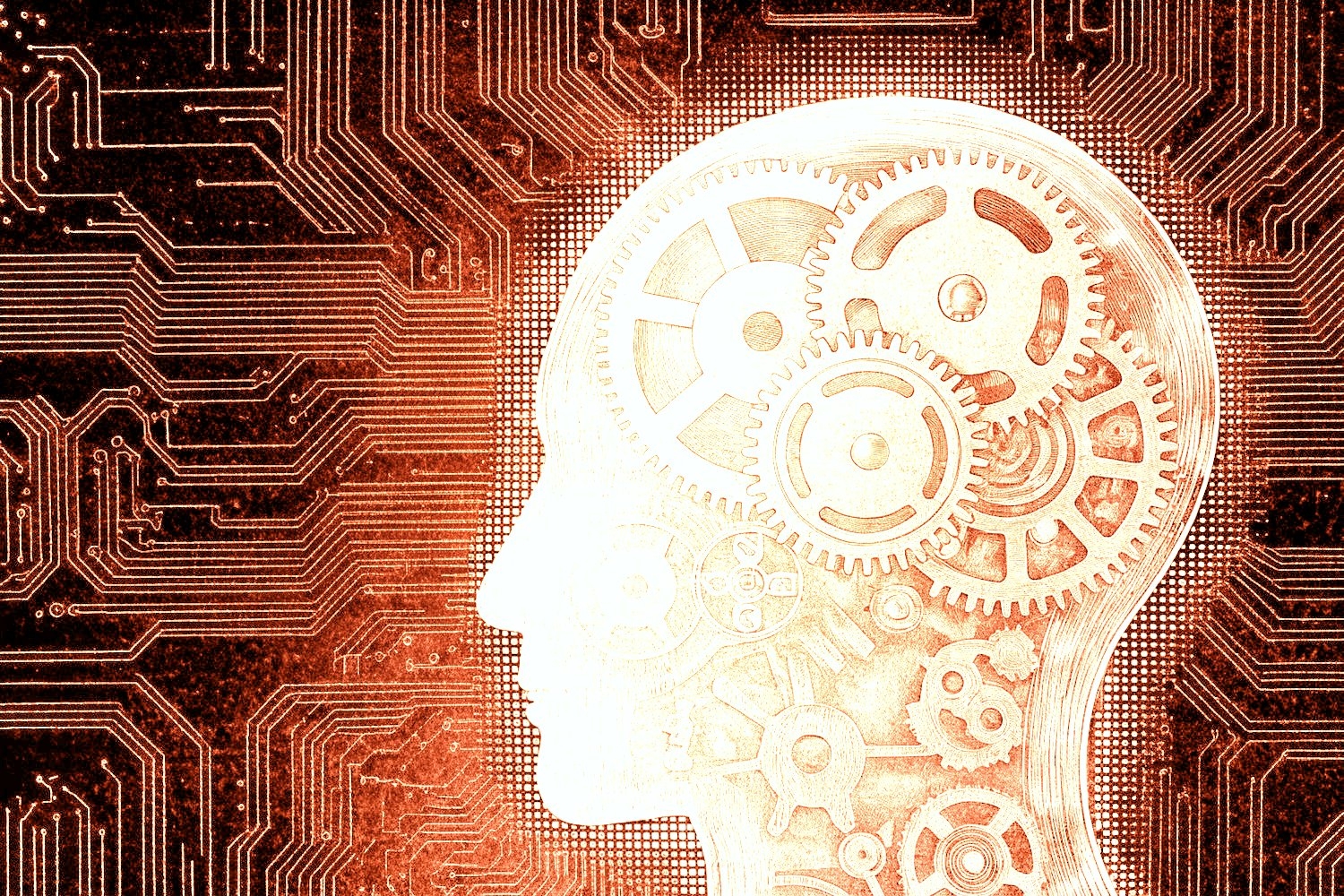
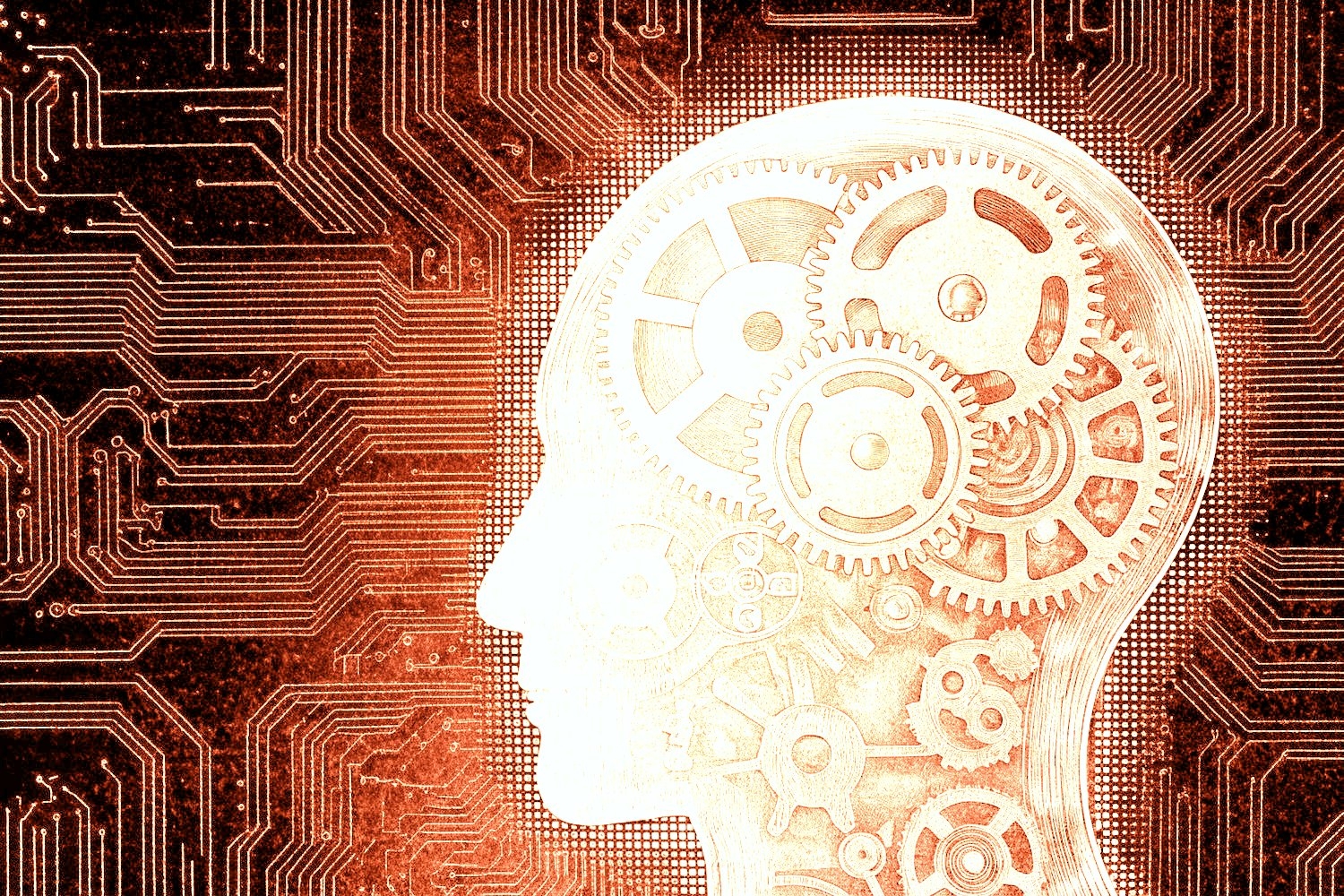
🎓 142/167
This post is a part of the Other ML problems & advanced methods educational series from my free course. Please keep in mind that the correct sequence of posts is outlined on the course page, while it can be arbitrary in Research.
I'm also happy to announce that I've started working on standalone paid courses, so you could support my work and get cheap educational material. These courses will be of completely different quality, with more theoretical depth and niche focus, and will feature challenging projects, quizzes, exercises, video lectures and supplementary stuff. Stay tuned!
