-
CREATE TABLE: Defines a new table.
<Code text={` CREATE TABLE sales ( sale_id SERIAL PRIMARY KEY, product_id INT NOT NULL, quantity INT NOT NULL, sale_date DATE NOT NULL ); `}/>
-
INSERT: Inserts a new row.
<Code text={` INSERT INTO sales (product_id, quantity, sale_date) VALUES (101, 3, '2025-01-10'); `}/>
-
UPDATE: Updates existing rows.
<Code text={` UPDATE sales SET quantity = quantity + 1 WHERE product_id = 101; `}/>
-
DELETE: Removes rows.
<Code text={` DELETE FROM sales WHERE sale_date < '2024-12-31'; `}/>
-
SELECT: Retrieves data.
<Code text={` SELECT product_id, SUM(quantity) AS total_sold FROM sales GROUP BY product_id ORDER BY total_sold DESC; `}/>
-
CREATE INDEX: Creates an index to speed up queries.
<Code text={` CREATE INDEX idx_product_id ON sales (product_id); `}/>
Over time, you'll likely expand your toolkit to include advanced joins, subqueries, window functions (like ROW_NUMBER, RANK, LAG), and other analytical capabilities that PostgreSQL supports.
transactions and concurrency control
PostgreSQL adheres to ACID properties:
- Atomicity: A transaction is treated as a single unit of work; either all operations succeed or all fail.
- Consistency: A transaction takes the database from one valid state to another, enforcing constraints and triggers.
- Isolation: Concurrent transactions do not affect each other's intermediate states. PostgreSQL implements Multi-Version Concurrency Control (MVCC), which avoids read locks by presenting each transaction with a consistent snapshot of the data.
- Durability: Once a transaction commits, the data is guaranteed to persist, even in the event of a crash (within the constraints of write-ahead logging).
A simple PostgreSQL transaction can be shown as:
<Code text={`
BEGIN;
INSERT INTO sales (product_id, quantity, sale_date)
VALUES (101, 2, '2025-02-14');
UPDATE inventory
SET stock = stock - 2
WHERE product_id = 101;
COMMIT;
`}/>
Here, if either the INSERT or the UPDATE statement fails, the entire transaction can be rolled back, leaving the database in its previous consistent state. By default, PostgreSQL runs each statement as a separate transaction if one is not explicitly started. For complex or multiple-step operations, you'll often explicitly start a BEGIN
block and finish with either COMMIT
or ROLLBACK
.
In terms of isolation levels, PostgreSQL supports:
- Read Uncommitted (though internally it treats it as Read Committed)
- Read Committed
- Repeatable Read
- Serializable
As you move from Read Committed to Serializable, you reduce phenomena like non-repeatable reads but potentially increase concurrency conflicts if transactions attempt to modify overlapping data.
advanced features of postgresql
PostgreSQL's extensibility is one of its core strengths. Popular advanced features include:
- Extensions: PostGIS (geospatial queries), full-text search, hstore (key-value within a column), and more.
- Partitioning: Large tables can be partitioned by range or list, improving query performance by scanning only relevant partitions.
- Stored Procedures & Functions: Written in
Procedural Language/PostgreSQL (PL/pgSQL) or other languages like Python (PL/Python), enabling complex logic to reside on the database side. This can reduce round-trips between applications and the DB.
- Triggers: Logic automatically executed before or after certain statements or row changes. For example, triggers can record audit logs or enforce custom constraints.
- Foreign Data Wrappers: Access external data sources (like CSV files, another database, or even an API) as if they were local tables.
For data scientists, the ability to embed advanced logic or efficiently handle semi-structured data can greatly streamline certain steps that might otherwise require an external process. You can, for instance, load large geospatial datasets and run advanced location-based queries entirely within the database, returning only the aggregated or filtered results to your application.
high availability and replication
Many production environments require high availability (HA) to ensure minimal downtime. PostgreSQL offers different replication strategies:
- Streaming replication (physical replication): Writes the changes from the primary to one or more standby servers. If the primary fails, a standby can be promoted.
- Logical replication: Replicates data based on the logical structure (table contents), enabling replication of specific tables or partial subsets.
