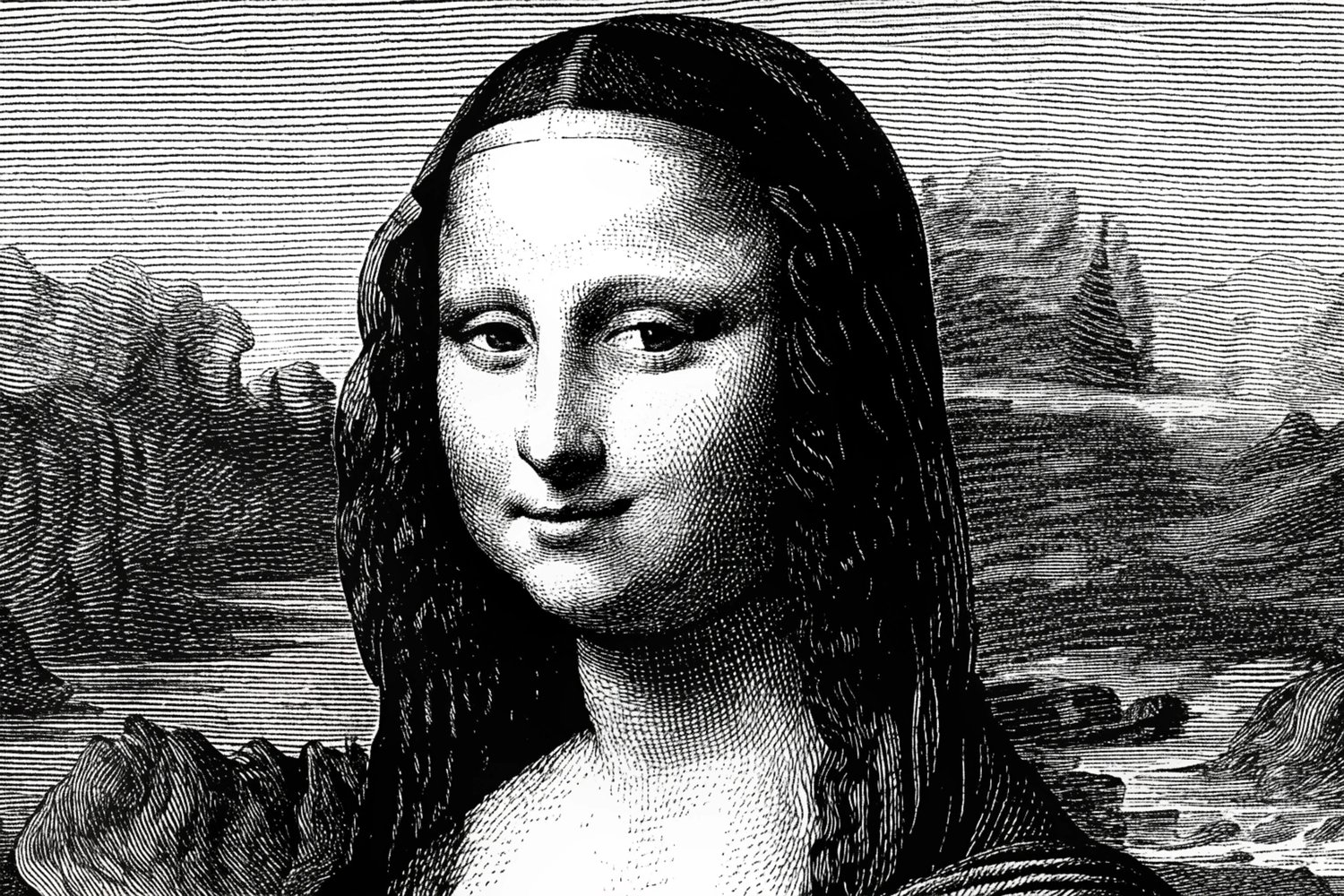
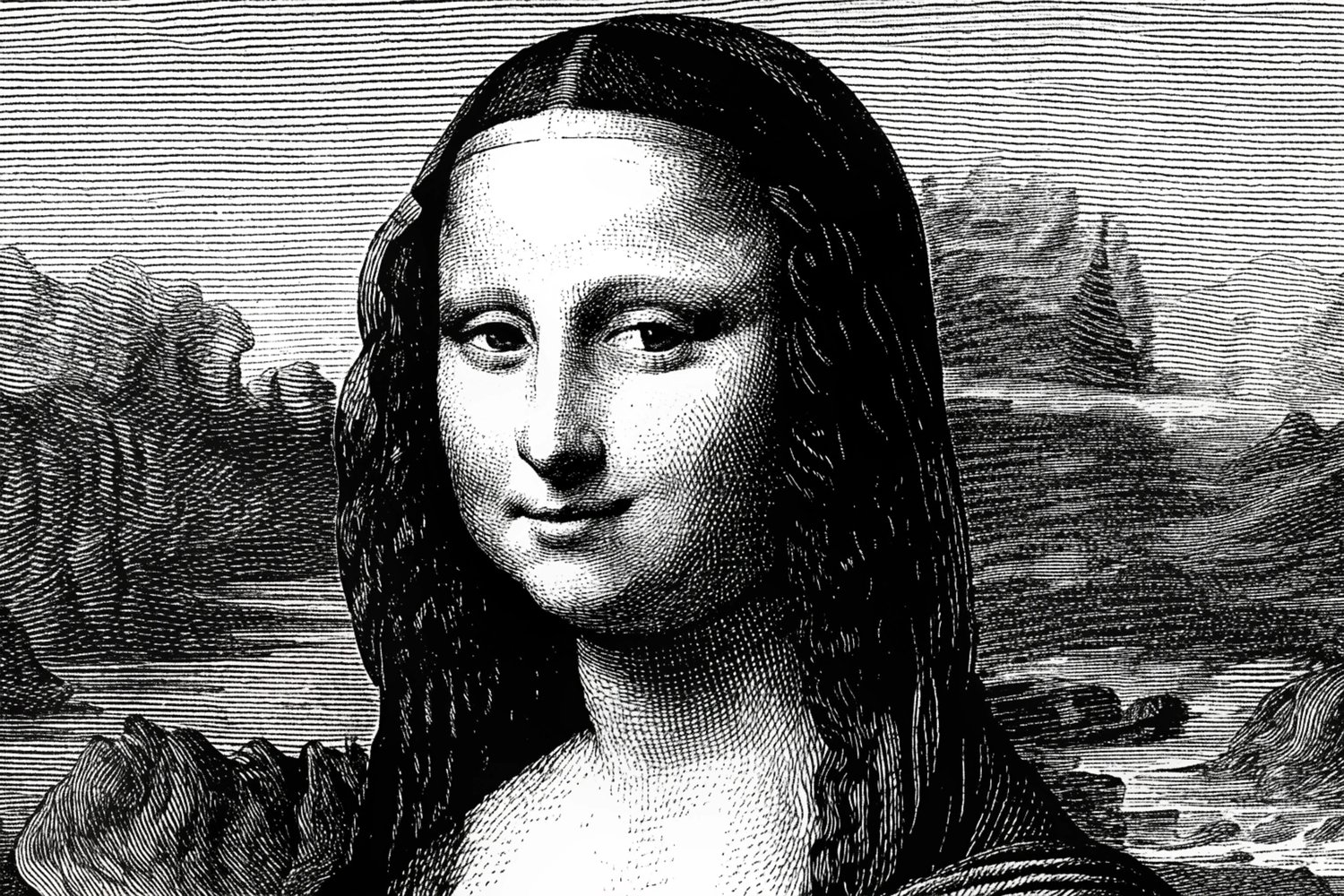
🎓 104/167
This post is a part of the Computer vision educational series from my free course. Please keep in mind that the correct sequence of posts is outlined on the course page, while it can be arbitrary in Research.
I'm also happy to announce that I've started working on standalone paid courses, so you could support my work and get cheap educational material. These courses will be of completely different quality, with more theoretical depth and niche focus, and will feature challenging projects, quizzes, exercises, video lectures and supplementary stuff. Stay tuned!
Image blending refers to the process of taking two or more images and merging them into a single composite image in such a way that the boundaries between the images become imperceptible or nearly invisible. In typical scenarios, you might wish to seamlessly insert a region from one image (for example, a foreground object) into another (for example, a background scene), and have the final composite look natural, consistent, and visually pleasing.
Historically, this problem has been widely addressed in fields like computer vision, computational photography, image editing, and graphic design. Simple solutions — such as cutting out a region from one photo and pasting it onto another — often produce harsh edges or unrealistic color/illumination differences around the seam. More advanced solutions use blending techniques that aim to preserve the integrity of boundaries, gradients, colors, and textures, thereby creating results that look coherent and smooth.