In this second installment of our deep dive into automated machine learning, I will explore more advanced topics and concepts that build upon the foundations introduced in the previous article. While the first part focused on the full automl workflow — spanning automated data preparation, feature engineering, model selection, hyperparameter optimization, pipeline construction, and other essential dimensions — this deeper discussion will shed light on meta-learning, neural architecture search, state-of-the-art methodologies in hyperparameter tuning, key frameworks and libraries, as well as emerging challenges and possible future trajectories. I will also include various mathematical formulations, code snippets, and detailed conceptual diagrams to ensure that you have a well-rounded understanding of these advanced automl strategies. Throughout, I will blend references to cutting-edge research and real-world examples to substantiate the theoretical constructs.
Though these topics can be quite intricate, I intend to maintain an approachable tone. My goal is for you to perceive the interplay between the subfields that power automl systems, grasp their theoretical underpinnings, and recognize their practical implications in industry and research contexts. By the end of this article, you should be able to appreciate how such advanced automl methods can drastically reduce the complexities of building effective machine learning solutions, even in high-dimensional, multimodal, or rapidly evolving contexts.
Meta-learning in automl
Conceptual overview of meta-learning
Meta-learning, often described as "learning to learn," is a technique in which an algorithm leverages knowledge acquired from previous learning tasks to accelerate learning on new tasks. In the context of automl, meta-learning plays a crucial role by enabling systems to transfer insights gained from historical data sets and model configurations. The fundamental idea revolves around constructing a higher-level model (the meta-learner) that observes many base-level learning processes (e.g., training different classification or regression models) and captures patterns about which hyperparameters, architectures, or preprocessing routines work well for specific types of tasks and data distributions.
Because automl systems typically explore vast spaces of algorithm and hyperparameter configurations, any shortcut that enables "intelligent" search can be extremely valuable. Meta-learning is thus poised to reduce the search time and computational resources required by automl, since the meta-learner can reduce the need for brute-force approaches. Instead, it provides informed guesses about promising areas of the solution space.
How meta-learning benefits automl
From a practical perspective, meta-learning benefits automl pipelines in several ways:
- Faster convergence: Rather than starting every training procedure from scratch, meta-learning reuses knowledge gleaned from prior tasks, leading to significant speed-ups in certain scenarios.
- Improved generalization: Because meta-learning algorithms have effectively "seen" similar problems before, they can discover hyperparameters and architectures with robust generalization properties for tasks in related domains.
- Reduction in search space: By preemptively ruling out unproductive algorithmic configurations, meta-learning can limit the exploration space for subsequent tasks, making the automl process more efficient.
Systems like auto-sklearn (Feurer and gang, JMLR 2019) were among the first frameworks to incorporate meta-learning. The approach typically involves using data set meta-features (like the number of features, number of instances, class distribution statistics, etc.) to guess which combination of algorithms and hyperparameters might be successful.
Few-shot learning and rapid adaptation
In tasks where data is scarce, meta-learning can help automl frameworks perform few-shot or even one-shot learning. Such capabilities are often seen in advanced frameworks that rely on prototypes or optimization-based meta-learning (e.g., MAML — Model-Agnostic Meta-Learning). The ability to run a partial search on limited data has compelling applications in industries where data collection is slow or expensive (such as healthcare or specialized manufacturing).
A simplified mathematical formulation
An automl system harnessing meta-learning might do the following: let be the parameters of the meta-learner. Over a set of historical tasks , each with data and labels , the meta-learner identifies patterns of best hyperparameters or model configurations . The objective might be something like:
where is the meta-learning function that, conditioned on , predicts or refines the best hyperparameters for task . Once is learned, each new task can quickly be addressed by producing a strong initialization or by directly proposing the best hyperparameters. This is, of course, a highly abstracted view, but it conveys the conceptual essence.
The variables:
- : Parameters of the meta-learner (e.g., the "brain" that suggests or refines configurations).