- Synchronous vs. asynchronous: Synchronous replication ensures that a transaction is not considered committed until at least one standby confirms receipt of the data, guaranteeing zero data loss. Asynchronous replication has lower latency but may risk a small amount of data loss in the event of a catastrophic primary failure.
Clustering solutions like Patroni or external frameworks like Citus can coordinate failovers, provide load balancing, or distribute data across nodes. These are critical in large-scale or mission-critical data science applications where downtime or data loss is unacceptable.
nosql databases
key principles of nosql
NoSQL emerged partly in response to the limitations that relational databases faced when scaling horizontally across many commodity servers. Some guiding principles:
- Schema flexibility: Tables need not have rigid column definitions; new attributes can be added dynamically, which is particularly helpful for rapidly evolving applications or data ingestion from varied sources.
- Horizontal scalability: Distribute data across multiple nodes in a cluster, enabling near-linear scaling as data volumes grow.
- Eventual consistency: Many NoSQL systems relax strict consistency to achieve better availability and partition tolerance. Writes propagate asynchronously, and replicas eventually converge to a consistent state.
This approach suits many big data use cases, such as user activity streams, sensor logs, social media feeds, or product catalogs with frequently changing attributes.
mongodb basics
MongoDB is a document-oriented database that stores data in collections of documents (similar to JSON objects). For instance:
<Code text={`
{
"_id": "6093a0...",
"name": "Alice",
"purchases": [
{ "product_id": 101, "quantity": 2 },
{ "product_id": 202, "quantity": 1 }
]
}
`}/>
Documents allow variable structures; you can nest arrays or objects, providing a high degree of flexibility. MongoDB provides a JavaScript-like query language, indexes (including text and geospatial), sharding for horizontal scaling, and replication for HA. Data scientists might use MongoDB for rapidly evolving applications or for storing complex objects that do not map neatly into relational tables.
cassandra fundamentals
Apache Cassandra is a column-family store. It organizes data into column families, similar to tables, but uses a decentralized architecture with no single point of failure. The data is automatically partitioned across the cluster according to a partition key. Cassandra is particularly well-suited for high-velocity, write-heavy workloads where nodes can quickly accept writes and replicate them across the ring. It also excels in multi-data-center or multi-region setups, due to tunable consistency and robust fault tolerance.
key-value stores
Key-value stores like Redis or Riak store data as a simple map of keys to values. While minimalistic, they excel in caching, session management, or real-time analytics scenarios. Redis, in particular, offers in-memory speed, built-in data structures (lists, sets, sorted sets), and pub-sub capabilities. Some data scientists incorporate Redis as a near-real-time layer for ephemeral data, such as storing feature values or partial aggregates for frequently updated dashboards.
sharding and replication in nosql
Sharding is central to scaling many NoSQL databases. Data is split across shards, each responsible for a subset of the data, often determined by a hash or range partition of the key. Replication then ensures redundancy and availability, so each shard is typically replicated to multiple nodes. If a node fails, replicas can serve requests.
<Image alt="Horizontal scaling illustration" path="" caption="Illustration of horizontal scaling with multiple shards and replicas." zoom="false" />
In the above image, each shard is replicated multiple times. A coordinator node (or a partition-aware driver) routes incoming read/writes to the appropriate shard. This approach works well when queries focus on specific partitions of data, but cross-shard queries can become more complex. Data scientists should consider this trade-off when designing queries for horizontally partitioned NoSQL systems.
when to choose nosql
While relational databases remain a strong choice for strongly consistent transactional workloads, NoSQL solutions become attractive in scenarios where:
- Huge data volume that must be distributed across multiple nodes.
- Rapid schema evolution is required, or the data is highly unstructured.
- Real-time streaming data with extremely high ingestion rates.
- Global distribution or low-latency reads/writes from multiple geographic regions.
However, the lack of strong consistency guarantees in some NoSQL platforms can complicate analytics. If you need complex joins or transactions across multiple entities, a relational approach may still be more natural. In many modern data science architectures, a polyglot persistence model can combine the strengths of both relational and NoSQL systems.
data warehousing
basics of olap systems
Traditional relational databases often excel at Online Transaction Processing (OLTP) with numerous small, concurrent operations (inserts, updates, short queries) and strict consistency requirements. By contrast, Online Analytical Processing (
OLAP) centers on large-scale queries, aggregates, and historical analysis. Data warehousing solutions such as Amazon Redshift, Snowflake, or on-premises solutions like Teradata or Oracle Exadata focus on OLAP workloads by optimizing for:
- Columnar storage: Minimizes I/O when scanning large volumes of data for select columns.