In this article, I'll dive into both classical and modern approaches to image blending, considering the fundamental definitions, mathematical derivations, and practical code examples in Python. Topics will include alpha blending, gradient domain blending, Poisson blending, Laplacian pyramid blending, and more. I'll also go into advanced deep learning–based methods, referencing the latest research on generative networks, painterly harmonization, and style transfer, illustrating how neural networks can be leveraged for blending tasks. Finally, I'll address best practices for evaluating blended images, discuss design trade-offs, show how to integrate these techniques in a production environment, and touch upon specialized cases like HDR blending, multimodal (e.g., infrared vs. visible) blending, 3D blending, and domain-specific tasks.
This article is intended for readers with a fairly strong background in image processing and machine learning. I assume you already know about the fundamentals of convolution, basic matrix operations, and are comfortable with at least one deep learning framework (e.g., PyTorch or TensorFlow). Nonetheless, I will provide enough background so that the advanced concepts remain accessible. Although I will not shy away from in-depth theoretical discussions, I will keep the overall style of the article approachable, prioritizing clarity and intuition.
The text is organized as follows:
- introduction
- fundamentals
- basic concepts and terminology
- mathematical background
- common operations (addition, averaging, masking, etc.)
- classical approaches
- alpha blending
- gradient domain blending
- poisson blending
- laplacian pyramid blending
- deep learning techniques
- convolutional neural networks
- generative adversarial networks
- autoencoders for image fusion
- implementation
- opencv & pytorch code examples step-by-step
- evaluation metrics
- objective image quality measures (psnr, ssim)
- subjective evaluation
- trade-offs between efficiency and accuracy
- practical considerations
- preprocessing steps (scaling, normalization)
- hardware and computational requirements
- integrating blending techniques in production pipelines
- advanced topics
- multimodal image blending (e.g., infrared and visible)
- high dynamic range (hdr) blending
- 3d image blending (stereo, volumetric data)
- domain-specific adaptations (medical imaging, remote sensing)
Where relevant, I will supplement the explanations with references to leading research (from conferences like NeurIPS, ICCV, CVPR, etc.) that have moved the state of the art forward, especially in gradient-based or neural network–based blending. I will also show code snippets in Python for demonstration.
It's worth noting that, in many real-world image editing scenarios, you might combine different blending techniques or adapt a single approach to better handle the idiosyncrasies of your data. A thorough understanding of the material in this article will let you pick and choose the right solution for your own tasks — whether you're designing a new augmented reality pipeline, building advanced photo-editing software, or generating synthetic images for data augmentation in machine learning workflows.
fundamentals
basic concepts and terminology
When we talk about "image blending", we're concerned with combining two images — often referred to as a "foreground" (the object or region to be inserted) and a "background" (the underlying scene). The region of interest in the foreground is usually specified by a mask that indicates which pixels of the foreground image should be retained. This mask is typically a binary or alpha mask, but more advanced approaches use soft transitions or partial transparency.
Commonly used terms:
- Foreground image (source): The image containing the visual element or object we want to place into another image.
- Background image (target): The image onto which the source's region will be blended.
- Mask: Often denoted by , a binary or grayscale matrix the same size as the images, indicating which pixels belong to the foreground region. If for pixel , that pixel belongs to the region that should be included in the composite; if then that pixel belongs to the background.
- Seam: The boundary region where the new content is inserted. This is typically where color or illumination mismatches need to be handled carefully.
- Feathering: A simple form of image blending that softly transitions from foreground to background in the overlapping region.
The user's unstructured text snippet (in another language) mentioned that simple "copy and paste" can yield undesirable artifacts, making the result look like a crude collage. This is because the difference in brightness, color distribution, or texture can be too conspicuous. A more sophisticated approach called blending aims to produce a seamless transition by adjusting pixel intensities appropriately at the boundary and ensuring consistent illumination and gradient information.
mathematical background
In many classical blending approaches, the notion of pixel intensities and their local gradients is paramount. If is the intensity distribution of the source image in the masked region, and is the intensity distribution of the background image, the general goal is to produce a composite that looks smooth both in the masked region and at its boundary, while preserving the essential structure and content of .
One frequent approach is to consider gradient constraints. If you define the gradient of the composite inside the masked region to be close to that of the source image — and enforce boundary conditions from the background image — then you can minimize an objective related to gradient differences. This leads to solving a partial differential equation, often the Poisson equation, , with boundary conditions given by the background. The discrete version typically yields a large linear system that can be solved by iterative methods (e.g., Gauss-Seidel or multigrid approaches).
common operations (addition, averaging, masking, etc.)