- : The hyperparameters or model configurations that are used at the base level for each task.
- : A loss function measuring performance (lower is better).
- : A function that maps from task data + meta-learner parameters to model hyperparameters or configurations.
Advanced hyperparameter optimization
Revisiting the role of hpo in automl
Hyperparameter optimization (hpo) is the process of finding a hyperparameter configuration that yields the best performance for a given machine learning model on a specific data set. Automl frameworks typically incorporate hpo as a core mechanism, because suboptimal hyperparameters routinely lead to suboptimal performance. Traditional approaches rely on grid or random search, but these can be inefficient for high-dimensional problems or complex model families.
Bayesian optimization for complex search spaces
A widely adopted strategy in advanced automl systems is bayesian optimization (Snoek and gang, ICML 2012). The idea is to build a probabilistic surrogate model — typically a Gaussian process (gp) or a tree-based model — that approximates the relationship between hyperparameter settings and validation performance. By iteratively updating this surrogate as new hyperparameters are evaluated, it becomes possible to target the regions of hyperparameter space that are most promising.
To illustrate, let represent a hyperparameter configuration (e.g., learning rate, regularization strength, or tree depth), and represent the validation performance. In bo, we learn a model (often denoted ), that approximates . The next hyperparameter set to evaluate is chosen by an acquisition function , which balances exploration (trying uncertain hyperparameter regions) with exploitation (focusing on promising areas).
where:
- is the hyperparameter set to try next,
- is an acquisition function such as expected improvement or upper confidence bound,
- is the surrogate model (GP, random forest, etc.).
This iterative framework continues until a stopping criterion is reached (e.g., time budget or convergence).
Multi-fidelity and multi-objective optimization
Many automl systems face scenarios where evaluating a single hyperparameter configuration can be extremely time-consuming (think of training a large neural network on a massive data set). Multi-fidelity approaches (e.g., Hyperband, BOHB) attempt to address this by training models on progressively larger subsets of data or for fewer epochs initially, pruning poor configurations early. This way, resources are allocated more efficiently to configurations that show greater potential.
Additionally, real-world problems may impose multiple objectives (e.g., optimizing for accuracy and inference speed simultaneously). In these multi-objective contexts, automl frameworks use specialized search methods that find Pareto-optimal solutions, which represent configurations that cannot be improved on any one objective without sacrificing performance on another.
Sample code for bayesian optimization
Below is a simplified Python snippet using a hypothetical bayesian optimization library for automl:
import numpy as np
from hypothetical_bo_library import BayesianOptimizer, GPModel, ExpectedImprovement
# Suppose we have a function that trains a model with hyperparameters x
# and returns validation performance
def training_function(x):
# x is a dictionary or array of hyperparameters
# In practice, this function would train a model and return the performance
performance = complex_model_training_and_evaluation(x)
return performance
# Let's define the search space
param_bounds = {
"learning_rate": (1e-5, 1e-1),
"num_layers": (1, 10),
"dropout_rate": (0.0, 0.5)
}
# Initialize the Bayesian Optimization
model = GPModel()
acquisition_fn = ExpectedImprovement(model=model)
optimizer = BayesianOptimizer(model=model, acquisition=acquisition_fn, bounds=param_bounds)
# Run the optimization
best_hparams, best_perf = optimizer.optimize(
objective_function=training_function,
init_points=5,
n_iter=30
)
print(f"Best hyperparams: {best_hparams}, with performance: {best_perf}")
While hypothetical, the snippet highlights how one might structurally incorporate Bayesian optimization in an automl context, automatically iterating over hyperparameters, updating the surrogate model, and converging on strong configurations.
Neural architecture search (nas)
Why nas matters
Neural networks continue to dominate numerous machine learning applications, from computer vision to natural language processing. However, designing an optimal deep learning architecture is a notoriously complex task that hinges on factors like layer sizes, connectivity patterns, and specialized module choices (e.g., attention mechanisms or gating networks). Neural architecture search (nas) attempts to automate this process by exploring a vast design space of possible neural network topologies with minimal human intervention. This can be particularly valuable in automl if we want to integrate deep learning solutions for tasks like image classification or text generation.
