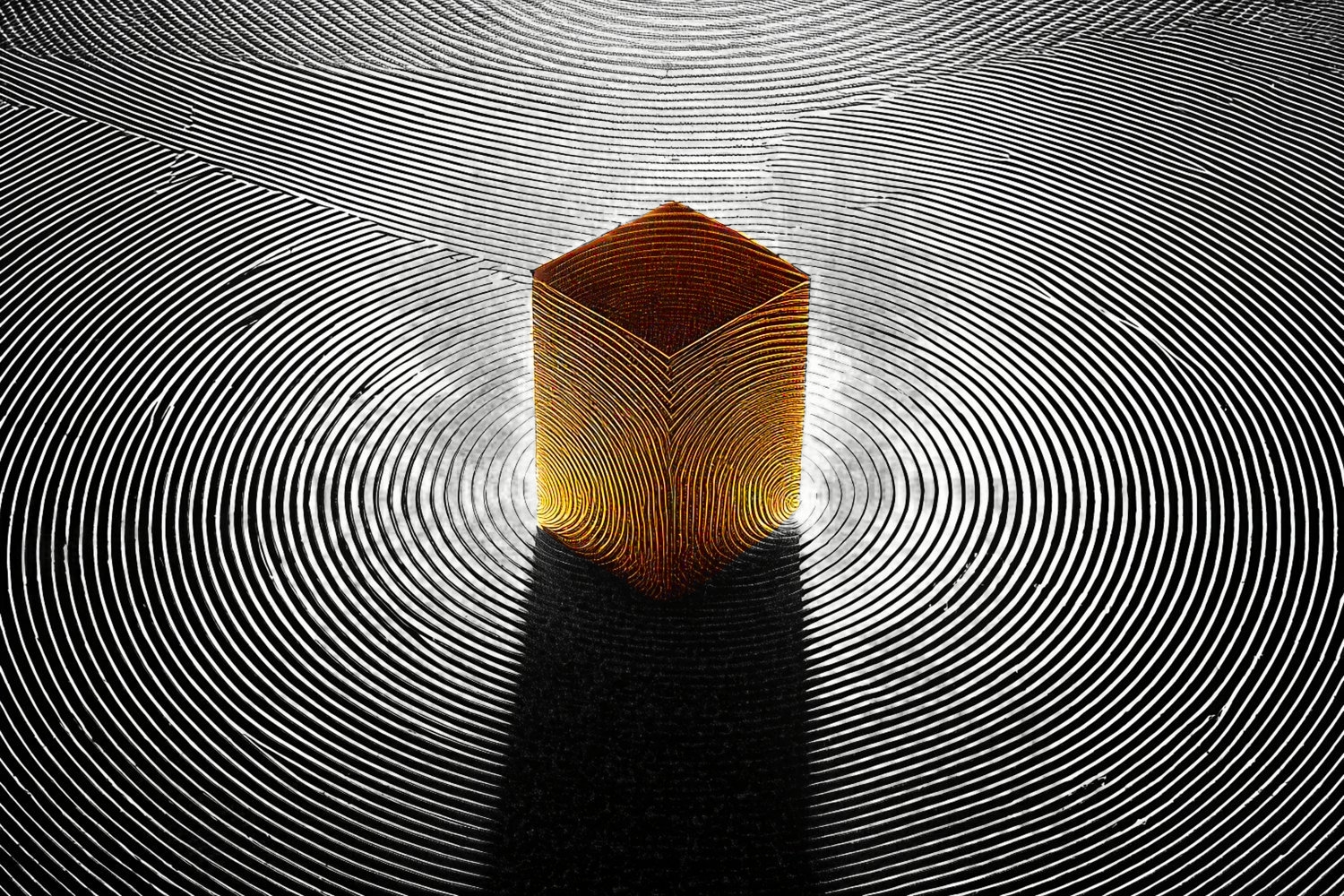
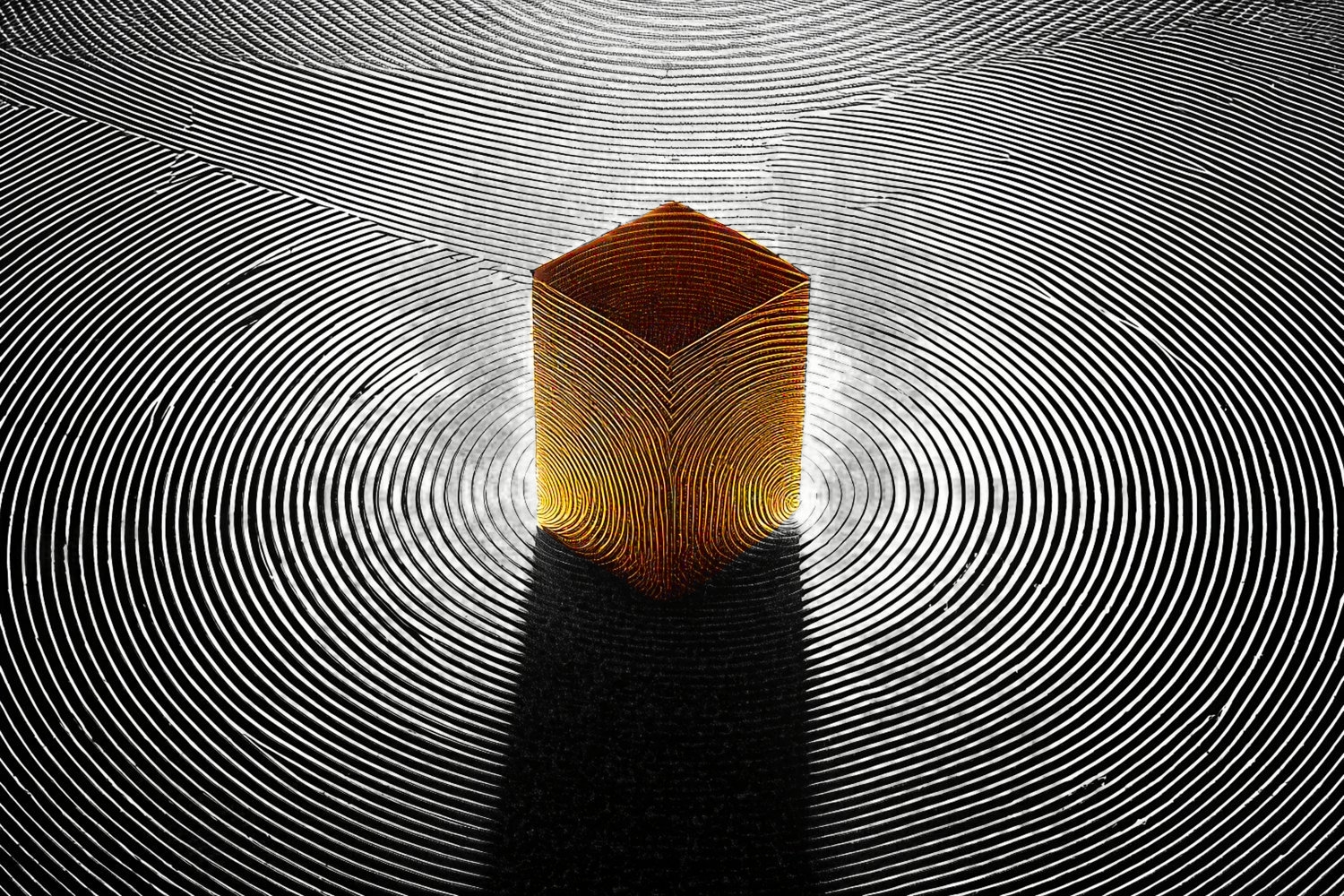
🎓 64/167
This post is a part of the Deep learning basics educational series from my free course. Please keep in mind that the correct sequence of posts is outlined on the course page, while it can be arbitrary in Research.
I'm also happy to announce that I've started working on standalone paid courses, so you could support my work and get cheap educational material. These courses will be of completely different quality, with more theoretical depth and niche focus, and will feature challenging projects, quizzes, exercises, video lectures and supplementary stuff. Stay tuned!
In the ever-evolving ecosystem of machine learning and deep learning frameworks, TensorFlow stands out as one of the most comprehensive and widely supported platforms for building, training, and deploying advanced neural networks. Originally developed by the Google Brain team and open-sourced in 2015 (Abadi and gang, OSDI 2016 introduced TensorFlow as a successor to DistBelief.), TensorFlow has continued to evolve, adding new features such as eager execution, distribution strategies for large-scale model training, and a high-level API called Keras that simplifies the process of model design and experimentation.