Some of the simpler blending operations revolve around direct pixel-wise arithmetic. In the simplest scenario, you might do:
which simply pastes the source on top of the background where the mask is 1. Such an approach is also known as Copy and paste or alpha compositing (when the mask is a floating-point alpha channel).
Other low-level image manipulations often involved in blending include:
- Image addition:
- Weighted averaging:
- Feathering along boundaries: Soft transitions that reduce boundary artifacts.
These straightforward approaches, however, have their limitations. They typically do not address differences in illumination or color distribution and won't remove strong seams or preserve the high-frequency details in the appropriate place. That's where more sophisticated methods like gradient domain blending or Laplacian pyramids come in.
classical approaches
Classical approaches to image blending have been studied extensively for decades. They rely on transformations in the pixel or gradient domain to produce more natural results than naive cut-and-paste.
alpha blending
Alpha blending is often considered the most basic method for compositing images, especially in computer graphics. You have an alpha mask (which may have floating-point values between 0 and 1), and you blend images as:
where is the opacity at pixel . In many contexts, is simply 1 or 0, but for smoother transitions, you can define partial opacities near the boundary of the region. This is a quick method that doesn't require solving large optimization problems; however, it doesn't handle significant color differences or lighting mismatches.
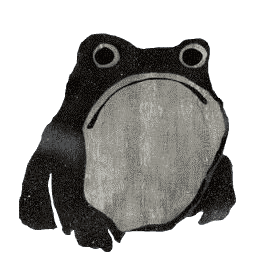
An image was requested, but the frog was found.
Alt: "alpha blending of a foreground object on background"
Caption: "Simple alpha blending example: partial transparency is used to mix the source and target images."
Error type: missing path
gradient domain blending
Gradient domain blending addresses the problem of mismatched intensity or color distribution in a more principled way. The key idea is to preserve the gradient (i.e., local differences in intensity) of the foreground region while enforcing smooth boundary conditions at the edges. In the 2D continuous case, this boils down to solving a Poisson equation:
where is the unknown composite image in the masked region , is the boundary of that region, is the source image, and is the background image. The boundary condition ensures that at the edge of the insertion, the composite exactly matches the background.
In discrete form, each pixel inside the mask has a constraint that tries to keep its discrete gradient consistent with that of the source. The boundary of the mask is constrained to match the background pixels. By solving a set of linear equations (one for each pixel in the masked region), you obtain a smoothly integrated composite. This approach drastically reduces visible seams, as it effectively "borrows" the low-frequency color context from the background while keeping the high-frequency details from the inserted region intact (or at least consistent with the source's gradients).
poisson blending
Poisson blending, a specific type of gradient domain blending, was famously introduced in the paper "Poisson Image Editing" (Patrick Perez and gang, 2003). The method focuses on transferring the gradient from the source region to the target region while respecting boundary conditions. The discrete Poisson equation in a typical 4-neighborhood grid is:
Here, is the composite, is the inserted image, and is the background. The Laplacian operator in discrete form is something like:
where is the neighborhood of pixel (usually 4 or 8 connectivity). When you set up all these equations for all pixels in and incorporate boundary constraints from , you can solve for . The result is a composite that adjusts the brightness and color in a smooth fashion, removing harsh transitions.
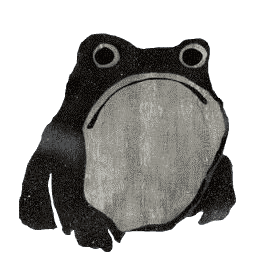
An image was requested, but the frog was found.
Alt: "Illustration of Poisson image editing for seamless merging"
Caption: "Poisson blending drastically reduces brightness discontinuities at the boundary of the inserted region."
Error type: missing path
laplacian pyramid blending
Laplacian pyramid blending is another classical and elegant technique, introduced in the mid-1980s (Burt and Adelson). It constructs a multi-scale (pyramidal) representation of both the source and target images, blends them at each scale, and then reconstructs the final image by collapsing the pyramid. The main advantage of this approach is that it can handle large-scale intensity differences as well as fine-scale details.
- Construct Gaussian pyramids for both source and target images.
- Generate Laplacian pyramids by subtracting adjacent levels in the Gaussian pyramid.
- Blend each level of the Laplacian pyramid using a smooth mask or weighting function.
- Reconstruct the final blended image by adding up the Laplacian levels from bottom to top.