- Massively parallel processing (
MPP): Distributing computations across multiple nodes.
- Star or snowflake schemas: Simplified denormalized designs for efficient aggregations. Fact tables store events (e.g., sales), and dimension tables store descriptive attributes (e.g., products, regions).
In an OLAP scenario, queries might join a fact table with multiple dimension tables to calculate aggregated metrics over large time ranges, such as monthly sales by region or year-over-year comparisons.
data lake vs. data warehouse
A data warehouse is typically a structured environment with carefully modeled schemas, curated data sets, and a strong emphasis on data quality. A data lake, on the other hand, is a more flexible, file-based repository (often in distributed storage like HDFS or Amazon S3), allowing ingestion of raw or lightly processed data of various formats (JSON, CSV, logs, images, etc.). The trade-offs:
- Data Warehouse:
- Structured, consistent schemas and queries
- High performance for analytical queries
- Potentially high upfront modeling and ETL overhead
- Data Lake:
- Flexible, supports varied data formats
- Can store raw data cheaply
- Requires additional steps or specialized engines (like Spark, Presto) for analytics
In many data science ecosystems, the lines can blur. A lakehouse architecture (Databricks and others) tries to combine the best of both worlds, with structured overlays on top of raw data storage and optimized query engines.
integrating postgresql in data warehousing
Although PostgreSQL is generally not labeled a data warehouse solution, it can serve smaller analytic workloads effectively. With the advent of features like table partitioning, indexing strategies, and parallel queries, PostgreSQL can handle moderate volumes of data for analysis. Extensions like Citus aim to distribute PostgreSQL across multiple nodes, effectively making it a horizontally scalable, PostgreSQL-compatible environment that can handle more extensive workloads. In data science workflows, PostgreSQL is often used as a staging area or a key component of the data lakehouse environment, acting as a source or sink for processed datasets.
etl and elt strategies
Data engineering practices revolve around moving data from source systems into analytics or modeling environments:
- ETL (Extract, Transform, Load): Data is extracted from source(s), transformed into the required format or schema, then loaded into a target (e.g., a data warehouse).
- ELT (Extract, Load, Transform): Data is first extracted and loaded in its raw or near-raw state into a data lake or big data platform, then transformations happen afterward, typically leveraging powerful distributed processing engines.
The choice often depends on the complexity of transformations, data volumes, and the tools available in the ecosystem. For instance, using PostgreSQL in a pipeline might entail:
- Extracting logs from an application server.
- Loading them into a staging table in PostgreSQL for cleaning.
- Applying transformations (e.g., splitting columns, converting types).
- Aggregating data.
- Exporting final results into an analytics environment or a machine learning feature store.
file systems and storage
efficient handling of large-scale data
As data volumes scale, naive approaches — like keeping all data in a single CSV file or in memory — become unsustainable. Data scientists must consider:
- Batch vs. streaming: Large batch uploads (ETL) vs. incremental or real-time ingestion (stream processing).
- Compression: Use columnar formats (Parquet, ORC) or compressed text formats (GZIP, LZ4) to save storage and minimize I/O costs.
- Partitioning: Organize data by date, region, or other criteria so queries can prune irrelevant partitions.
overview of distributed file systems (e.g., hadoop hdfs)
Distributed file systems like Hadoop Distributed File System (HDFS) store data across multiple nodes, handling replication, fault tolerance, and parallel processing. MapReduce introduced a programming paradigm for analyzing these large datasets in a distributed manner, though modern ecosystems often use higher-level abstractions (Apache Spark, Hive, or Impala) for improved developer productivity and performance.
cloud storage solutions and best practices
Commercial cloud providers (AWS S3, Google Cloud Storage, Azure Blob Storage) have become go-to solutions for storing massive amounts of data. Key advantages include pay-as-you-go pricing, built-in redundancy and durability (e.g., Amazon's claim of 99.999999999% durability), and easy integration with managed analytics services. Best practices often involve:
- Lifecycle policies: Automatically move older data to cheaper storage classes.