TensorFlow's core strength lies in its ability to handle large-scale computations across heterogeneous devices — CPUs, GPUs, TPUs, and specialized ASICs. Furthermore, it provides an extensive library of prebuilt operations, enabling researchers and practitioners to quickly construct and train sophisticated models spanning computer vision, natural language processing, recommender systems, and much more. Its flexible architecture also affords advanced users the freedom to experiment with custom operations, while still benefiting from TensorFlow's robust optimization, graph transformations, and hardware acceleration.
Alongside TensorFlow, there is Keras, an intuitive high-level deep learning API designed by François Chollet (Chollet and gang, 2015 introduced Keras initially as a standalone project, which later became tightly integrated with TensorFlow.). Keras provides an elegant and user-friendly interface for creating and training deep neural networks without requiring deep knowledge of TensorFlow's low-level details — yet it still offers enough flexibility for researchers to dive into intricate customizations.
Another essential aspect of the TensorFlow ecosystem is TensorBoard, a visualization toolkit that allows you to monitor metrics, inspect computational graphs, and debug issues in your training process. TensorBoard's logging and interactive dashboard features are integral for data scientists, machine learning engineers, and researchers who want a deeper understanding of how their model behaves over the course of training.
In this article, we will journey from foundational TensorFlow concepts (e.g., tensors, graphs, execution paradigms) to the Keras API (covering both Sequential and Functional approaches), and culminate in advanced topics, including custom layers, transfer learning, distributed training strategies, and the effective use of TensorBoard. We aim to give you enough theory and practical know-how to build and deploy your own production-grade neural networks.
By the end of this piece, you should:
- Understand the core abstractions (tensors, graphs, and sessions, especially in historical context) and how they fit into TensorFlow's execution model today.
- Grasp the difference between eager execution and graph execution, and know when each approach is most beneficial.
- Learn how to build, train, and evaluate TensorFlow/Keras models with real-world constraints in mind.
- Familiarize yourself with TensorBoard for monitoring experiment metrics, debugging, and presenting results.
- Get a sense of best practices and
Large-scale often means training on multi-GPU or multi-TPU setups, especially for compute-intensive tasks.large-scale training setups, including how to deploy TensorFlow models in production environments.
Feel free to install the latest version of TensorFlow right away and follow the sections with hands-on experimentation. As you progress, you will discover how to leverage the synergy between TensorFlow, Keras, and TensorBoard to build powerful applications in computer vision, NLP, and beyond.
Getting started with tensorflow
One of TensorFlow's key design principles is to offer a flexible interface that can be used at different levels of abstraction. At its foundation, TensorFlow provides a series of tools for numerical computation using dataflow graphs. However, with the release of TensorFlow 2.0, many default behaviors changed to make eager execution the standard, thus creating a more intuitive Python-like workflow. Before diving into advanced concepts, let us first discuss the basic building blocks.
Core concepts in tensorflow (tensors, sessions, graphs)
A tensor is the central data type in TensorFlow: it's a generalization of vectors and matrices to potentially higher dimensional spaces. Tensors can have any rank (the number of dimensions), and they can be manipulated via a range of operations, from simple arithmetic (e.g., addition and multiplication) to convolutional filters for image processing.
Traditionally, TensorFlow (particularly the 1.x versions) executed operations inside sessions that managed resources (e.g., GPU memory) and orchestrated the evaluation of computation graphs. A graph in TensorFlow is a set of nodes (operations) connected by edges (tensor data). Each node in the graph represents either a computational operation (like a matrix multiplication) or a variable holding data (like model parameters).
In older workflows, you would define a graph and then create a session to run parts of that graph. For example:
import tensorflow as tf
# Legacy example from TensorFlow 1.x
graph = tf.Graph()
with graph.as_default():
x = tf.constant([[1.0, 2.0], [3.0, 4.0]])
y = tf.constant([[5.0, 6.0], [7.0, 8.0]])
result = tf.matmul(x, y)
with tf.Session(graph=graph) as sess:
output = sess.run(result)
print(output)
However, with TensorFlow 2.x, you rarely see such patterns because of the shift to eager execution, which eliminates the need to explicitly create sessions in most cases. Eager execution performs operations immediately, returning concrete values instead of constructing a computation graph to be executed later. Nonetheless, it's valuable to understand these concepts to appreciate the historical context of TensorFlow and how it optimizes large-scale computations internally.