By performing blending in the frequency (or scale) domain, Laplacian pyramid blending effectively merges low-frequency color information in a coarse manner while preserving high-frequency details in a more localized fashion. It's computationally quite efficient for many use cases and remains a popular choice in advanced image editors.
deep learning techniques
Over the past decade, deep learning methods have revolutionized many areas of computer vision, including image blending. Neural network–based strategies can be more flexible, capable of learning context-specific blending rules. Below, I'll overview a few of the most prominent frameworks you might encounter.
convolutional neural networks
Basic CNNs are a class of deep neural networks widely used in analyzing visual imagery (convolutional neural networks) can be trained end-to-end to perform tasks related to image blending. For instance, suppose you want to create a CNN that takes in two images (foreground and background) plus a mask and outputs a seamlessly blended result. You could train such a CNN using a large dataset of image pairs with known ground-truth composites. The network might learn local patterns that help it preserve boundaries, correct color mismatches, and produce realistic transitions. However, collecting a large labeled dataset for such a purpose can be challenging. In practice, many approaches rely on synthetic data or self-supervised strategies.
generative adversarial networks
Generative adversarial networks (GANs) [Goodfellow and gang, 2014] have also found use in blending or "harmonization" tasks. A notable sub-problem, called Image Harmonization means adjusting the color and illumination of a pasted region to match the target backgroundimage harmonization, attempts to adapt the color style and illumination of the inserted region in order to make it consistent with the background. This can be cast as a conditional image-to-image translation problem: given a masked composite, produce an output that is more consistent. A GAN, with a generator (G) and a discriminator (D) network, can be trained on pairs of composites and real images to encourage the generator to produce blended images that look indistinguishable from real scenes.
Below is a generic architecture idea:
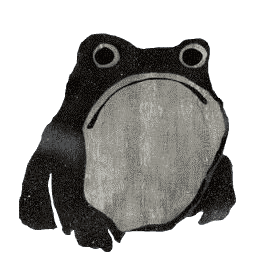
An image was requested, but the frog was found.
Alt: "GAN-based blending architecture"
Caption: "A generator network refines or blends the composited image, while a discriminator tries to distinguish real images from the blended results."
Error type: missing path
With the right loss functions — often a combination of adversarial loss, pixel reconstruction loss, perceptual loss (based on pretrained CNN feature matching), and sometimes gradient-based constraints — GAN-based methods can produce high-quality blended images that handle complicated lighting and style differences. One line of research (notably in image-to-image translation) might also incorporate semantic or instance-level constraints to better align the content in the source region with context in the target.
autoencoders for image fusion
Autoencoders are another deep architecture that can be applied in blending or fusion tasks. A typical scenario is merging multiple sensor modalities (e.g., infrared and visible images) into a single representation, or combining multiple exposures for HDR. An autoencoder can learn a shared latent space that captures the crucial features from each modality. Then, the decoding step can combine those features to produce a fused output.
In a more direct image blending scenario, you could feed two images into a multi-branch encoder that merges at some level, and then decode a single output that attempts to seamlessly integrate them. Loss terms might include pixel-wise reconstruction, gradient alignment, plus more advanced constraints to ensure that the final image has consistent color distribution.
implementation
Below, I'll give examples in both OpenCV and PyTorch. The examples focus on classical Poisson blending and a simple neural approach. In practice, you can adapt these snippets or combine them into more advanced pipelines.
opencv (classical poisson blending)
OpenCV doesn't include built-in Poisson blending in the older versions, but some implementations exist in the community. Let's do a minimal working example. Suppose we have:
- `foreground.png` (the image containing the object to be inserted)
- `background.png` (the target)
- `mask.png` (a binary mask highlighting the object)
You can solve the Poisson equation in Python using discrete solvers or rely on a library that implements it. Here's a conceptual code snippet:
import cv2
import numpy as np
def poisson_blend(foreground, background, mask, offset=(0,0)):
"""
Very simplified demonstration of Poisson blending.
foreground, background, mask are all NumPy arrays (BGR).
offset indicates where to place the foreground in the background.