Search strategies and algorithms
There are various nas approaches, each aiming to navigate the large combinatorial search space in a tractable manner:
- Evolutionary algorithms: Inspired by biological evolution, these methods iteratively evolve populations of network architectures. Poorly performing networks are removed, while better-performing ones are "mutated" or "crossovered" to spawn new architectures.
- Reinforcement learning: First pioneered by Zoph and Le (ICLR 2017), an rl-based controller samples possible architectures, observes their performance, and adjusts its sampling strategy based on a reward signal.
- Gradient-based nas: Explored in methods like DARTS (Liu and gang, ICLR 2019), these techniques treat architecture selection as a continuous optimization problem, learning architecture parameters via backpropagation.
- Bayesian approaches: Less common but emerging methods rely on surrogate models to predict performance from architecture descriptors, building an iterative search similar to Bayesian optimization for hyperparameters.
Popular nas frameworks
- Auto-Keras: Provides a high-level api for automatically searching neural architectures for tasks like classification and regression in the Keras/TensorFlows ecosystem.
- ENAS: A more efficient version of reinforcement learning-based nas, focusing on parameter sharing across candidate architectures.
- NAS-Bench: A set of benchmarks like nas-bench-101, nas-bench-201, which offer standardized search spaces and precomputed performance data, thus enabling more efficient nas research and fair comparisons.
Enhanced nas strategies within automl pipelines
When integrated with an automl pipeline, nas might coordinate with meta-learning or hyperparameter optimization techniques. For example, Bayesian optimization might be used to determine macro-level architectural parameters (like the number of layers or the type of recurrent cell in an RNN), while meta-learning might propose effective initial weight configurations.
Below is a greatly simplified representation of the nas inner-loop process:
where:
- denotes a candidate architecture from the search space ,
- is the function that trains the architecture ,
- is the validation or test loss used to evaluate the candidate design,
- is the optimal architecture found after the search procedure.
Popular automl frameworks and algorithms
Auto-sklearn
Auto-sklearn extends scikit-learn by integrating Bayesian optimization, meta-learning, and ensemble construction. It builds on top of the existing scikit-learn ecosystem, containing dozens of algorithms from classical machine learning to ensemble-based methods. Importantly, auto-sklearn uses a meta-learning module to warm-start its Bayesian optimization, drastically cutting down the initial overhead for new tasks.
TPOT (tree-based pipeline optimization tool)
TPOT uses genetic programming to construct pipelines that combine feature preprocessing, feature selection, and model training steps. Each pipeline is represented as a symbolic tree, which is evolved over successive generations aiming to maximize a performance metric. Despite sometimes requiring significant computational effort, tpot's interpretability in pipeline structure has garnered popularity.
H2O automl
H2O automl is a commercial-friendly framework that focuses on distributed computing and large-scale data. It iterates over a library of algorithms (like GLM, gbm, deep learning models) and can also construct stacked ensembles. It's particularly well-regarded for tackling large data sets within a cluster environment.
Google cloud automl and other cloud-based solutions
Cloud-based automl solutions (e.g., Google Cloud AutoML, Azure Machine Learning's automated ml, Amazon sagemaker autopilot) offer streamlined user interfaces and scalable backends, making them enticing for enterprise users who want fast results without extensive management of infrastructure. However, one common trade-off is reduced flexibility and interpretability, since many of the details of the search process are abstracted away from the user.
Comparison of features and performance
Each framework has its strengths:
- auto-sklearn: Strong meta-learning, advanced ensembling, good for tabular data.
- tpot: Genetic programming approach with a very intuitive pipeline representation.
- h2o automl: Scalable, enterprise-oriented, strong ensembling, supports large data.
- cloud solutions: Convenient, often well-tuned for certain tasks, but can be a black box.