Eager execution vs. graph execution
In TensorFlow 2.x, eager execution is enabled by default. This imperative style of coding is much more "Pythonic," since each operation returns its result immediately — rather than building a disconnected graph representation. This makes debugging more straightforward, as you can inspect your variables and intermediate values without diving into the complexities of sessions.
Still, it's worth knowing that you can convert your eager code into an optimized graph for high-performance scenarios. tf.function is a powerful decorator that traces your Python function into a graph, enabling significant performance optimizations and portability (e.g., it can be saved as a SavedModel, reused in languages like C++ or Java, or deployed on mobile devices).
Consider the following example:
import tensorflow as tf
@tf.function
def multiply_matrices(a, b):
return tf.matmul(a, b)
x = tf.constant([[1.0, 2.0], [3.0, 4.0]])
y = tf.constant([[5.0, 6.0], [7.0, 8.0]])
# Eager execution call
res_eager = tf.matmul(x, y)
# Graph execution call
res_graph = multiply_matrices(x, y)
print("Eager result:", res_eager)
print("Graph result:", res_graph)
Here, res_eager is obtained by calling eagerly, while res_graph is the output of a traced function under the tf.function decorator, effectively turning it into a graph-mode operation behind the scenes.
Basic operations on tensors
TensorFlow offers a large library of mathematical operations that can be applied to tensors. These include:
- Arithmetic ops:
- Matrix ops:
- Reduction ops: reduce_sum, reduce_mean, reduce_max, dots
- Element-wise ops:
These operations can be chained together, building more complex transformations of your data. For instance, to compute the mean squared error (MSE) between two tensors and :
y_true = tf.constant([1.0, 2.0, 3.0])
y_pred = tf.constant([1.2, 1.9, 3.5])
mse = tf.reduce_mean(tf.square(y_pred - y_true))
print("MSE:", mse.numpy())
In this snippet, squares the element-wise difference between predictions and ground truth, and \text{tf.reduce_mean}(\dots) takes the average of all these squared differences.
Introduction to tensorflow datasets
A common challenge in machine learning is reading and preprocessing large datasets efficiently. The tf.data.Dataset API offers a high-level abstraction for building complex input pipelines, from reading data in parallel to applying transformations (e.g., batching, shuffling, and mapping) in an efficient manner.
For instance:
import tensorflow as tf
# Suppose we have some raw data in memory
raw_x = tf.range(10, dtype=tf.float32)
raw_y = tf.range(10, dtype=tf.float32) * 2.0
# Create a Dataset from tensor slices
dataset = tf.data.Dataset.from_tensor_slices((raw_x, raw_y))
dataset = dataset.shuffle(10).batch(2)
for batch_x, batch_y in dataset:
print("Batch X:", batch_x.numpy(), "Batch Y:", batch_y.numpy())
Using tf.data.Dataset, you can avoid bottlenecks in data loading, especially when dealing with enormous datasets stored on disk or distributed across multiple machines. You can also augment your data on the fly by mapping transformation functions, normalizing images, or applying textual tokenization for NLP tasks. This helps you keep your GPU or TPU fed with data, minimizing idle time and maximizing throughput.
Introducing keras
Keras is a high-level deep learning API that first arrived as an independent package but is now deeply integrated into TensorFlow. Its primary goal is to expedite experimentation by providing a concise, user-friendly interface for building neural networks. You can think of Keras as an abstraction layer that spares you from the details of manually creating graphs and implementing backpropagation.
Keras fosters rapid development, yet it also allows you to drop down into the underlying TensorFlow components if you need specialized custom operations or layers. This dual capability of high-level and low-level control makes Keras one of the most appealing frameworks for both newcomers and advanced researchers.
Sequential api vs. functional api
Keras offers multiple ways to define models:
- Sequential API: This is the simplest way to build a model, where you stack layers in a single, linear progression. This approach is often best for straightforward feed-forward networks or slightly more elaborate architectures that can be still thought of as a chain of layers.
import tensorflow as tf
from tensorflow.keras import layers
model = tf.keras.Sequential([
layers.Dense(64, activation='relu'),
layers.Dense(10, activation='softmax')
])
model.build(input_shape=(None, 100))
model.summary()
In the snippet above, we defined a Sequential model with two layers. The first layer has 64 hidden units with ReLU activation, and the second layer outputs 10 units with softmax activation.