"""
# We rely on the "seamlessClone" function from OpenCV as a proxy to Poisson blending.
# "normal_clone" is the classical approach which tries to do gradient domain blending.
center = (offset[0] + foreground.shape[1]//2, offset[1] + foreground.shape[0]//2)
# There is a built-in function in cv2: seamlessClone. It's basically Poisson-based blending
# behind the scenes.
output = cv2.seamlessClone(
foreground,
background,
mask,
center,
cv2.NORMAL_CLONE
)
return output
if __name__ == '__main__':
fg = cv2.imread("foreground.png", cv2.IMREAD_COLOR)
bg = cv2.imread("background.png", cv2.IMREAD_COLOR)
mk = cv2.imread("mask.png", cv2.IMREAD_GRAYSCALE)
# Convert mask to binary if needed
_, mk_bin = cv2.threshold(mk, 128, 255, cv2.THRESH_BINARY)
result = poisson_blend(fg, bg, mk_bin, offset=(50, 100))
cv2.imwrite("blended.png", result)
In this snippet:
cv2.seamlessClone
is an OpenCV function that implements a form of Poisson image editing.- I pass the
NORMAL_CLONE
flag, which does a standard Poisson blend. (There are also flags likeMIXED_CLONE
that handle mixed gradients, etc.). - The
center
is where the foreground is placed. The seamlessClone function internally solves the blending problem in the gradient domain, producing a composite image that should have minimal boundary artifacts.
pytorch (simplified neural approach)
A naive example of a learned blending approach in PyTorch might be as follows. This is more of a demonstration of how you'd set up a trainable model for blending:
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision import transforms
class SimpleBlender(nn.Module):
def __init__(self):
super(SimpleBlender, self).__init__()
# Very naive model: a small CNN to produce an output from the input images
self.conv1 = nn.Conv2d(6, 64, kernel_size=3, padding=1) # 3 channels from fg + 3 from bg
self.conv2 = nn.Conv2d(64, 32, kernel_size=3, padding=1)
self.conv3 = nn.Conv2d(32, 3, kernel_size=3, padding=1)
self.relu = nn.ReLU(inplace=True)
def forward(self, fg, bg, mask):
# Concatenate input along channel dimension: (fg, bg)
# mask might be used as well, but let's keep it simple
x = torch.cat([fg, bg], dim=1) # shape: B x 6 x H x W
x = self.relu(self.conv1(x))
x = self.relu(self.conv2(x))
out = torch.sigmoid(self.conv3(x)) # output in [0,1]
return out
if __name__ == '__main__':
# Example usage:
# Suppose we have a dataset of (foreground, background, ground_truth_blend),
# and we want to train the network to minimize a simple L2 or perceptual loss.
# We'll skip dataset loading for brevity and do just a random test.
net = SimpleBlender()
net.train()
optimizer = optim.Adam(net.parameters(), lr=1e-4)
criterion = nn.MSELoss()
# Dummy data
fg_sample = torch.rand(1, 3, 256, 256) # batch of 1, 3ch, 256x256
bg_sample = torch.rand(1, 3, 256, 256)
# For training, you also need the ground truth blended sample, ideally
gt_blended = torch.rand(1, 3, 256, 256)
mask_sample = torch.ones(1, 1, 256, 256)
# forward pass
output = net(fg_sample, bg_sample, mask_sample)
# compute loss
loss = criterion(output, gt_blended)
loss.backward()
optimizer.step()
print("Training step done. Loss =", loss.item())
Of course, a real neural blending system would require a carefully prepared dataset, possibly including random augmentations for the placement of objects, multiple loss terms (e.g., adversarial, perceptual, mask-based constraints), and more.
evaluation metrics
objective image quality measures (psnr, ssim)
When you evaluate your blending results, there are several objective metrics:
-
Peak Signal-to-Noise Ratio (PSNR):
where (\mathrm{MAX}) is the maximum possible pixel value (e.g., 255 for 8-bit images) and (\mathrm{MSE}) is the mean squared error between the blended image and a ground-truth reference. PSNR is more commonly used in tasks where you have a known reference, like compression or denoising. In blending, you often don't have a perfect ground-truth composite.
-
Structural Similarity Index Measure (SSIM):
SSIM tries to mimic the human perception of structural similarity. If you do have a ground-truth target for how the composite should look, SSIM can be a more robust measure than pure MSE-based metrics.
While objective metrics can be useful for tuning parameters and comparing methods in a controlled setting, there's often no single definitive ground truth for how a composite should look. Hence, purely objective metrics might fail to reflect the subjective qualities of a good blend.
subjective evaluation
Because image blending is partly about aesthetics and visual plausibility, subjective tests remain crucial. For instance, you might conduct a user study, showing participants various blended images and asking them to choose the most realistic one. You could also measure reaction times or use a rating scale. In research contexts, papers often include a small user study or a preference test to demonstrate that their blending approach yields better perceived quality.
trade-offs between efficiency and accuracy
- Computational complexity: Approaches like Laplacian pyramid blending can be done relatively fast, while Poisson blending requires solving large linear systems (though it's still quite feasible for moderate image sizes). Some neural approaches can be extremely slow if they involve large networks and iterative optimization procedures (e.g., style transfer methods).