- Server-side encryption: Protect data at rest, ensuring compliance with security standards.
- Identity and Access Management: Strict control of who can read or write to the buckets.
hybrid storage models
Some enterprises maintain on-premises data centers for sensitive or mission-critical workloads, while leveraging cloud services for scale-out analytics or backup. Hybrid strategies balance the compliance and performance needs of on-premises systems with the flexibility and elasticity of the cloud. Technologies like AWS Outposts or Azure Stack let organizations run cloud-like services on premises, bridging these environments further.
practical applications in data science
etl (extract, transform, load) workflows
A typical data science workflow might involve:
- Extract: Pull data from an API, a CSV file, or another database.
- Transform: Clean missing values, convert data types, engineer features, remove outliers.
- Load: Insert the processed data into PostgreSQL or another database for final consumption.
<Code text={`
import pandas as pd
import psycopg2
# 1. Extract
df = pd.read_csv("transactions.csv")
# 2. Transform
df = df.dropna(subset=['user_id', 'amount'])
df['amount'] = df['amount'].apply(lambda x: max(x, 0)) # remove negative
# 3. Load into PostgreSQL
conn = psycopg2.connect(
dbname="my_database",
user="my_user",
password="my_password",
host="localhost",
port="5432"
)
cursor = conn.cursor()
for index, row in df.iterrows():
cursor.execute(
"INSERT INTO transactions (user_id, amount, transaction_date) VALUES (%s, %s, %s)",
(row['user_id'], row['amount'], row['transaction_date'])
)
conn.commit()
cursor.close()
conn.close()
`}/>
By automating these steps in a robust workflow orchestration tool (e.g., Airflow, Luigi, Prefect), you ensure consistent, reliable data ingestion, which is vital to maintaining trust in the data science outputs.
data cleaning and preparation using sql
SQL remains a powerful language for data cleaning. Sometimes it's easier to let the database handle filtering or transformations if the data is already in a relational format. For instance:
<Code text={`
UPDATE transactions
SET amount = 0
WHERE amount < 0;
`}/>
You can also use CASE expressions in SELECT
queries to recast categories, remove outliers, or fill missing data. Coupled with window functions, you can quickly find and eliminate anomalies or duplicates. The database engine can handle these operations very efficiently, especially if the relevant columns are properly indexed or partitioned.
advanced analytics with postgresql
PostgreSQL supports:
- Window functions:
ROW_NUMBER()
,RANK()
,DENSE_RANK()
,LAG()
,LEAD()
, enabling advanced ranking, cumulative sums, or rolling averages. - Common Table Expressions (CTEs): Write modular queries, break complex logic into smaller pieces, or recursively traverse hierarchical data.
- Geospatial queries: PostGIS extension with distance calculations, bounding boxes, and geometric shapes.
- Full-text search: Allows complex text-based queries with ranking of relevance.
For instance, the following advanced analytics query calculates a weekly rolling average of sales:
<Code text={`
WITH weekly_sales AS (
SELECT
date_trunc('week', sale_date) AS week_start,
SUM(quantity) AS weekly_quantity
FROM sales
GROUP BY date_trunc('week', sale_date)
ORDER BY date_trunc('week', sale_date)
)
SELECT
week_start,
weekly_quantity,
AVG(weekly_quantity) OVER (
ORDER BY week_start
ROWS BETWEEN 3 PRECEDING AND CURRENT ROW
) AS rolling_avg_4_weeks
FROM weekly_sales;
`}/>
This query first aggregates weekly sales, then uses a window function to compute a rolling average over the last 4 weeks of data. Such queries might be used for forecasting, anomaly detection, or seasonality analysis in data science projects.
combining relational and nosql data sources
In modern polyglot persistence designs, it's common to store some data in a relational database (e.g., transactional data in PostgreSQL) while other data (e.g., logs, documents) reside in a NoSQL store like MongoDB. Data scientists might combine these sources during analysis, using frameworks that can join data at the application or distributed compute layer. Alternatively, you can use foreign data wrappers in PostgreSQL to query external NoSQL data directly:
<Code text={`
CREATE EXTENSION mongo_fdw;
CREATE SERVER mongo_server
FOREIGN DATA WRAPPER mongo_fdw
OPTIONS (address 'mongo_host', port '27017');
CREATE FOREIGN TABLE external_collection (
...