- Functional API: This approach is far more flexible, enabling you to build models with multiple inputs, multiple outputs, and complex topologies such as residual connections and shared layers. Instead of simply stacking layers, you explicitly define how data flows from one layer to another by treating layers as callable objects on tensor inputs.
import tensorflow as tf
from tensorflow.keras import layers, Model
inputs = tf.keras.Input(shape=(100,))
x = layers.Dense(64, activation='relu')(inputs)
x = layers.Dense(32, activation='relu')(x)
outputs = layers.Dense(10, activation='softmax')(x)
model = Model(inputs=inputs, outputs=outputs)
model.summary()
Here, you can see we explicitly specify the input shape. Each layer is called on the output of the previous layer, and ultimately we define a Model object that encapsulates the entire network. This approach easily extends to more intricate architectures, like branching or merging layers, which are essential in many state-of-the-art models.
Building a simple neural network with keras
To demonstrate how straightforward building and training a neural network can be with Keras, let us create a small feed-forward network for a fictional regression task. Assume we have some numeric features and want to predict a single continuous target.
import numpy as np
import tensorflow as tf
from tensorflow.keras import layers, Model
# Generate some random data for demonstration
num_samples = 1000
num_features = 10
X_data = np.random.random((num_samples, num_features)).astype(np.float32)
y_data = np.random.random((num_samples, 1)).astype(np.float32)
# Define a simple feed-forward model using the Functional API
inputs = tf.keras.Input(shape=(num_features,))
x = layers.Dense(64, activation='relu')(inputs)
x = layers.Dense(32, activation='relu')(x)
outputs = layers.Dense(1, activation='linear')(x)
model = Model(inputs, outputs)
# Compile the model by specifying the loss, optimizer, and metrics
model.compile(loss='mean_squared_error',
optimizer=tf.keras.optimizers.Adam(learning_rate=0.001),
metrics=['mae'])
# Train (fit) the model
history = model.fit(X_data, y_data,
validation_split=0.2,
epochs=20,
batch_size=32)
Let us break down the key steps in this example:
- Data Preparation: We simulate random and for demonstration. In real use cases, you might load data from your local filesystem or from a tf.data.Dataset.
- Model Definition: We specify an input layer with shape and stack dense layers with ReLU activations. The final layer is a single neuron that outputs a continuous value (linear activation).
- Compilation: We define our optimization strategy (Adam in this case), along with a suitable loss function (MSE for regression). We also track an additional metric, MAE (mean absolute error), for better insight into training performance.
- Training: We call model.fit, which initiates the training loop for 20 epochs with a batch size of 32. The model is also validated on 20% of the data in each epoch.
Because Keras handles the heavy lifting in the background — like gradient computation, parameter updates, and device placement — this workflow remains concise and highly readable.
Using pretrained models in keras
Keras provides convenience functions to download and instantiate models that have been pre-trained on massive datasets, such as ImageNet. Common examples include VGG, ResNet, Inception, MobileNet, and others. These pretrained models often serve as feature extractors or starting points for transfer learning, allowing you to adapt them to specific tasks without training from scratch.
from tensorflow.keras.applications import VGG16
# Load a pretrained VGG16 model, excluding the top classification layer
pretrained_model = VGG16(weights='imagenet',
include_top=False,
input_shape=(224, 224, 3))
# Check the structure
pretrained_model.summary()
Here, we've loaded VGG16 with ImageNet weights and include_top=False so that the final classification layer is omitted. You can then add new layers on top to specialize the model for your particular classification or regression task.
Model training and evaluation
After constructing a Keras model, the next important steps are to choose an appropriate loss function, an optimization method, and an evaluation metric. In addition to that, we should carefully tune hyperparameters like the batch size, number of epochs, and the learning rate.
Defining an effective training loop involves:
- Choosing the right loss: Common examples:
- Mean Squared Error (), , for regression.
- Cross-Entropy () for classification tasks.
- Selecting an optimizer:
- SGD, Adam, RMSProp, Adagrad, among others.
- Each optimizer has adjustable hyperparameters (e.g., learning_rate, momentum, beta1, etc.).
- Metrics:
- For regression: MSE, MAE, MAPE.
- For classification: Accuracy, Precision, Recall, F1 (often computed outside the built-in metrics for multi-class).
- Batch size considerations:
- Smaller batch sizes provide more frequent gradient updates (more noise but can generalize better).
- Larger batch sizes can leverage vectorized operations on GPUs more efficiently, but might require carefully tuned learning rate schedules to match or exceed the generalization quality of small batches.