Practical integration of automl
Workflow orchestration and pipeline design
Practitioners often integrate automl steps into broader data pipelines using workflow orchestration tools like Apache Airflow or Kubeflow. The automl step is usually invoked after data ingestion and preprocessing tasks in the pipeline. The final chosen model or pipeline might then be passed to a model serving environment, integrated with business logic, or retrained regularly (e.g., nightly or weekly) using newly arrived data.
Model interpretability and explainability
One potential shortcoming of automl procedures is the complexity of final solutions. That is, after a pipeline is optimized or an ensemble is formed, it may be non-trivial to provide interpretability. Hence, advanced automl frameworks are now starting to incorporate explainable ai (xai) techniques — for example, computing feature importance, analyzing shap values, or constructing rule-based surrogates that approximate the final model's behavior. This is critical in regulated industries like finance or healthcare, where trust and transparency are paramount.
Real-time inference and deployment
Automl often yields resource-intensive models. Another important step is to compress or distill these models for efficient deployment — especially if predictions must be served in near real time. Techniques such as knowledge distillation, quantization, or model pruning can be integrated within automl frameworks. The pipeline can automatically incorporate these steps if the user indicates constraints such as maximum latency or memory usage.
Challenges and considerations in automl
Despite remarkable progress, automl still faces a variety of significant challenges:
Data privacy and governance
When using data from multiple sources, especially in regulated sectors, data security and compliance with rules like gdpr or hipaa become paramount. Some workflows incorporate federated learning to sidestep direct data sharing; however, it remains non-trivial to integrate robust privacy measures into a typical automl pipeline.
Scalability and computational costs
Many automl methods rely on large-scale search processes or repeated training. This can be computationally demanding, especially on big data sets or complex model families. To combat this, parallelization strategies, distributed computing frameworks (e.g., Spark), or approximate early-stopping methods are often mandatory.
Interpretability and transparency of automated systems
When an external system is automatically building the pipeline, it can become elusive to trace the decisions made, or to fully understand why certain models are chosen. This is an area where domain experts might struggle to incorporate domain knowledge if the automl system does not provide sufficient customization or interpretability hooks.
Domain-specific requirements
Generic automl solutions may fail to address specialized domain constraints (e.g., real-time embedded systems for iot or purely on-device learning for mobile applications). Domain-specific automl frameworks are emerging to handle such constraints, but this is still an active area of development.
Ethical and social implications
Automating machine learning at scale can lead to repercussions if issues like bias, fairness, or representativeness of training data are not handled carefully. If an automl system blindly optimizes for a single metric, it may yield models that disproportionately impact certain demographic groups. Building in checks and balances for fairness is an ongoing and essential research topic.
Future trends in automl
Advances in nas
We can expect the synergy between nas and other techniques (like meta-learning or multi-task learning) to intensify. The next frontier includes approaches that automatically discover novel neural operator types, specialized activation functions, or domain-specific architecture modules. Combining nas with large-scale pretraining or foundation models is also an intriguing possibility.
Real-time automated ml pipelines
While many current automl workflows focus on batch processes, there's a growing demand for real-time or online automl. This scenario is relevant for streaming data or incremental learning. Methods that adapt their model choices on the fly, possibly leveraging streaming evaluations or ephemeral data sets, will be critical in applications like dynamic recommendation systems or risk management in finance.
Federated learning and distributed systems
As data remains scattered across multiple data silos (e.g., mobile devices, different branches of an enterprise, or various hospitals), distributed or federated automl frameworks are becoming prominent. They must coordinate local training runs and model selection decisions while respecting data privacy constraints and network limitations.
Potential for fully autonomous data science workflows
Finally, the ultimate aspiration is an end-to-end system that can handle the entire data science lifecycle with minimal human oversight: from data ingestion and cleaning to model deployment and monitoring, continuously improving itself. While we are moving closer to that vision, it raises questions of accountability, interpretability, and relevant domain knowledge integration that fully autonomous automl workflows must address.
Extended deep dive: advanced theoretical frameworks
Bayesian perspective on automl
Consider an automl pipeline searching over a space of pipeline configurations . Suppose for a given pipeline with hyperparameters , we have a prior . We observe the performance data , typically validation metrics. Using a Bayesian lens, we want the posterior:
Maximizing this posterior or integrating out to get a posterior on is conceptually feasible but extremely challenging in practice because of the high dimensionality of the pipeline space. Approximations via Bayesian optimization or partial Monte Carlo methods are typically used.