) SERVER mongo_server OPTIONS (database 'mydb', collection 'mycollection');
`}/>
With these steps, you can effectively query your MongoDB collection as if it were part of PostgreSQL. Although performance might vary, the approach often simplifies data exploration.
real-time analytics
Databases can also be integrated with streaming technologies (Apache Kafka, Amazon Kinesis) to ingest real-time events. PostgreSQL triggers or logical decoding can capture row changes and publish them to a message queue, enabling near-instant downstream consumption. Alternatively, event streaming platforms can feed data into a real-time analytics engine, which then stores summaries or aggregates back to the database for consumption by dashboards or machine learning pipelines.
best practices and future trends
query tuning and performance optimization
For best performance in data-intensive applications:
- Index proactively but selectively: Identify high-impact columns used in WHERE clauses or joins, but avoid over-indexing tables with frequent writes.
- Partition large tables: Break them up by date or other criteria to reduce scanning overhead.
- Monitor query plans: Use
EXPLAIN ANALYZE
to identify bottlenecks and track down suboptimal scans. - Leverage caching: Both at the database layer (e.g., query caching, increased shared buffers) and in the application layer (e.g., Redis for ephemeral caching).
- Optimize hardware resources: Ensure adequate CPU, memory, and disk I/O. Postgres can be sensitive to disk performance, so using SSDs or appropriate RAID configurations is often essential.
security and compliance considerations
Data scientists often deal with sensitive personal data (e.g., health records, financial transactions). Protecting this data is paramount:
- Encryption at rest (disk-level or tablespace-level encryption).
- Encryption in transit (TLS/SSL connections).
- Role-based access control: Granular privileges so each user has only the minimum permissions necessary.
- Audit logging: Track who changes data or queries sensitive tables.
- Regulatory compliance: For instance, ensuring compliance with HIPAA (healthcare) or GDPR (European data protection) can require specialized data handling or anonymization.
emerging trends in database technologies
Several new trends promise to reshape database and data management ecosystems in data science:
- Serverless databases: Offer automatic scaling and pay-per-usage, exemplified by Amazon Aurora Serverless or Azure SQL Database serverless.
- Multi-model databases: Single systems that natively handle multiple data models (document, graph, relational) under one engine, like ArangoDB or OrientDB.
- AI-powered query optimization: Research (Smith and gang, NeurIPS 2022) hints at the potential for machine learning to guide or even replace cost-based optimizers for certain workloads, potentially leading to real-time adaptive query plans.
- New storage engines: Hybrid row-columnar solutions or distributed PostgreSQL variants like Citus that aim to handle both OLTP and OLAP workloads efficiently.
- SQL on everything: Tools that let you run SQL queries on data in file systems, streams, or specialized data stores (Trino, Presto, Apache Drill), bridging the gap between structured and unstructured data.
conclusion and next steps
Databases remain a cornerstone of any robust data science project. PostgreSQL's advanced feature set, strong compliance with SQL standards, and vibrant open-source ecosystem make it a solid backbone for many analytical tasks. When combined with NoSQL solutions, data warehouses, and distributed file systems, you can craft end-to-end data architectures that seamlessly handle everything from real-time data ingestion to batch analytics and advanced machine learning.
Going forward, it's worthwhile to:
- Deepen your understanding of PostgreSQL internals: concurrency, indexing, partitioning strategies.
- Explore advanced analytics: window functions, CTEs, geospatial queries, and specialized extensions.
- Investigate modern data stack components: cloud object storage, distributed computing frameworks like Spark, and streaming tools like Kafka for real-time pipeline design.
- Stay informed about emerging trends, from AI-driven optimizers to new multi-model solutions.
A well-planned database architecture and a grasp of SQL best practices will help you — as a data scientist or data engineer — to unlock faster, more accurate insights while ensuring that your organization's data remains organized, consistent, and secure. By laying this foundational groundwork, you position yourself to build sophisticated, scalable, and future-ready data science applications.