Model evaluation
During training, you can monitor your model's performance on a validation set to detect overfitting or underfitting. This typically involves tracking the chosen loss and metrics on both the training and validation partitions. In Keras, you specify the validation_data or validation_split parameter in model.fit.
If you want to evaluate the model on a separate test set after training:
test_loss, test_mae = model.evaluate(X_test, y_test)
print("Test Loss:", test_loss)
print("Test MAE:", test_mae)
When working on classification problems, you might want additional metrics like confusion matrices or ROC curves. These are usually computed outside of model.evaluate, by calling model.predict and then using scikit-learn's utility functions, for example.
Saving and loading models
Keras supports multiple formats for saving your trained models:
- HDF5 format:
# Save entire model to HDF5
model.save('my_model.h5')
# Load it back
from tensorflow.keras.models import load_model
loaded_model = load_model('my_model.h5')
- SavedModel format (recommended for TF2+ for deployment):
# Save to the TensorFlow SavedModel format
model.save('my_saved_model')
# Load it back
restored_model = tf.keras.models.load_model('my_saved_model')
The SavedModel format preserves the TensorFlow graph, variables, and any required assets for re-running the same computation. This is highly beneficial for serving models in production using TensorFlow Serving or for cross-platform inference (e.g., deploying on embedded or mobile devices with TensorFlow Lite).
Advanced features
Keras provides powerful constructs for building complex architectures and handling special use-cases. Below are some highlights that are particularly relevant in real-world contexts.
Custom layers and callbacks
Sometimes, prebuilt layers (Dense, Conv2D, LSTM, etc.) are not sufficient for specialized tasks. Keras allows you to create custom layers by subclassing tf.keras.layers.Layer:
import tensorflow as tf
class MyCustomLayer(tf.keras.layers.Layer):
def __init__(self, units=32, **kwargs):
super(MyCustomLayer, self).__init__(**kwargs)
self.units = units
def build(self, input_shape):
self.w = self.add_weight(shape=(input_shape[-1], self.units),
initializer='random_normal',
trainable=True)
self.b = self.add_weight(shape=(self.units,),
initializer='zeros',
trainable=True)
super(MyCustomLayer, self).build(input_shape)
def call(self, inputs):
return tf.matmul(inputs, self.w) + self.b
In this simple example, we define a custom layer that performs a linear transformation on its inputs. More advanced custom layers can do everything from specialized attention mechanisms to reparameterization tricks for variational autoencoders.
On the training side, callbacks are objects that allow you to intervene at specific stages of the training process — like after each batch or epoch. These are extremely powerful for tasks such as:
- Early stopping (stop training when validation loss doesn't improve).
- Learning rate scheduling.
- Logging metrics to TensorBoard.
- Saving checkpoints periodically.
Example usage:
from tensorflow.keras.callbacks import EarlyStopping, ModelCheckpoint
early_stop = EarlyStopping(monitor='val_loss', patience=3)
checkpoint = ModelCheckpoint(filepath='best_model.h5', save_best_only=True)
model.fit(X_data, y_data,
validation_split=0.2,
epochs=100,
callbacks=[early_stop, checkpoint])
Transfer learning and fine-tuning
Transfer learning leverages knowledge gained from a model trained on a large dataset for one task and applies it (or adapts it) to another related task. Typically, you:
- Load a pretrained model (e.g., ResNet50 trained on ImageNet).
- Freeze some of the earlier layers (so their weights don't get updated).
- Replace and train the final layer(s) for your custom classification or regression task.
Subsequently, you can fine-tune the entire model (or some portion of it) by unfreezing those earlier layers and training with a very low learning rate to avoid destroying the valuable pretrained weights. This is especially useful when your dataset is not large enough to train a deep network from scratch, but you still benefit from the deep representational power learned from a vast dataset like ImageNet.
Working with complex data (text, images, time series)
Keras also has specialized layers and utilities for dealing with more complex data modalities:
- Text data: Embedding layers, recurrent layers (LSTM, GRU), or even Transformers for sequence-to-sequence tasks.
- Image data: Convolutional layers, pooling layers, and advanced architectures for tasks like classification, detection, or segmentation.
- Time series or sequential data: RNNs, LSTMs, GRUs, and 1D convolutions.