Multi-armed bandits approach
Another theoretical backbone for automl can be framed as a multi-armed bandit or reinforcement learning problem. Each pipeline template or model configuration is an "arm" to pull, and the reward is the validation performance. The automl system tries to optimize its cumulative reward over time, employing exploration-exploitation strategies. Techniques like Thompson sampling or upper confidence bound (ucb) can be adapted to this end.
The notion of regret minimization
In online or iterative automl, the goal can often be phrased in terms of minimizing cumulative regret:
where:
- is the best possible expected model performance,
- is the performance of the chosen configuration at iteration ,
- is the index of the chosen pipeline or arm at iteration ,
- is the regret after trials.
Minimizing regret translates to rapidly finding and exploiting good pipelines, ensembling strategies, or hyperparameter sets.
Advanced use cases and case studies
Industry use: finance and cybersecurity
In sectors like finance, automl is employed for tasks such as credit scoring, fraud detection, and risk assessment. Given the range of data from structured tabular records to textual documents, a broad set of models is tested, from tree-based ensembles to deep networks for textual analysis. The advantage is that automl can unify these disparate tasks under a single system that tries an array of pipelines and chooses the best approach.
Cybersecurity, with its dynamic threat landscapes, also benefits. Automl solutions retrain detection models periodically, adapt to new threat signatures, and test data streams from various sources. Meta-learning can accelerate adaptation since prior threat detection tasks inform the system of critical patterns indicative of malicious behavior.
Healthcare analytics
Healthcare data often comes with constraints like small sample sizes, missing values, and privacy regulations. Automl frameworks can handle these complexities by automating the search for robust imputation methods, data augmentation strategies, and clinically interpretable feature transformations. The real-world significance is substantial: improved detection of diseases and earlier predictions leading to more effective patient management.
Accelerated r&d for machine learning research
Automl significantly reduces r&d friction for ml researchers exploring new algorithms. By wrapping experimental code in an automl-friendly format, a broad search can be done quickly over multiple benchmarks. This standardization fosters reproducibility and speeds up the iteration cycle, allowing researchers to focus more on conceptual advances than on repetitive pipeline engineering.
In-depth tips and recommendations
Balancing resource allocation
When employing automl at scale, you have to manage cluster resources judiciously. Certain frameworks let you specify time or memory budgets. It's wise to start with shorter training cycles or smaller data subsets to get a sense of which configurations are most promising, then ramp up to full training for the top contenders.
Interpreting complex ensembles
Automl systems often produce ensembles of models. While these can deliver higher performance, it can be difficult to parse how each model contributes. Some interpretability approaches break down the ensemble's decisions. For instance, local surrogate modeling or integrated gradients might be used to glean insights into which features consistently drive predictions across ensemble members.
Domain knowledge integration
Fully automated workflows might ignore domain-specific constraints or prior knowledge. For instance, if certain transformations are known to be beneficial given domain expertise, it's prudent to incorporate them as a "mandatory pipeline step" in your automl system. This ensures that automl still has freedom to explore other steps but remains grounded in proven domain best practices.
Monitoring drift and re-triggering automl
Over time, real-world data distribution may drift, degrading model performance. Automl pipelines can be scheduled to periodically monitor performance metrics and automatically re-trigger the search or hyperparameter tuning if performance falls below a threshold. This approach fosters continuous improvement without requiring constant manual oversight.