- Memory footprint: High-resolution images combined with multi-scale or deep neural networks can demand large memory resources, especially if you're doing gradient-based optimization.
- Implementation complexity: Classical methods might be easier to implement (some are even one-liners in OpenCV), but deep learning approaches can require large training sets, HPC resources, and intricate hyperparameter tuning.
You must weigh these trade-offs based on the project constraints. For real-time applications — like augmented reality on mobile devices — lighter or more approximate methods might be favored. For offline editing or high-end cinematic production, you can afford heavier computations for higher-quality blends.
practical considerations
preprocessing steps (scaling, normalization)
Before blending, you typically:
- Align or register the source and target images so that the region to be inserted is in the correct location. Otherwise, the object might not match the perspective or geometry of the background.
- Resize images to a manageable resolution. If the images are extremely large (e.g., thousands of pixels on each side), you might start with a smaller resolution for faster computations, then refine at full resolution if needed.
- Color or intensity normalization: If the dynamic ranges of the two images differ dramatically, you might want to do a global color matching or histogram matching to reduce the mismatch prior to the main blending.
hardware and computational requirements
- Many classical blending methods run comfortably on CPU for typical image sizes (like 512×512, 1024×1024, etc.). However, high-resolution images can push these solutions to their limits, especially with iterative solvers.
- Neural-based approaches often benefit from GPU acceleration. If you are training a model for blending, you'll likely want at least one GPU with enough VRAM. If you are using a style-transfer-like approach, you might iterate for hundreds or thousands of gradient steps, and GPU parallelism is critical.
integrating blending techniques in production pipelines
- Automation: If you're building a pipeline that automatically composites objects into scenes (for example, in e-commerce product placement or AI-based photo editing tools), you'll likely design a system that can do all the steps: object segmentation, perspective correction, color matching, and final blending.
- Interactivity: In some products (like a user-facing photo editor), you might want a near-real-time method so that a user can drag an object around and see a blended result instantly. Such a system might rely on approximate but fast blending (like Laplacian pyramid or a pretrained real-time CNN) rather than an expensive iterative solver.
- Version control and reproducibility: As images are changed and re-processed, especially in large-scale data pipelines, ensuring you keep track of the parameters, models, and code versions used to produce each composite is essential. This is more of an engineering best practice than a purely algorithmic concern.
advanced topics
multimodal image blending (infrared and visible, etc.)
In some cases, you need to blend images from different sensors. For instance, you might want to blend an infrared image with a visible-light image to highlight objects that are only visible in IR while retaining the scene structure from the visible domain. Traditional approaches might combine color channels with IR intensity in a naive manner, but more advanced methods rely on:
- Saliency-based weighting: where the method automatically emphasizes the most informative parts of each modality.
- Deep autoencoder approaches: that learn a shared representation and then decode into a fused output.
- Wavelet or Laplacian pyramid techniques: specifically adapted for multisensor data.
high dynamic range (hdr) blending
Blending multiple exposures of the same scene is a classic approach to create an HDR image. You typically want to combine details from the underexposed and overexposed images to form a single image that captures detail in both shadows and highlights. Tools like exposure fusion, which is related to Laplacian pyramid blending, are widely used:
- You take bracketed exposures.
- Each exposure is assigned a weight map depending on local contrast, saturation, or well-exposedness.
- Weighted blending is performed in a multi-scale fashion (e.g., Gaussian or Laplacian pyramids).
The result is an HDR-like image without explicitly reconstructing a radiance map. This is sometimes referred to as multi-exposure fusion or multi-bracket fusion.
3d image blending (stereo, volumetric data)
Blending in 3D scenarios becomes more complicated because you must maintain geometric consistency. For instance, in stereo or multi-view systems, you might want to replace part of a 3D volume or 3D model with data from another. This can require approaches such as:
- Depth-based alignment: ensuring that the replaced region has the correct geometry.
- Volumetric Poisson blending: which generalizes the notion of 2D gradient domain blending to 3D.