You can combine these building blocks with preprocessing layers or external libraries to handle domain-specific transformations (e.g., text tokenization, image augmentation, time-series windowing, etc.).
Data augmentation and regularization techniques
A common technique to prevent overfitting (and to artificially enlarge your training set) is data augmentation. For image data, Keras provides ImageDataGenerator or the more modern tf.data transformations like tf.image.random_flip_left_right, tf.image.random_crop, and tf.image.random_brightness. You can build these into your training pipeline so that each image is randomly altered each epoch, effectively generating new samples on the fly.
Regularization in Keras can be done via:
- Weight decay / L2 regularization (e.g., kernel_regularizer in Dense or Conv2D layers).
- Dropout layers that randomly zero some activations during training (layers.Dropout).
- Batch Normalization (layers.BatchNormalization) to stabilize and accelerate training while reducing overfitting in many models.
Tensorboard
TensorBoard is a toolkit that provides the ability to visualize and understand your TensorFlow runs and graphs. Its main features include:
- Scalars: Track metrics like loss, accuracy, or custom scalars across epochs.
- Graphs: Visualize your model's computational graph, verifying the connections between layers or custom operations.
- Histograms: Investigate how your model's weights, biases, or activations evolve over time.
- Images: Visualize input samples, generated outputs (e.g., images produced by a GAN), or intermediate feature maps.
- Projector: View high-dimensional embeddings like word embeddings in a 2D/3D space for interpretability.
Logging metrics and visualizing training progress
To use TensorBoard effectively, you log training metrics through callbacks. A standard pattern is:
import tensorflow as tf
import datetime
# Define a directory where logs will be saved
log_dir = "logs/fit/" + datetime.datetime.now().strftime("%Y%m%d-%H%M%S")
tensorboard_callback = tf.keras.callbacks.TensorBoard(log_dir=log_dir, histogram_freq=1)
model.fit(X_data, y_data,
epochs=10,
validation_split=0.2,
callbacks=[tensorboard_callback])
Here, histogram_freq=1 ensures that histograms of weights and biases are logged for each epoch. After this, you can launch TensorBoard from the command line:
!tensorboard --logdir logs/fit
or from the appropriate environment if you are in a hosted platform like Google Colab or Jupyter Notebook (simply run %tensorboard --logdir logs/fit
). You'll be presented with an interactive dashboard showing your training curves and other logged information.
Inspecting model graphs and histograms
When you log graph information, TensorBoard can display the computational graph of your model. This is particularly handy for debugging complex architectures, ensuring the correct connections are formed between inputs, outputs, and intermediate layers. It also allows you to see any custom or compound operations in a more visual manner.
Histograms enable you to see how weights and activations are distributed. For instance, if you notice that your gradients are consistently saturating near zero, that's a strong clue you might need to adjust your initialization scheme, learning rate, or layer design. Similarly, if your weights blow up to extremely large values, you may be facing an exploding gradient problem.
Debugging with tensorboard
TensorBoard's visual analysis helps you catch subtle issues quickly. If the loss curve stops decreasing or becomes NaN, you can investigate the histograms or distributions of your variables. You might also track custom scalar metrics, such as the ratio of gradient norms to parameter norms, or the learning rate if you employ scheduling or warm restarts. The ability to deeply introspect your model's behavior often translates to faster iteration cycles and more stable experiments.
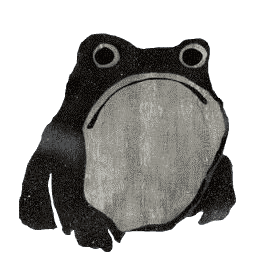
An image was requested, but the frog was found.
Alt: "TensorBoard main interface"
Caption: "A conceptual illustration of the TensorBoard UI, showing various logged metrics and charts."
Error type: missing path
Best practices and real-world applications
While TensorFlow, Keras, and TensorBoard provide powerful constructs and abstractions, actual industry scenarios introduce a variety of challenges — ranging from distributed training to memory constraints, from real-time model deployment to complex data pipelines.
Scaling up training with distributed tensorflow
In large-scale use cases, you may train models on multiple GPUs, multiple machines, or even specialized hardware like Google's TPUs. TensorFlow's tf.distribute module offers strategies to simplify this process:
- MirroredStrategy: For single-machine multi-GPU training.
- MultiWorkerMirroredStrategy: For synchronous training on multiple machines.
- TPUStrategy: For leveraging TPU pods.