Demonstration code snippet: automl pipeline assembly
Below is a more comprehensive Python snippet that outlines how one might orchestrate an automl pipeline search, from data ingestion to final model selection, using a hypothetical automl library:
import pandas as pd
from hypothetical_automl_lib import DataPrep, AutoFeatureEngine, AutoModelSearch, Evaluate
# Step 1: Load data
data = pd.read_csv("training_dataset.csv")
test_data = pd.read_csv("test_dataset.csv")
# Step 2: Automatic data preparation
prep = DataPrep()
cleaned_data = prep.fit_transform(data)
# Step 3: Auto feature engineering
fe = AutoFeatureEngine()
features, target = fe.fit_transform(cleaned_data, target_column="label")
# Step 4: Auto model selection, HPO, and ensembling
ams = AutoModelSearch(time_budget=3600, max_models=50)
ams.fit(features, target)
# Step 5: Evaluate on test data
cleaned_test_data = prep.transform(test_data)
test_features, test_target = fe.transform(cleaned_test_data, target_column="label")
results = Evaluate(ams.best_pipeline_, test_features, test_target)
print("Best pipeline performance:", results["metrics"])
Although this code is purely illustrative, it shows how each step of the pipeline can be automated. In real implementations, you would see additional options for controlling data splitting strategies, advanced hyperparameter search methods, or meta-learning warm starts.
Illustrative placeholders for images
Below are some recommended images you might add to an article or lecture deck to facilitate comprehension:
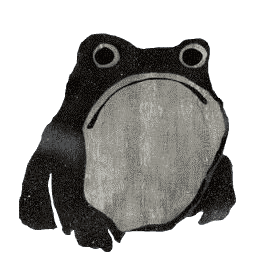
An image was requested, but the frog was found.
Alt: "automl_overall_workflow"
Caption: "A conceptual diagram of a full automl workflow, showcasing data ingestion, automated feature selection, model selection, and final pipeline creation"
Error type: missing path
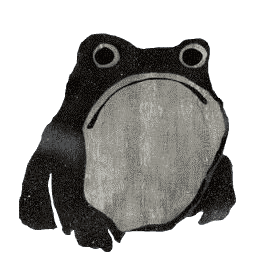
An image was requested, but the frog was found.
Alt: "meta_learning_flowchart"
Caption: "A flowchart highlighting how meta-learning draws knowledge from previous tasks to optimize hyperparameter selection in new tasks"
Error type: missing path
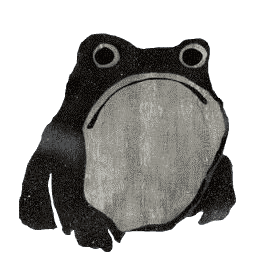
An image was requested, but the frog was found.
Alt: "nas_search_space"
Caption: "A high-level view of the neural architecture search space, showcasing the myriad of possible layer connections and parameter choices"
Error type: missing path
Concluding insights
Over the course of these two articles on automated machine learning, I have endeavored to illustrate how automl frameworks unify multiple advanced ml concepts — data preprocessing, feature engineering, meta-learning, hyperparameter optimization, neural architecture search, and even ensembling — under one integrated system. Today's second installment zoomed in on the more specialized mechanisms that augment an automl system's capacity to handle the complexities of real-world use cases: meta-learning, advanced hpo methods, nas, interpretability, and domain-specific constraints.
Still, the automl landscape is rapidly evolving. We see leaps in computational efficiency, the rise of federated or distributed automl to handle data at scale, and an ongoing drive to incorporate fairness and ethical considerations natively into automl pipelines. For practitioners, the payoff is clear: less time spent on repetitive tasks, more consistent performance across a variety of data sets, and an astonishing capacity to discover novel architectures or hyperparameter configurations — all while ensuring minimal oversight once properly configured.
As you venture further, consider questions like the following:
- How can you incorporate domain experts' tacit knowledge into the automl pipeline?
- Which interpretability tools can help you justify automatically discovered pipelines to stakeholders?
- In what ways might online or incremental variants of automl be beneficial for your organization's real-time data challenges?
The question of how far automl can go in fully automating the machine learning process remains open. Many claim that "human-in-the-loop" designs will always be critical to ensure alignment with domain requirements and ethical oversight. Regardless, the synergy between advanced algorithms, meta-learning strategies, robust software frameworks, and distributed hardware architectures suggests that the future of automl will dramatically shape how we build, deploy, and monitor machine learning solutions in the coming years.