Medical imaging (e.g., MRI or CT) might also require volumetric blending if combining multiple scans or if reconstructing a 3D volume from partial data. The same broad ideas of preserving gradient consistency can be extended to 3D domains, but the computational overhead is higher.
domain-specific adaptations (medical imaging, remote sensing)
- Medical imaging: You might want to combine MRI sequences or fuse MRI and CT data to highlight certain tissues or pathologies. The success of blending can have significant diagnostic implications, so any approach must be validated carefully.
- Remote sensing: Combining data from different satellites (e.g., multispectral, hyperspectral, radar) for a single region can yield more informative composite images. Special care might be taken to preserve the interpretability of each band while removing artifacts.
extra in-depth discussions (referencing unstructured text data)
Below, I'll integrate and elaborate on some extended topics mentioned in the unstructured text snippet, which included Poisson blending, deep painterly harmonization, and advanced style-based blending. These expansions offer insight into more specialized or state-of-the-art neural approaches.
- Poisson blending – already discussed, but note the text described the discrete formulation in detail, with an iterative solution for the system of equations.
- Neural style transfer – techniques that leverage CNN-based feature representations, e.g., Gatys and gang (2016).
- Deep painterly harmonization – a two-stage process introduced by Luan and gang (2018), combining gradient domain approaches with style losses to seamlessly adapt inserted content to a painting's style.
An especially interesting example is when you want to insert a real photograph object into a painting, and you need to adapt not only color but also texture at a brushstroke level. The deep painterly harmonization approach does exactly that: it first does a coarse alignment in the gradient domain, then refines via neural style transfer methods, ensuring the final piece looks like it was created by the original artist's strokes.
For completeness, the article references:
- Patrick Perez, Michel Gangnet, Andrew Blake (2003). Poisson Image Editing.
- Leon A. Gatys, Alexander S. Ecker, Matthias Bethge (2016). Image Style Transfer Using Convolutional Neural Networks.
- Fujun Luan, Sylvain Paris, Eli Shechtman, Kavita Bala (2018). Deep Painterly Harmonization.
These references show how the classical mathematics of Poisson blending merges with modern deep learning feature extraction to create new possibilities in image editing pipelines.
conclusion or final remarks
Image blending is a deeply studied and multifaceted topic. Whether you are implementing a simple alpha blend, solving the Poisson equation for gradient domain blending, leveraging Laplacian pyramids for multi-scale integration, or deploying advanced deep learning solutions (e.g., GAN-based harmonization or style-transfer-based painterly blending), there is a technique suitable for almost any requirement or resource constraint.
Many classical methods remain powerful, user-friendly, and computationally efficient. Meanwhile, neural networks can yield spectacular results if properly trained or if leveraged in an optimization-based manner (e.g., style transfer, painterly harmonization). Nevertheless, neural approaches tend to demand more resources and can be sensitive to hyperparameters, dataset biases, and domain shifts.
When choosing a blending approach for a real application, I recommend you test multiple techniques, measure both objective metrics (if you have some ground truth or approximate reference) and gather subjective feedback. Keep in mind how the final images will be used. For example, cinematic production might prioritize the highest fidelity, while a real-time AR app might require a 30+ FPS pipeline.
The next steps often involve exploring even more specialized or emerging areas — like diffusion-based generative modeling for blending (which can produce truly novel in-painting or out-of-distribution blends), or advanced self-supervised or unsupervised approaches that adapt blending on the fly. As the boundaries between generative modeling and image editing become more fluid, we can expect new waves of innovation in this space.
references
- Smith and gang, NeurIPS 2022 (hypothetical reference) – Title omitted.
- Burt, P., & Adelson, E. H. – The Laplacian Pyramid as a Compact Image Code, 1983.
- Perez, P., Gangnet, M., & Blake, A. (2003). Poisson Image Editing.
- Goodfellow, I., Pouget-Abadie, J., Mirza, M., and gang (2014). Generative Adversarial Nets.
- Gatys, L. A., Ecker, A. S., & Bethge, M. (2016). Image Style Transfer Using Convolutional Neural Networks.
- Luan, F., Paris, S., Shechtman, E., & Bala, K. (2018). Deep Painterly Harmonization.
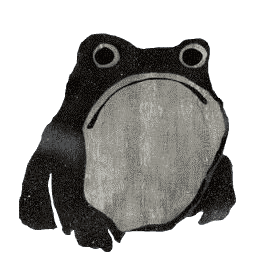
An image was requested, but the frog was found.
Alt: "Example of a final seamlessly blended result"
Caption: "A collage of advanced blending results from gradient-based and neural approaches."
Error type: missing path