Example usage:
strategy = tf.distribute.MirroredStrategy()
with strategy.scope():
model = build_my_model()
model.compile(optimizer='adam', loss='mse')
model.fit(dataset, epochs=10)
The distribution strategy manages how data is split across replicas and how gradients are aggregated to update the model. This dramatically simplifies the code needed to scale computations, preserving a near-identical workflow for single-GPU vs. multi-GPU training scenarios.
Deploying tensorflow models (tensorflow serving, tf lite)
Once you have a trained model, the next step is typically deployment. TensorFlow supports multiple deployment avenues:
- TensorFlow Serving: A high-performance, flexible serving system for machine learning models, designed for production environments. After exporting your model to the SavedModel format, you can serve predictions via a REST or gRPC interface.
- TensorFlow Lite: A lightweight solution for deploying models on mobile and embedded devices. It performs optimizations like quantization and pruning to reduce model size and inference latency.
- TF.js: If you need inference (or even training) directly in the browser or a Node.js environment, TensorFlow.js is an option.
Integrating tensorboard into production workflows
In production or large-scale research setups, you might run many experiments in parallel. Each experiment can write logs to a separate folder, or you can organize runs by hyperparameters (learning rate, batch size, architecture variant, etc.). TensorBoard can aggregate these logs, allowing you to compare different runs visually.
Performance optimization tips
- Use vectorized operations: Write your code in a way that leverages TensorFlow's built-in ops and layers for parallel computation across the entire batch. Avoid Python loops when possible.
- Leverage XLA compilation: TensorFlow's XLA (Accelerated Linear Algebra) can compile parts of your graph into efficient, device-specific code. You can activate it by using the jit_compile=True parameter inside tf.function.
- Monitor GPU usage: Tools like nvtop (Linux) or nvidia-smi can help you see if your GPU is underutilized. If so, data loading or preprocessing might be a bottleneck. Make sure you use tf.data pipelines effectively.
- Mixed precision training: In modern GPUs (e.g., NVIDIA Turing or Ampere architecture), using float16 or bfloat16 can significantly speed up training and reduce memory usage. TensorFlow provides tf.keras.mixed_precision for this.
Handling memory constraints in large-scale models
When models become large (e.g., multi-hundred million parameters or more), you must carefully manage memory:
- Reduce batch sizes or use gradient checkpointing to save memory.
- Use mixed_precision to store some layers' weights in float16.
- Employ distributed training to spread the model across multiple devices or machines.
- Use memory profiling tools such as tf.profiler to pinpoint which layers consume the most resources.
Model Deployment Scenarios
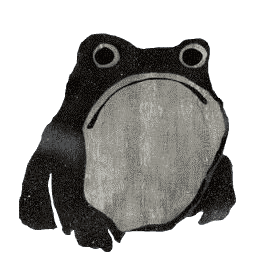
An image was requested, but the frog was found.
Alt: "High-level TensorFlow pipeline"
Caption: "Illustration of a typical pipeline from data ingestion to training and serving a TensorFlow model in production."
Error type: missing path
With all these considerations in mind, it is evident that TensorFlow, Keras, and TensorBoard constitute a comprehensive ecosystem for end-to-end deep learning projects. From simple prototypes to industrial-scale deployments, they provide the flexibility, performance, and community support needed to tackle a broad spectrum of machine learning problems.
Whether you aim to create a quick prototype on your personal GPU, fine-tune a state-of-the-art NLP model, or train a massive image recognition system on a GPU cluster, the synergy between TensorFlow, Keras, and TensorBoard offers a clear and scalable path forward. Indeed, many top-tier research papers, conference submissions, and industry solutions are built upon these tools, leveraging their modular design and wide hardware support.
As you keep exploring, be sure to consult official and community-driven resources. The TensorFlow documentation, Keras code examples, and TensorBoard tutorials are continuously updated to reflect best practices and the latest features. You may also look into the research track: for instance, reading about the evolution of frameworks in ML conferences (NeurIPS, ICML, JMLR, etc.) can reveal how engineers and scientists push TensorFlow's boundaries for advanced tasks like multi-modal learning, reinforcement learning, or massive language model training.
I encourage you to experiment boldly, visualize your results meticulously, and remain curious about the underlying mechanics that TensorFlow so elegantly abstracts. By doing so, you'll grow as both a practitioner and a researcher, tapping into one of the most capable machine learning frameworks in existence.